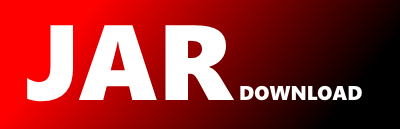
net.sourceforge.cilib.entity.topologies.AbstractTopology Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cilib-library Show documentation
Show all versions of cilib-library Show documentation
A library of composable components enabling simpler Computational Intelligence
/** __ __
* _____ _/ /_/ /_ Computational Intelligence Library (CIlib)
* / ___/ / / / __ \ (c) CIRG @ UP
* / /__/ / / / /_/ / http://cilib.net
* \___/_/_/_/_.___/
*/
package net.sourceforge.cilib.entity.topologies;
import com.google.common.collect.ForwardingList;
import com.google.common.collect.Lists;
import java.util.AbstractCollection;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import net.sourceforge.cilib.controlparameter.ConstantControlParameter;
import net.sourceforge.cilib.controlparameter.ControlParameter;
import net.sourceforge.cilib.entity.Entity;
import net.sourceforge.cilib.entity.Topology;
import net.sourceforge.cilib.entity.visitor.TopologyVisitor;
/**
* This an abstract class which extends from the abstract Topology class.
* All {@linkplain net.sourceforge.cilib.algorithm.population.PopulationBasedAlgorithm}
* Topologies must inherit from this class.
*
* @param The {@code Entity} type.
*/
public abstract class AbstractTopology extends ForwardingList implements Topology {
private static final long serialVersionUID = -9117512234439769226L;
protected List entities;
protected ControlParameter neighbourhoodSize;
/**
* Default constructor.
*/
public AbstractTopology() {
this.entities = Lists.newArrayList();
this.neighbourhoodSize = ConstantControlParameter.of(3.0);
}
/**
* Copy constructor.
*/
public AbstractTopology(AbstractTopology copy) {
this.neighbourhoodSize = copy.neighbourhoodSize.getClone();
this.entities = Lists.newArrayList();
for (E entity : copy.entities) {
this.entities.add((E) entity.getClone());
}
}
/**
* {@inheritDoc}
*/
@Override
public Iterator iterator() {
return entities.iterator();
}
/**
* {@inheritDoc}
*/
@Override
public void accept(TopologyVisitor visitor) {
visitor.visit(this);
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
final AbstractTopology other = (AbstractTopology) obj;
if (this.entities != other.entities && (this.entities == null || !this.entities.equals(other.entities))) {
return false;
}
return true;
}
@Override
public int hashCode() {
int hash = 7;
hash = 43 * hash + (this.entities != null ? this.entities.hashCode() : 0);
return hash;
}
@Override
protected List delegate() {
return this.entities;
}
public void setNeighbourhoodSize(ControlParameter neighbourhoodSize) {
this.neighbourhoodSize = neighbourhoodSize;
}
public int getNeighbourhoodSize() {
return Long.valueOf(Math.round(neighbourhoodSize.getParameter())).intValue();
}
protected abstract Iterator neighbourhoodOf(E e);
public final Collection neighbourhood(final E e) {
return new AbstractCollection() {
@Override
public Iterator iterator() {
return neighbourhoodOf(e);
}
@Override
public int size() {
return getNeighbourhoodSize();
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy