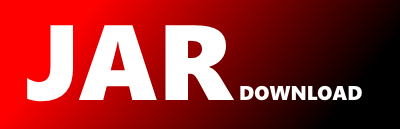
net.calledtoconstruct.Tuple2 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of flow Show documentation
Show all versions of flow Show documentation
A small collection of potentially useful classes for streamlining process flow.
The newest version!
package net.calledtoconstruct;
import java.util.Optional;
public class Tuple2 implements Tuple {
private final T1 firstValue;
private final T2 secondValue;
public Tuple2(T1 firstValue, T2 secondValue) {
this.firstValue = firstValue;
this.secondValue = secondValue;
}
/**
* A function that returns a new {@link Tuple3} containing
* the values of this instance plus the supplied {@code value}.
*
* @param The type of {@code value} being added.
* @param value A value to be added.
* @return An instance of {@link Tuple3}
*/
public Tuple3 push(T value) {
return new Tuple3<>(firstValue, secondValue, value);
}
/**
* A function that returns a new instance of {@link Tuple1} containing the
* first value from this instance.
*
* @return A {@link Tuple1} containing the first value from this instance.
*/
public Tuple1 pop() {
return new Tuple1<>(firstValue);
}
/**
* A function that returns a new instance of {@link Tuple1} containing the
* last value from this instance.
*
* @return A {@link Tuple1} containing the last value from this instance.
*/
public Tuple1 shift() {
return new Tuple1<>(secondValue);
}
/**
* A function that returns a new {@link Tuple3} containing
* the supplied {@code value} plus the value of this instance.
*
* @param The type of {@code value} being added.
* @param value A value to be added.
* @return An instance of {@link Tuple3}
*/
public Tuple3 unshift(T value) {
return new Tuple3<>(value, firstValue, secondValue);
}
@Override
public Optional tryPop() {
return Optional.of(pop());
}
@Override
public Optional tryPush(T value) {
return Optional.of(push(value));
}
@Override
public Optional tryShift() {
return Optional.of(shift());
}
@Override
public Optional tryUnshift(T value) {
return Optional.of(unshift(value));
}
/**
* A function that returns the first value from this instance.
*
* @return A value of type {@code T1}
*/
public T1 getFirst() {
return firstValue;
}
/**
* A function that returns the second value from this instance.
*
* @return A value of type {@code T2}
*/
public T2 getSecond() {
return secondValue;
}
public Tuple3 append(final Tuple1 other) {
return new Tuple3<>(firstValue, secondValue, other.getFirst());
}
public Tuple4 append(final Tuple2 other) {
return new Tuple4<>(firstValue, secondValue, other.getFirst(), other.getSecond());
}
public Tuple5 append(final Tuple3 other) {
return new Tuple5<>(firstValue, secondValue, other.getFirst(), other.getSecond(), other.getThird());
}
public Tuple6 append(final Tuple4 other) {
return new Tuple6<>(firstValue, secondValue, other.getFirst(), other.getSecond(), other.getThird(), other.getFourth());
}
public Tuple7 append(final Tuple5 other) {
return new Tuple7<>(firstValue, secondValue, other.getFirst(), other.getSecond(), other.getThird(), other.getFourth(), other.getFifth());
}
public Tuple8 append(final Tuple6 other) {
return new Tuple8<>(firstValue, secondValue, other.getFirst(), other.getSecond(), other.getThird(), other.getFourth(), other.getFifth(), other.getSixth());
}
public Tuple3 prepend(final Tuple1 other) {
return new Tuple3<>(other.getFirst(), firstValue, secondValue);
}
public Tuple4 prepend(final Tuple2 other) {
return new Tuple4<>(other.getFirst(), other.getSecond(), firstValue, secondValue);
}
public Tuple5 prepend(final Tuple3 other) {
return new Tuple5<>(other.getFirst(), other.getSecond(), other.getThird(), firstValue, secondValue);
}
public Tuple6 prepend(final Tuple4 other) {
return new Tuple6<>(other.getFirst(), other.getSecond(), other.getThird(), other.getFourth(), firstValue, secondValue);
}
public Tuple7 prepend(final Tuple5 other) {
return new Tuple7<>(other.getFirst(), other.getSecond(), other.getThird(), other.getFourth(), other.getFifth(), firstValue, secondValue);
}
public Tuple8 prepend(final Tuple6 other) {
return new Tuple8<>(other.getFirst(), other.getSecond(), other.getThird(), other.getFourth(), other.getFifth(), other.getSixth(), firstValue, secondValue);
}
@Override
public Optional tryGetFirst(Class clazz) {
if (clazz.isAssignableFrom(firstValue.getClass())) {
final var cast = clazz.cast(firstValue);
return Optional.of(cast);
}
return Optional.empty();
}
@Override
public Optional tryGetLast(Class clazz) {
if (clazz.isAssignableFrom(secondValue.getClass())) {
final var cast = clazz.cast(secondValue);
return Optional.of(cast);
}
return Optional.empty();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy