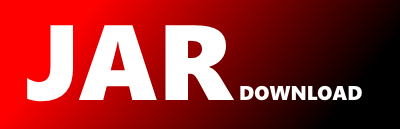
net.sourceforge.cilib.niching.VectorBasedFunctions Maven / Gradle / Ivy
/** __ __
* _____ _/ /_/ /_ Computational Intelligence Library (CIlib)
* / ___/ / / / __ \ (c) CIRG @ UP
* / /__/ / / / /_/ / http://cilib.net
* \___/_/_/_/_.___/
*/
package net.sourceforge.cilib.niching;
import fj.F;
import fj.F2;
import fj.Ordering;
import net.sourceforge.cilib.pso.particle.Particle;
import net.sourceforge.cilib.type.types.container.Vector;
import net.sourceforge.cilib.util.distancemeasure.DistanceMeasure;
public final class VectorBasedFunctions {
private VectorBasedFunctions() {}
public static F dot(final Particle nBest) {
return new F() {
@Override
public Double f(Particle p) {
Vector gBest = (Vector) nBest.getBestPosition();
Vector vg = gBest.subtract((Vector) p.getCandidateSolution());
Vector vp = ((Vector) p.getBestPosition()).subtract((Vector) p.getCandidateSolution());
return vp.dot(vg);
}
};
}
public static F> sortByDistance(final Particle nBest, final DistanceMeasure distance) {
return new F2() {
@Override
public Ordering f(Particle a, Particle b) {
double aDist = distance.distance(a.getCandidateSolution(), nBest.getBestPosition());
double bDist = distance.distance(b.getCandidateSolution(), nBest.getBestPosition());
return Ordering.values()[Double.compare(aDist, bDist) + 1];
}
}.curry();
}
public static F2 equalParticle = new F2() {
@Override
public Boolean f(Particle a, Particle b) {
return a.getBestPosition().equals(b.getBestPosition())
&& a.getBestFitness().equals(b.getBestFitness())
&& a.getCandidateSolution().equals(b.getCandidateSolution())
&& a.getFitness().equals(b.getFitness());
}
};
public static F filter(final DistanceMeasure distanceMeasure, final Particle nBest, final double nRadius) {
return new F() {
@Override
public Boolean f(Particle p) {
double pRadius = distanceMeasure.distance(p.getCandidateSolution(), nBest.getBestPosition());
return pRadius < nRadius && dot(nBest).f(p) > 0;
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy