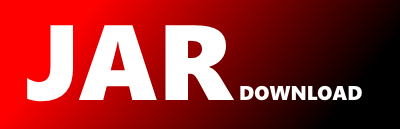
net.sourceforge.cilib.entity.topologies.VonNeumannNeighbourhood Maven / Gradle / Ivy
package net.sourceforge.cilib.entity.topologies;
import com.google.common.collect.Lists;
import fj.F;
import fj.data.List;
public class VonNeumannNeighbourhood extends Neighbourhood {
private E find(List list, int n, int r, int c) {
return list.index(r * n + c);
}
@Override
public List f(final List list, final E target) {
final int np = list.length();
final int index = Lists.newArrayList(list).indexOf(target);
final int sqSide = (int) Math.round(Math.sqrt(np));
final int nRows = (int) Math.ceil(np / (double) sqSide);
final int row = index / sqSide;
final int col = index % sqSide;
final F colsInRow = new F() {
@Override
public Integer f(Integer r) {
return r == nRows - 1 ? np - r * sqSide : sqSide;
}
};
final E north = find(list, sqSide, (row - 1 + nRows) % nRows - ((col >= colsInRow.f((row - 1 + nRows) % nRows)) ? 1 : 0), col);
final E south = find(list, sqSide, (row + 1) % nRows - ((col >= colsInRow.f((row + 1) % nRows)) ? sqSide : 0), col);
final E east = find(list, sqSide, row, (col + 1) % colsInRow.f(row));
final E west = find(list, sqSide, row, (col - 1 + colsInRow.f(row)) % colsInRow.f(row));
return List.list(target, north, east, south, west);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy