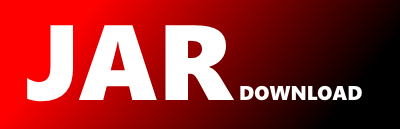
net.sourceforge.cilib.io.StandardDataTable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cilib-library Show documentation
Show all versions of cilib-library Show documentation
A library of composable components enabling simpler Computational Intelligence
/** __ __
* _____ _/ /_/ /_ Computational Intelligence Library (CIlib)
* / ___/ / / / __ \ (c) CIRG @ UP
* / /__/ / / / /_/ / http://cilib.net
* \___/_/_/_/_.___/
*/
package net.sourceforge.cilib.io;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import net.sourceforge.cilib.util.Cloneable;
/**
* Class represents the standard implementation of the {@link DataTable DataTable}
* where the underlying datastructures used are ArrayLists and rows are represented
* as a List of type T. Both the row and column type is the same.
* @param type of elements contained in a row.
*/
public class StandardDataTable implements DataTable, List> {
private List> dataTable;
private HashMap columnNames;
public StandardDataTable() {
dataTable = new ArrayList>();
columnNames = new HashMap();
}
/**
* Copy constructor.
* @param orig the datatable to copy.
*/
public StandardDataTable(StandardDataTable orig) {
dataTable = new ArrayList>(orig.getNumRows());
for (List row : orig) {
ArrayList newRow = new ArrayList(row.size());
for (T t : row) {
newRow.add(t.getClone());
}
dataTable.add(newRow);
}
columnNames = new HashMap();
int size = orig.getNumColums();
for (int i = 0; i < size; i++) {
this.setColumnName(i, orig.getColumnName(i));
}
}
/**
* Add a row to the table. The row data is added into a new list, thereby
* maintaining control of the row data structure (which is an ArrayList).
* @param rowData A list representing a row.
*/
@Override
public void addRow(List rowData) {
ArrayList row = new ArrayList(rowData.size());
row.addAll(rowData);
dataTable.add(row);
}
/**
* Add a column to the table. The column data is added unto the end of the
* existing lists.
* @param columnData A list representing a row.
*/
@Override
public void addColumn(List columnData) {
int size = columnData.size();
if (dataTable.size() == 0) {
for (int i = 0; i < size; i++) {
ArrayList row = new ArrayList();
row.add(columnData.get(i));
this.dataTable.add(row);
}
} else if (size != dataTable.size()) {
throw new UnsupportedOperationException("Cannot add column that is " +
"of different size than table columns.");
} else {
for (int i = 0; i < size; i++) {
dataTable.get(i).add(columnData.get(i));
}
}
}
/**
* Add multiple rows to the table. Calls the {@link #addRow(java.util.List) addRow}
* method for each of the multiple rows.
* @param multipleRowData a list of lists representing multiple rows.
*/
@Override
public void addRows(List> multipleRowData) {
for (List row : multipleRowData) {
this.addRow(row);
}
}
/**
* Add multiple columns to the table. Calls the {@link #addColumn(java.util.List) addColumn}
* method for each of the multiple columns.
* @param multipleColumnData a list of lists representing multiple columns.
*/
@Override
public void addColumns(List> multipleColumnData) {
for (List column : multipleColumnData) {
this.addColumn(column);
}
}
/**
* Removes and returns a row from the table.
* @param index the index of the row to remove.
* @return the row that has been removed.
*/
@Override
public List removeRow(int index) {
return dataTable.remove(index);
}
/**
* Gets a row from the table.
* @param index the required row.
* @return a new list containing the row elements.
*/
@Override
public List getRow(int index) {
return this.dataTable.get(index);
}
/**
* Sets a row in the datatable.
* @param index the index of the row to set.
* @param rowData the new row's data.
*/
@Override
public void setRow(int index, List rowData) {
this.dataTable.set(index, rowData);
}
/**
* Gets a column from the datatable as a new ArrayList.
* @param index the index of the column to retrieve.
* @return a new ArrayList containing the column elements.
*/
@Override
public List getColumn(int index) {
ArrayList column = new ArrayList(dataTable.size());
for (List row : dataTable) {
column.add(row.get(index));
}
return column;
}
/**
* Sets a column in the datatable. The elements are set individually in each row.
* @param index the index of the column to set.
* @param columnData the new column data.
*/
@Override
public void setColumn(int index, List columnData) {
int size = columnData.size();
if (size != dataTable.size()) {
throw new UnsupportedOperationException("Cannot set column that is " +
"of different size than table columns.");
}
for (int i = 0; i < size; i++) {
List row = dataTable.get(i);
row.set(index, columnData.get(i));
}
}
/**
* Gets the name of a column.
* @param index the index of the column name to retrieve.
* @return the column's name.
*/
@Override
public String getColumnName(int index) {
String name = columnNames.get(index);
if (name == null) {
return "";
}
return name;
}
/**
* Sets the name of a column.
* @param index the index of the column name to set.
* @param name the column's new name.
*/
@Override
public void setColumnName(int index, String name) {
columnNames.put(index, name);
}
/**
* {@inheritDoc }
*/
@Override
public List getColumnNames() {
ArrayList names = new ArrayList();
for (int i = 0; i < this.getNumColums(); i++) {
names.add(this.getColumnName(i));
}
return names;
}
/**
* {@inheritDoc }
*/
@Override
public void setColumnNames(List names) {
for (int i = 0; i < names.size(); i++) {
this.setColumnName(i, names.get(i));
}
}
/**
* Gets the number of rows in the table.
* @return the number of rows in the table.
*/
@Override
public int size() {
return dataTable.size();
}
/**
* Gets the number of rows in the table.
* @return the number of rows in the table.
*/
@Override
public int getNumRows() {
return dataTable.size();
}
/**
* Gets the number of columns in the table.
* @return the number of columns in the table.
*/
@Override
public int getNumColums() {
if (dataTable.isEmpty()) {
return 0;
}
return dataTable.get(0).size();
}
/**
* {@inheritDoc }
*/
@Override
public Iterator> iterator() {
return dataTable.iterator();
}
/**
* {@inheritDoc }
*/
@Override
public void clear() {
this.dataTable.clear();
this.columnNames.clear();
}
@Override
public Object getClone() {
return new StandardDataTable(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy