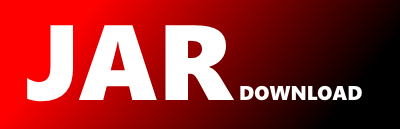
net.sourceforge.cilib.math.StatsTests Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cilib-library Show documentation
Show all versions of cilib-library Show documentation
A library of composable components enabling simpler Computational Intelligence
/** __ __
* _____ _/ /_/ /_ Computational Intelligence Library (CIlib)
* / ___/ / / / __ \ (c) CIRG @ UP
* / /__/ / / / /_/ / http://cilib.net
* \___/_/_/_/_.___/
*/
package net.sourceforge.cilib.math;
import fj.*;
import static fj.Function.*;
import static fj.Ord.*;
import fj.data.List;
import static fj.data.List.*;
import fj.data.Stream;
import static fj.function.Doubles.*;
import net.sourceforge.cilib.util.functions.Utils;
import static net.sourceforge.cilib.util.functions.Utils.pairwise;
public final class StatsTests {
private StatsTests() {}
public static >> P2 friedman(final double alpha, T a) {
return friedman(alpha).f(a);
}
public static >> List postHoc(final double alpha, final double statistic, T a) {
return postHoc(alpha, statistic).f(a);
}
public static F>, P2>
friedman(final double alpha) {
return new F>, P2>() {
@Override
public P2 f(Iterable extends Iterable> a) {
final List> ranks = iterableList(a)
.map(Stats.rank.andThen(Utils.iterableList()));
final int k = ranks.length();
final int m = ranks.isNotEmpty() ? iterableList(ranks.head()).length() : 0;
final double numerator = sum(ranks.foldLeft(pairwise(add), replicate(m, 0.0))
.map(new F() {
@Override
public Double f(Double a) {
return Math.pow(a - k * (m + 1) / 2, 2);
}
})) * (m - 1);
final double denominator = sum(ranks.map(flip(power).f(2.0).mapList())
.foldLeft(pairwise(add), replicate(m, 0.0)))
- k * m * (m + 1) * (m + 1) / 4;
return P.p(numerator / denominator, StatsTables.chisqrDistribution(m - 1, alpha));
}
};
}
public static F>, List>
postHoc(final double alpha, final double statistic) {
return new F>, List>() {
@Override
public List f(Iterable extends Iterable> a) {
final List> ranks = iterableList(a)
.map(Stats.rank.andThen(Utils.iterableList()));
final int k = ranks.length();
final int m = ranks.isNotEmpty() ? iterableList(ranks.head()).length() : 0;
Stream> sumOfRanks = ranks.foldLeft(pairwise(add), replicate(m, 0.0)).toStream().zipIndex();
final double denominator = sum(ranks.map(flip(power).f(2.0).mapList())
.foldLeft(pairwise(add), replicate(m, 0.0)))
- k * m * (m + 1) * (m + 1) / 4;
final double posthocStatistic = StatsTables.tDistribution(m - 1, alpha / 2.0);
final double posthocDenominator = Math.sqrt((2 * k)
* (1 - statistic / (k * (m - 1))) * denominator / ((k - 1) * (m - 1)));
final P2 best = sumOfRanks.sort(p2Ord(doubleOrd, intOrd)).head();
return Stream.unzip(sumOfRanks.filter(new F, Boolean>() {
@Override
public Boolean f(P2 a) {
return Math.abs(best._1() - a._1()) / posthocDenominator <= posthocStatistic;
}
}))._2().toList();
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy