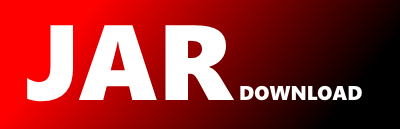
net.calledtoconstruct.Right Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of flow Show documentation
Show all versions of flow Show documentation
A small collection of potentially useful classes for streamlining process flow.
The newest version!
package net.calledtoconstruct;
import java.util.Arrays;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiFunction;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Supplier;
import java.util.stream.Collectors;
public class Right implements Either {
private final TRight value;
/**
* An implementation of {@link Either} in which the {@link Right} value of type {@code TRight} is reatined.
*
* @param value The value of type {@code TRight} to store.
*/
public Right(TRight value) {
this.value = value;
}
@Override
public Either onLeftApply(Function function) {
return new Right<>(value);
}
@Override
public Either onRightApply(Function function) {
final TOther other = function.apply(value);
return new Right<>(other);
}
@Override
public Either onLeftAccept(Consumer consumer) {
return this;
}
@Override
public Either onRightAccept(Consumer consumer) {
consumer.accept(value);
return this;
}
@Override
public Either onLeftSupply(Supplier supplier) {
return new Right<>(value);
}
@Override
public Either onRightSupply(Supplier supplier) {
final var other = supplier.get();
return new Right<>(other);
}
/**
* Get the contained value of type {@code TRight}.
*
* @return the value of type {@code TRight}
*/
public TRight getValue() {
return value;
}
/**
* A function which accepts zero to many instances of {@link Either}, collects all the values from those of type {@link Right}, and invokes the supplied
* {@code consumer}, passing the {@link java.util.List} of {@code TRight} values as the parameter.
*
* @param The type for the {@link Left} value.
* @param The type for the {@link Right} value.
* @param consumer A method which receives a {@link java.util.List} of {@code TRight} values collected from any of the supplied {@code input} which are of type {@link Right}.
* @param input Zero or more instances of {@link Either} to be evaluated.
* @return {@code true} when {@code input} contains at least one instance of {@link Right}, otherwise, false.
*/
@SafeVarargs
public static boolean acceptAll(Consumer> consumer, Either... input) {
final var values = Arrays.stream(input)
.map(either -> (either instanceof Right right) ? right.getValue() : null)
.filter(Objects::nonNull)
.collect(Collectors.toList());
if (values.size() == input.length) {
consumer.accept(values);
return true;
}
return false;
}
/**
* A function which accepts zero to many instances of {@link Either}, searches for any instance of type {@link Right}, then extracts its value and returns
* it as an {@link java.util.Optional}. When no instance of type {@link Right} is provided, an empty {@link java.util.Optional} is returned.
*
* @param The type for the {@link Left} value.
* @param The type for the {@link Right} value.
* @param input Zero or more instances of {@link Either} to be evaluated.
* @return And {@link java.util.Optional} of {@code TRight} or empty.
*/
@SafeVarargs
public static Optional any(Either... input) {
for (var either : input) {
if (either instanceof Right right) {
return Optional.of(right.getValue());
}
}
return Optional.empty();
}
@Override
public Either mergeFailToLeft(
Either other,
BiFunction functionMergeLeft,
BiFunction functionMergeRight,
Function transformThis,
Function transformOther
) {
if (other instanceof Right right) {
return new Right<>(functionMergeRight.apply(value, right.value));
} else if (other instanceof Left left) {
return new Left<>(transformOther.apply(left.getValue()));
} else {
throw new UnexpectedNeitherException();
}
}
@Override
public Either mergeFailToRight(
Either other,
BiFunction functionMergeLeft,
BiFunction functionMergeRight,
Function transformThis,
Function transformOther
) {
if (other instanceof Right right) {
return new Right<>(functionMergeRight.apply(value, right.value));
} else if (other instanceof Left) {
return new Right<>(transformThis.apply(value));
} else {
throw new UnexpectedNeitherException();
}
}
@Override
public Either flip() {
return new Left<>(value);
}
@Override
public Either onLeftFlatMap(
Function> function,
Function otherwise
) {
return new Right<>(value);
}
@Override
public Either onRightFlatMap(
Function> function,
Function otherwise
) {
return function.apply(value)
.map(out -> new Right(out))
.orElse(new Right<>(otherwise.apply(value)));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy