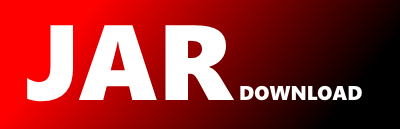
net.codebox.readableregex.Token Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of readable-regex Show documentation
Show all versions of readable-regex Show documentation
This library provides a way to make complex regular expressions in Java code more readable.
The newest version!
package net.codebox.readableregex;
import java.util.regex.Pattern;
/**
* Class representing part of a regular expression.
*/
public class Token {
private Quantifier quantifier;
private boolean requiresBrackets;
private String tokenString;
protected Token(String tokenString, boolean requiresBrackets, Quantifier quantifier) {
this.tokenString = tokenString;
this.requiresBrackets = requiresBrackets;
this.quantifier = quantifier;
}
protected Token(Token token, Quantifier quantifier) {
this.tokenString = token.tokenString;
this.requiresBrackets = token.requiresBrackets;
this.quantifier = quantifier;
}
private static char[] SPECIAL_CHARS = new char[]{'\\', '[', '^', '$', '.', '|', '?', '*', '+', '(', ')', '{', '}'};
@Override
public final String toString(){
if (quantifier == null){
return tokenString;
} else {
if (requiresBrackets){
return "(" + tokenString + ")" + quantifier.toString();
} else {
return tokenString + quantifier.toString();
}
}
}
/**
* Encloses the specified series of tokens in a pair of brackets, creating a capturing group.
*
* @param tokens the tokens to be enclosed
*
* @return a new Token instance
*/
public static Token groupOf(final Token... tokens) {
return new Token(
"(" + RegExBuilder.build(tokens) + ")", false, null
);
}
/**
* Encloses the specified series of tokens in a pair of brackets, creating a non-capturing group.
*
* @param tokens the tokens to be enclosed
*
* @return a new Token instance
*/
public static Token nonCapturingGroup(final Token... tokens) {
return new Token(
"(?:" + RegExBuilder.build(tokens) + ")", false, null
);
}
/**
* Encloses the specified series of tokens in a pair of brackets, creating an independent non-capturing group.
*
* @param tokens the tokens to be enclosed
*
* @return a new Token instance
*/
public static Token independentNonCapturingGroup(final Token... tokens) {
return new Token(
"(?>" + RegExBuilder.build(tokens) + ")", false, null
);
}
/**
* Creates a token that specifies a zero-width positive lookahead.
*
* @param tokens the tokens to be used to define the lookahead
*
* @return a new Token instance
*/
public static Token positiveLookAhead(final Token... tokens) {
return new Token(
"(?=" + RegExBuilder.build(tokens) + ")", false, null
);
}
/**
* Creates a token that specifies a zero-width negative lookahead.
*
* @param tokens the tokens to be used to define the lookahead
*
* @return a new Token instance
*/
public static Token negativeLookAhead(final Token... tokens) {
return new Token(
"(?!" + RegExBuilder.build(tokens) + ")", false, null
);
}
/**
* Creates a token that specifies a zero-width positive look-behind.
*
* @param tokens the tokens to be used to define the look-behind
*
* @return a new Token instance
*/
public static Token positiveLookBehind(final Token... tokens) {
return new Token(
"(?<=" + RegExBuilder.build(tokens) + ")", false, null
);
}
/**
* Creates a token that specifies a zero-width negative look-behind.
*
* @param tokens the tokens to be used to define the look-behind
*
* @return a new Token instance
*/
public static Token negativeLookBehind(final Token... tokens) {
return new Token(
"(? 1, null
);
}
/**
* Creates a token that matches any single character that does not correspond to any of
* the specified CharacterTokenPart instances.
*
* @param characterTokens an array of CharacterTokenPart objects which must not be matched
*
* @return a new Token instance
*/
public static Token anyCharacterExcept(final CharacterTokenPart... characterTokens){
final String tokenString;
setFirstAndLastParts(characterTokens);
String txt = Utils.appendObjects(characterTokens);
tokenString = "[^" + txt + "]";
return new Token(tokenString, false, null);
}
/**
* Creates a token that matches any single character that corresponds to any of the
* specified CharacterTokenPart instances.
*
* @param characterTokens an array of CharacterTokenPart objects, one of which must be matched
*
* @return a new Token instance
*/
public static Token anyOneOf(final CharacterTokenPart... characterTokens){
final String tokenString;
setFirstAndLastParts(characterTokens);
String txt = Utils.appendObjects(characterTokens);
tokenString = "[" + txt + "]";
return new Token(tokenString, false, null);
}
private static void setFirstAndLastParts(final CharacterTokenPart... characterTokens){
final int count = characterTokens.length;
for(int i=0; i 0){
sb.append("|");
}
sb.append(token.toString());
}
final boolean requiresBrackets;
if (tokens.length > 1){
requiresBrackets = true;
} else {
requiresBrackets = tokens[0].requiresBrackets;
}
return new Token(sb.toString(), requiresBrackets, null);
}
/**
* An alias for the 'or' method.
*
* @param tokens an array of Tokens, one of which must be matched
*
* @return a new Token instance
*/
public static Token oneOf(final Token... tokens) {
return or(tokens);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy