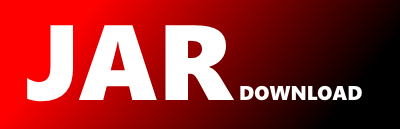
net.sourceforge.cilib.util.functions.Entities Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cilib-library Show documentation
Show all versions of cilib-library Show documentation
A library of composable components enabling simpler Computational Intelligence
The newest version!
/** __ __
* _____ _/ /_/ /_ Computational Intelligence Library (CIlib)
* / ___/ / / / __ \ (c) CIRG @ UP
* / /__/ / / / /_/ / http://cilib.net
* \___/_/_/_/_.___/
*/
package net.sourceforge.cilib.util.functions;
import com.google.common.collect.Lists;
import fj.F;
import fj.F2;
import net.sourceforge.cilib.entity.Entity;
import net.sourceforge.cilib.entity.MemoryBasedEntity;
import net.sourceforge.cilib.entity.SocialEntity;
import net.sourceforge.cilib.problem.Problem;
import net.sourceforge.cilib.problem.solution.Fitness;
import net.sourceforge.cilib.type.types.container.StructuredType;
import net.sourceforge.cilib.util.Cloneable;
public final class Entities {
private Entities() {}
public static F clone_() {
return new F() {
@Override
public E f(E e) {
return (E) e.getClone();
}
};
}
public static F getCandidateSolution() {
return new F() {
@Override
public StructuredType f(E a) {
return a.getCandidateSolution();
}
};
}
public static java.util.List getCandidateSolutions(java.util.List list) {
return Lists.newArrayList(fj.data.List.iterableList(list).map(new F() {
@Override
public E f(T e) {
return (E) e.getCandidateSolution();
}
}));
}
public static F2 setCandidateSolution() {
return new F2() {
@Override
public E f(StructuredType a, E b) {
b.setCandidateSolution(a);
return b;
}
};
}
public static F evaluate() {
return new F() {
@Override
public E f(E a) {
a.calculateFitness();
return a;
}
};
}
public static F getFitness() {
return new F() {
@Override
public Fitness f(E a) {
return a.getFitness();
}
};
}
public static F getBestFitness() {
return new F() {
@Override
public Fitness f(E a) {
return a.getBestFitness();
}
};
}
public static F reinitialise() {
return new F() {
@Override
public E f(E a) {
a.reinitialise();
return a;
}
};
}
public static F2 initialise() {
return new F2() {
@Override
public E f(Problem a, E b) {
b.initialise(a);
return b;
}
};
}
public static F getBestPosition() {
return new F() {
@Override
public StructuredType f(E a) {
return a.getBestPosition();
}
};
}
public static F getSocialFitness() {
return new F() {
@Override
public Fitness f(E a) {
return a.getSocialFitness();
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy