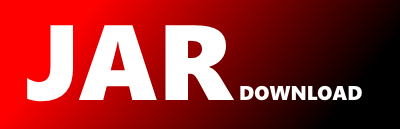
net.sourceforge.cilib.util.functions.Populations Maven / Gradle / Ivy
/** __ __
* _____ _/ /_/ /_ Computational Intelligence Library (CIlib)
* / ___/ / / / __ \ (c) CIRG @ UP
* / /__/ / / / /_/ / http://cilib.net
* \___/_/_/_/_.___/
*/
package net.sourceforge.cilib.util.functions;
import net.sourceforge.cilib.algorithm.population.SinglePopulationBasedAlgorithm;
import net.sourceforge.cilib.entity.Entity;
import net.sourceforge.cilib.pso.particle.Particle;
import net.sourceforge.cilib.pso.particle.ParticleBehavior;
import fj.F;
import fj.F2;
import fj.data.List;
public final class Populations {
/**
* Returns an empty population of the given population type;
*/
public static F
emptyPopulation() {
return new F
() {
@Override
public P f(P a) {
P tmp = (P) a.getClone();
tmp.setTopology(List.nil());
return tmp;
}
};
}
/**
* Converts a swarm into a list of single entity populations.
*/
public static
F
> populationToAlgorithms() {
return new F
>() {
@Override
public List
f(final P a) {
return Populations.
entitiesToAlgorithms().f((Iterable) a.getTopology(), a);
}
};
}
/**
* Converts a single entity to a population of the given type.
*/
public static F2 entityToAlgorithm() {
return new F2() {
@Override
public P f(E e, P p) {
P tmp = (P) p.getClone();
tmp.setTopology(List.single(e));
//((fj.data.List) tmp.getTopology()).add(e);
return tmp;
}
};
}
/**
* Converts a list of entities into single entity populations.
*/
public static F2, P, List> entitiesToAlgorithms() {
return new F2, P, List>() {
@Override
public List
f(Iterable a, P b) {
return List.iterableList(a).map(Populations.entityToAlgorithm().flip().f(b));
}
};
}
/**
* Makes sure a swarm has a neighbourhood best and the correct particle behavior.
*
* @param pb The particle behavior to give each entity in the swarm.
*
* @return The enforced swarm.
*/
public static
F
enforceTopology(final ParticleBehavior pb) {
return new F
() {
@Override
public P f(P a) {
P tmp = (P) a.getClone();
if (!tmp.getTopology().isEmpty() && tmp.getTopology().head() instanceof Particle) {
List local = tmp.getTopology();
for (Entity e : local) {
Particle p = (Particle) e;
p.setParticleBehavior(pb);
}
}
return tmp;
}
};
}
}