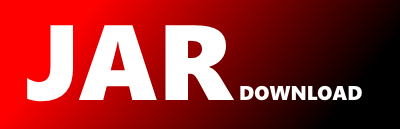
net.cassite.style.aggregation.IterableFuncSup Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of style Show documentation
Show all versions of style Show documentation
Java 8 functional programming toolbox supporting basic jdk libraries and providing language enhancing.
The newest version!
package net.cassite.style.aggregation;
import java.util.Collection;
import java.util.Iterator;
import net.cassite.style.StyleRuntimeException;
import net.cassite.style.def;
import net.cassite.style.ptr;
import net.cassite.style.control.*;
import net.cassite.style.interfaces.RFunc1;
import static net.cassite.style.Style.*;
import static net.cassite.style.aggregation.Aggregation.*;
/**
* Supporter of iterables
*
* @param element type of the iterable
* @author wkgcass
*/
public class IterableFuncSup implements A1FuncSup {
protected final Iterable iterable;
IterableFuncSup(Iterable iterable) {
this.iterable = iterable;
}
public R forThose(RFunc1 predicate, def func) {
Iterator it = iterable.iterator();
ptr i = ptr(0);
IteratorInfo info = new IteratorInfo<>();
return While(it::hasNext, (loopInfo) -> {
T t = it.next();
try {
return If(predicate.apply(t), () -> {
return func.apply(t, info.setValues(i.item - 1, i.item + 1, i.item == 0, it.hasNext(), loopInfo.currentIndex,
loopInfo.effectiveIndex, loopInfo.lastRes));
}).Else(() -> null);
} catch (Throwable err) {
StyleRuntimeException sErr = $(err);
Throwable throwable = sErr.origin();
if (throwable instanceof Remove) {
it.remove();
} else {
throw sErr;
}
} finally {
i.item += 1;
}
return null;
});
}
@Override
public T first() {
Iterator it = iterable.iterator();
if (it.hasNext()) {
return it.next();
} else {
return null;
}
}
public > Transformer to(Coll collection) {
return new Transformer<>(iterable, collection);
}
/**
* Transformer which transforms an iterable into a collection
*
* @param element type of collection to transform to
* @param element type of the iterable
* @param collection
* @author wkgcass
*/
public static class Transformer> implements A1Transformer {
protected final Coll collection;
protected final Iterable iterable;
Transformer(Iterable iterable, Coll collection) {
this.iterable = iterable;
this.collection = collection;
}
@Override
public Coll via(def method) {
$(iterable).forEach(e -> {
collection.add(method.apply(e));
});
return collection;
}
}
@Override
public > Coll findAll(def filter, Coll toColl, int limit) {
return $(iterable).to(toColl).via(e -> {
Boolean ret = filter.apply(e);
if (null == ret || ret.equals(false))
Continue();
if (limit > 0 && limit <= toColl.size()) {
Break();
}
return e;
});
}
/**
* Interface of classes to transform an iterable object into another
* array
*
* @param returned array type
* @param input iterable type
* @author wkgcass
*/
public static class ArrTransformer implements A1ArrTransformer {
protected final R[] retArr;
protected final Iterable iterable;
ArrTransformer(Iterable iterable, R[] retArr) {
this.iterable = iterable;
this.retArr = retArr;
}
@Override
public R[] via(def method) {
$(iterable).forEach((e, i) -> {
if (retArr.length == i.effectiveIndex) {
Break();
} else {
retArr[i.effectiveIndex] = method.apply(e);
}
});
return retArr;
}
}
@Override
public A1ArrTransformer to(R[] arr) {
return new ArrTransformer<>(iterable, arr);
}
@Override
public int size() {
return forEach((e, i) -> i.currentIndex);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy