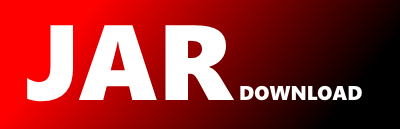
net.sourceforge.cilib.algorithm.population.SinglePopulationBasedAlgorithm Maven / Gradle / Ivy
/** __ __
* _____ _/ /_/ /_ Computational Intelligence Library (CIlib)
* / ___/ / / / __ \ (c) CIRG @ UP
* / /__/ / / / /_/ / http://cilib.net
* \___/_/_/_/_.___/
*/
package net.sourceforge.cilib.algorithm.population;
import net.sourceforge.cilib.algorithm.AbstractAlgorithm;
import net.sourceforge.cilib.algorithm.initialisation.PopulationInitialisationStrategy;
import net.sourceforge.cilib.coevolution.cooperative.ParticipatingAlgorithm;
import net.sourceforge.cilib.coevolution.cooperative.contributionselection.ContributionSelectionStrategy;
import net.sourceforge.cilib.coevolution.cooperative.contributionselection.ZeroContributionSelectionStrategy;
import net.sourceforge.cilib.entity.Entity;
import net.sourceforge.cilib.entity.topologies.GBestNeighbourhood;
import net.sourceforge.cilib.entity.topologies.Neighbourhood;
import fj.data.List;
import net.sourceforge.cilib.util.functions.Entities;
/**
* Base class for algorithms that focus on a single populations of entities.
* These types of algorithms typically include PSO , EC, ACO etc.
*/
public abstract class SinglePopulationBasedAlgorithm extends AbstractAlgorithm
implements HasTopology, HasNeighbourhood, ParticipatingAlgorithm {
private static final long serialVersionUID = -4095104893057340895L;
protected PopulationInitialisationStrategy initialisationStrategy;
protected List topology;
protected Neighbourhood neighbourhood;
protected ContributionSelectionStrategy contributionSelection;
/**
* Create an empty {@linkplain PopulationBasedAlgorithm}.
*/
protected SinglePopulationBasedAlgorithm() {
this.topology = List.nil();
this.neighbourhood = new GBestNeighbourhood<>();
this.contributionSelection = new ZeroContributionSelectionStrategy();
}
/**
* Create a copy of the provided instance.
* @param copy The {@linkplain PopulationBasedAlgorithm} to copy.
*/
protected SinglePopulationBasedAlgorithm(SinglePopulationBasedAlgorithm copy) {
super(copy);
this.initialisationStrategy = copy.initialisationStrategy.getClone();
this.topology = copy.topology.map(Entities.clone_());
this.neighbourhood = copy.neighbourhood;
this.contributionSelection = copy.contributionSelection.getClone();
}
/**
* {@inheritDoc}
*/
@Override
public abstract SinglePopulationBasedAlgorithm getClone();
/**
* Perform the iteration within the algorithm.
*/
@Override
protected abstract void algorithmIteration();
/**
* {@inheritDoc}
*/
@Override
public List getTopology() {
return topology;
}
/**
* Set the Topology for the population-based algorithm.
* @param topology The {@linkplain Topology} to be set.
*/
@Override
public void setTopology(List topology) {
this.topology = topology;
}
/**
* Get the currently set {@linkplain PopulationInitialisationStrategy}.
* @return The current {@linkplain PopulationInitialisationStrategy}.
*/
public PopulationInitialisationStrategy getInitialisationStrategy() {
return initialisationStrategy;
}
/**
* Set the {@linkplain PopulationInitialisationStrategy} to be used.
* @param initialisationStrategy The {@linkplain PopulationInitialisationStrategy} to use.
*/
public void setInitialisationStrategy(PopulationInitialisationStrategy initialisationStrategy) {
this.initialisationStrategy = initialisationStrategy;
}
/**
* {@inheritDoc}
*/
@Override
public void setContributionSelectionStrategy(ContributionSelectionStrategy strategy) {
this.contributionSelection = strategy;
}
/**
* {@inheritDoc}
*/
@Override
public ContributionSelectionStrategy getContributionSelectionStrategy() {
return contributionSelection;
}
public void setNeighbourhood(Neighbourhood f) {
this.neighbourhood = f;
}
@Override
public Neighbourhood getNeighbourhood() {
return this.neighbourhood;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy