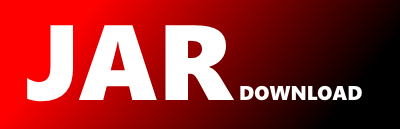
net.sourceforge.cilib.pso.iterationstrategies.moo.NonDominatedMOOSynchronousIterationStrategy Maven / Gradle / Ivy
/** __ __
* _____ _/ /_/ /_ Computational Intelligence Library (CIlib)
* / ___/ / / / __ \ (c) CIRG @ UP
* / /__/ / / / /_/ / http://cilib.net
* \___/_/_/_/_.___/
*/
package net.sourceforge.cilib.pso.iterationstrategies.moo;
import fj.data.List;
import net.sourceforge.cilib.algorithm.AbstractAlgorithm;
import net.sourceforge.cilib.algorithm.population.AbstractIterationStrategy;
import net.sourceforge.cilib.pso.particle.Particle;
import net.sourceforge.cilib.problem.Problem;
import net.sourceforge.cilib.problem.solution.MOFitness;
import net.sourceforge.cilib.problem.solution.OptimisationSolution;
import net.sourceforge.cilib.pso.PSO;
/**
* Implementation of the synchronous iteration strategy for PSO.
*
*/
public class NonDominatedMOOSynchronousIterationStrategy extends AbstractIterationStrategy {
/**
* {@inheritDoc}
*/
@Override
public NonDominatedMOOSynchronousIterationStrategy getClone() {
return this;
}
/**
* This is an Synchronous strategy:
*
*
* - For all particles:
* - Update the particle velocity
* - Update the particle position
* - For all particles:
* - Calculate the particle fitness
* - For all particles in the current particle's neighbourhood:
* - Update the neighbourhood best
*
*
* @param pso The {@link PSO} to have an iteration applied.
*/
@Override
public void performIteration(PSO pso) {
List topology = pso.getTopology();
for (Particle current : topology) {
current.updateVelocity();
current.updatePosition(); // TODO: replace with visitor (will simplify particle interface)
boundaryConstraint.enforce(current);
}
Problem problem = AbstractAlgorithm.getAlgorithmList().get(0).getOptimisationProblem();
for (Particle current : topology) {
current.calculateFitness();
for (Particle other : pso.getNeighbourhood().f(topology, current)) {
Particle p1 = current.getNeighbourhoodBest().getClone();
Particle p2 = other.getNeighbourhoodBest().getClone();
OptimisationSolution s1 = new OptimisationSolution(p1.getCandidateSolution().getClone(), problem.getFitness(p1.getCandidateSolution().getClone()));
OptimisationSolution s2 = new OptimisationSolution(p2.getCandidateSolution().getClone(), problem.getFitness(p2.getCandidateSolution().getClone()));
MOFitness fitness1 = (MOFitness)s1.getFitness();
MOFitness fitness2 = (MOFitness)s2.getFitness();
if (fitness1.compareTo(fitness2) >= 0) {
other.setNeighbourhoodBest(current);
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy