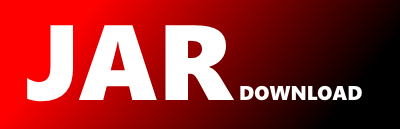
net.sourceforge.cilib.entity.Topologies Maven / Gradle / Ivy
/** __ __
* _____ _/ /_/ /_ Computational Intelligence Library (CIlib)
* / ___/ / / / __ \ (c) CIRG @ UP
* / /__/ / / / /_/ / http://cilib.net
* \___/_/_/_/_.___/
*/
package net.sourceforge.cilib.entity;
import java.util.Collections;
import java.util.Comparator;
import java.util.LinkedHashSet;
import java.util.Set;
import net.sourceforge.cilib.entity.comparator.AscendingFitnessComparator;
import net.sourceforge.cilib.entity.topologies.Neighbourhood;
import com.google.common.collect.Lists;
import fj.F;
import fj.data.List;
/**
* Topology related utilities.
*/
public final class Topologies {
private Topologies() {
}
/**
* Obtain the best entity from each neighbourhood and return them.
*/
public static Set getNeighbourhoodBestEntities(List p, Neighbourhood neighbourhood) {
return getNeighbourhoodBestEntities(p, neighbourhood, new AscendingFitnessComparator());
}
/**
* Gather the best entity of each neighbourhood (in this {@link Topology}) in a
* {@link Set} (duplicates are not allowed) and return them. A single {@link Entity} may
* dominate in more than one neighbourhood, but we just want unique entities.
*/
public static Set getNeighbourhoodBestEntities(List p,
Neighbourhood neighbourhood, Comparator super E> c) {
Set nBests = new LinkedHashSet(p.length());
F> partial = neighbourhood.f(p);
for (E e : p) {
E best = getBestEntity(partial.f(e), c);
if (best != null) {
nBests.add(best);
}
}
return nBests;
}
/**
* Returns the current best entity from a given topology based on the current
* fitness of the entities.
*/
public static T getBestEntity(List p) {
return getBestEntity(p, new AscendingFitnessComparator());
}
/**
* Returns an entity from a given topology using the given comparator.
*/
public static T getBestEntity(List p, Comparator super T> c) {
if (p.isEmpty()) {
return null;
}
java.util.List tmp = Lists.newArrayList(p);
Collections.sort(tmp, c);
return tmp.get(tmp.size() - 1);
}
/**
* Returns the current best entity from the neighbourhood of an entity
* in a topology based on the current fitness of the entities.
*/
public static T getNeighbourhoodBest(List p, T e, Neighbourhood neighbourhood) {
return getNeighbourhoodBest(p, e, neighbourhood, new AscendingFitnessComparator());
}
/**
* Returns an entity from the neighbourhood of an entity in a topology using
* the given comparator.
*/
public static T getNeighbourhoodBest(List p, T e, Neighbourhood neighbourhood, Comparator super T> c) {
return getBestEntity(neighbourhood.f(p, e), c);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy