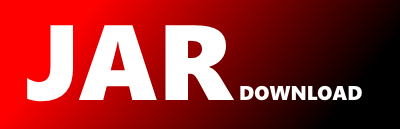
net.sourceforge.cilib.pso.dynamic.responsestrategies.ReevaluationReactionStrategy Maven / Gradle / Ivy
/** __ __
* _____ _/ /_/ /_ Computational Intelligence Library (CIlib)
* / ___/ / / / __ \ (c) CIRG @ UP
* / /__/ / / / /_/ / http://cilib.net
* \___/_/_/_/_.___/
*/
package net.sourceforge.cilib.pso.dynamic.responsestrategies;
import java.util.List;
import net.sourceforge.cilib.algorithm.population.SinglePopulationBasedAlgorithm;
import net.sourceforge.cilib.entity.Entity;
import net.sourceforge.cilib.pso.PSO;
import net.sourceforge.cilib.pso.particle.Particle;
import net.sourceforge.cilib.pso.particle.StandardParticle;
import net.sourceforge.cilib.util.selection.Samples;
import net.sourceforge.cilib.util.selection.recipes.RandomSelector;
/**
* This reaction strategy reevaluates the specified
* {@link #setReevaluationRatio(double) ratio} of randomly chosen entities in
* the given {@link Topology}.
*
* @param some {@link PopulationBasedAlgorithm population based algorithm}
*/
public class ReevaluationReactionStrategy extends EnvironmentChangeResponseStrategy {
private static final long serialVersionUID = -5549918743502730714L;
protected double reevaluationRatio = 0.0;
public ReevaluationReactionStrategy() {
reevaluationRatio = 0.1;
}
public ReevaluationReactionStrategy(ReevaluationReactionStrategy rhs) {
super(rhs);
reevaluationRatio = rhs.reevaluationRatio;
}
@Override
public ReevaluationReactionStrategy getClone() {
return new ReevaluationReactionStrategy(this);
}
/**
* Just reevaluate the {@link Entity entities} inside the topology. An
* entity's implementation should handle updating anything else that is
* necessary, e.g. a {@link StandardParticle}'s
* personal best position
in the case of {@link PSO}.
*
* {@inheritDoc}
*/
@Override
protected > void performReaction(
A algorithm) {
fj.data.List extends Entity> entities = algorithm.getTopology();
int reevaluateCount = (int) Math.floor(reevaluationRatio * entities.length());
reevaluate(entities, reevaluateCount);
}
/**
* Reevaluate a specified percentage of the given entities.
*
* @param entities a {@link List} of entities that should be considered for
* reevaluation
* @param reevaluateCount an int specifying how many entities should be
* reevaluated
*/
protected void reevaluate(fj.data.List extends Entity> entities, int reevaluateCount) {
RandomSelector selector = new RandomSelector();
List extends Entity> subList = selector.on(entities).select(Samples.first(reevaluateCount));
for (Entity entity : subList) {
// FIXME: does not reevaluate the _best_ position.
entity.calculateFitness();
}
}
/**
* Set the ratio of entities that should be reevaluated.
*
* @param rer a double value in the range (0.0, 1.0)
* @throws {@link IllegalArgumentException} when the ratio is not within the
* above mentioned range
*/
public void setReevaluationRatio(double rer) {
if (rer < 0.0 || rer > 1.0) {
throw new IllegalArgumentException("The reevaluationRatio must be in the range (0.0, 1.0)");
}
reevaluationRatio = rer;
}
/**
* Get the ratio of entities that should be reevaluated.
*
* @return the ratio of entities that should be reevaluated
*/
public double getReevaluationRatio() {
return reevaluationRatio;
}
}