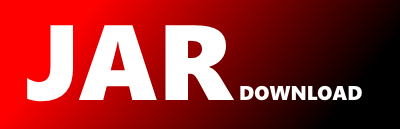
net.sourceforge.cilib.util.selection.Selection Maven / Gradle / Ivy
/** __ __
* _____ _/ /_/ /_ Computational Intelligence Library (CIlib)
* / ___/ / / / __ \ (c) CIRG @ UP
* / /__/ / / / /_/ / http://cilib.net
* \___/_/_/_/_.___/
*/
package net.sourceforge.cilib.util.selection;
import static com.google.common.base.Preconditions.checkArgument;
import static com.google.common.base.Preconditions.checkNotNull;
import static com.google.common.base.Preconditions.checkState;
import com.google.common.base.Predicate;
import com.google.common.collect.Iterables;
import com.google.common.collect.Lists;
import java.util.List;
import net.sourceforge.cilib.util.selection.arrangement.Arrangement;
import net.sourceforge.cilib.util.selection.weighting.Weighting;
public final class Selection implements WeighableSelection, PartialSelection {
private final Object[] elements;
// need to hide this method somehow
public static Selection copyOf(Iterable iterable) {
checkNotNull(iterable);
return new Selection(copyOfInternal(iterable));
}
// Check array size
private static Object[] copyOfInternal(Iterable iterable) {
checkArgument(Iterables.size(iterable) >= 1, "Attempting to create a "
+ "selection on an empty collection is not valid.");
List list = Lists.newArrayList(iterable);
return list.toArray();
}
Selection(Object[] array) {
this.elements = array;
}
@Override
public Selection exclude(T... items) {
return exclude(Lists.newArrayList(items));
}
@Override
public Selection exclude(Iterable iterable) {
List list = Lists.newArrayList(iterable);
List result = Lists.newArrayList();
for (Object o : elements) {
if (!list.contains(o)) {
result.add((T) o);
}
}
return new Selection(copyOfInternal(result));
}
@Override
public WeightedSelection weigh(Weighting weighing) {
List list = (List) Lists.newArrayList(elements);
List result = Lists.newArrayList(weighing.weigh(list));
return new WeightedSelection(result.toArray(new WeightedObject[]{}));
}
@Override
public Selection orderBy(Arrangement arrangement) {
List list = (List) Lists.newArrayList(elements);
List result = Lists.newArrayList(arrangement.arrange((Iterable) list));
return new Selection(result.toArray());
}
/**
* TODO: this can be made more efficient: no need to apply the predicate if not needed.
*/
@Override
public Selection filter(Predicate super T> predicate) {
List result = Lists.newArrayList();
for (Object o : elements) {
if (!predicate.apply((T) o)) {
result.add((T) o);
}
}
return new Selection(copyOfInternal(result));
}
@Override
public T select() {
checkState(elements.length >= 1, "Selection is invalid, please verify selection state.");
return (T) elements[0];
}
@Override
public List select(Samples sample) {
return (List) sample.sample(Lists.newArrayList(this.elements));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy