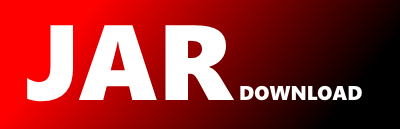
net.calledtoconstruct.Tuple Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of flow Show documentation
Show all versions of flow Show documentation
A small collection of potentially useful classes for streamlining process flow.
The newest version!
package net.calledtoconstruct;
import java.lang.reflect.Array;
import java.util.Optional;
public interface Tuple {
/**
* A function that returns a new {@link java.util.Optional} of {@link Tuple} containing
* the values of this instance minus the last one, or an empty {@link java.util.Optional}
* of {@link Tuple} if only a single value was present.
*
* @return When the source {@link Tuple} contains two more more values, {@link java.util.Optional} of {@link Tuple}, else
* and empty {@link java.util.Optional} of {@link Tuple}
*/
Optional tryPop();
/**
* A function that returns a new {@link java.util.Optional} of {@link Tuple} containing
* the values of this instance plus the supplied {@code value}. However, if adding the
* value exceeds the available {@link Tuple} classes, then an empty {@link java.util.Optional}
* is returned.
*
* @param The type of the {@code value} being added.
* @param value The value being added.
* @return A new {@link java.util.Optional} of {@link Tuple}, or an empty {@link java.util.Optional}
*/
Optional tryPush(T value);
/**
* A function that returns a new {@link java.util.Optional} of {@link Tuple} containing
* the values of this instance minus the first one, or an empty {@link java.util.Optional}
* of {@link Tuple} if only a single value was present.
*
* @return When the source {@link Tuple} contains two more more values, {@link java.util.Optional} of {@link Tuple}, else
* and empty {@link java.util.Optional} of {@link Tuple}
*/
Optional tryShift();
/**
* A function that returns a new {@link java.util.Optional} of {@link Tuple} containing
* the values of this instance plus the supplied {@code value} prepended as the first value. However,
* if adding the value exceeds the available {@link Tuple} classes, then an empty {@link java.util.Optional}
* is returned.
*
* @param The type of the {@code value} being added.
* @param value The value being added.
* @return A new {@link java.util.Optional} of {@link Tuple}, or an empty {@link java.util.Optional}
*/
Optional tryUnshift(T value);
static T[] toArray(final Tuple1 tuple, Class clazz) {
final var array = Array.newInstance(clazz, 1);
Array.set(array, 0, tuple.getFirst());
return (T[]) array;
}
static T[] toArray(final Tuple2 tuple, Class clazz) {
final var array = Array.newInstance(clazz, 2);
Array.set(array, 0, tuple.getFirst());
Array.set(array, 1, tuple.getSecond());
return (T[]) array;
}
static T[] toArray(final Tuple3 tuple, Class clazz) {
final var array = Array.newInstance(clazz, 3);
Array.set(array, 0, tuple.getFirst());
Array.set(array, 1, tuple.getSecond());
Array.set(array, 2, tuple.getThird());
return (T[]) array;
}
static T[] toArray(final Tuple4 tuple, Class clazz) {
final var array = Array.newInstance(clazz, 4);
Array.set(array, 0, tuple.getFirst());
Array.set(array, 1, tuple.getSecond());
Array.set(array, 2, tuple.getThird());
Array.set(array, 3, tuple.getFourth());
return (T[]) array;
}
static T[] toArray(final Tuple5 tuple, Class clazz) {
final var array = Array.newInstance(clazz, 5);
Array.set(array, 0, tuple.getFirst());
Array.set(array, 1, tuple.getSecond());
Array.set(array, 2, tuple.getThird());
Array.set(array, 3, tuple.getFourth());
Array.set(array, 4, tuple.getFifth());
return (T[]) array;
}
static T[] toArray(final Tuple6 tuple, Class clazz) {
final var array = Array.newInstance(clazz, 6);
Array.set(array, 0, tuple.getFirst());
Array.set(array, 1, tuple.getSecond());
Array.set(array, 2, tuple.getThird());
Array.set(array, 3, tuple.getFourth());
Array.set(array, 4, tuple.getFifth());
Array.set(array, 5, tuple.getSixth());
return (T[]) array;
}
static T[] toArray(final Tuple7 tuple, Class clazz) {
final var array = Array.newInstance(clazz, 7);
Array.set(array, 0, tuple.getFirst());
Array.set(array, 1, tuple.getSecond());
Array.set(array, 2, tuple.getThird());
Array.set(array, 3, tuple.getFourth());
Array.set(array, 4, tuple.getFifth());
Array.set(array, 5, tuple.getSixth());
Array.set(array, 6, tuple.getSeventh());
return (T[]) array;
}
static T[] toArray(final Tuple8 tuple, Class clazz) {
final var array = Array.newInstance(clazz, 8);
Array.set(array, 0, tuple.getFirst());
Array.set(array, 1, tuple.getSecond());
Array.set(array, 2, tuple.getThird());
Array.set(array, 3, tuple.getFourth());
Array.set(array, 4, tuple.getFifth());
Array.set(array, 5, tuple.getSixth());
Array.set(array, 6, tuple.getSeventh());
Array.set(array, 7, tuple.getEighth());
return (T[]) array;
}
Optional tryGetFirst(Class clazz);
Optional tryGetLast(Class clazz);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy