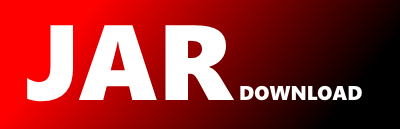
net.codecrete.qrbill.generator.SwicoS1Encoder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qrbill-generator Show documentation
Show all versions of qrbill-generator Show documentation
Java library for generating Swiss QR bills
//
// Swiss QR Bill Generator
// Copyright (c) 2018 Manuel Bleichenbacher
// Licensed under MIT License
// https://opensource.org/licenses/MIT
//
package net.codecrete.qrbill.generator;
import net.codecrete.qrbill.generator.SwicoBillInformation.PaymentCondition;
import net.codecrete.qrbill.generator.SwicoBillInformation.RateDetail;
import java.math.BigDecimal;
import java.text.DecimalFormat;
import java.text.DecimalFormatSymbols;
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.util.List;
import java.util.Locale;
/**
* Encodes structured bill information according to Swico S1 syntax.
*
* The encoded bill information can be used in a Swiss QR bill
* in the field {@code StrdBkgInfo}.
*
*
* Also see http://swiss-qr-invoice.org/downloads/qr-bill-s1-syntax-de.pdf.
*
*/
class SwicoS1Encoder {
private SwicoS1Encoder() {
// may not instantiate
}
/**
* Encodes the specified bill information.
*
* @param billInfo the bill information
* @return encoded bill information text
*/
static String encode(SwicoBillInformation billInfo) {
StringBuilder sb = new StringBuilder();
sb.append("//S1");
if (billInfo.getInvoiceNumber() != null)
sb.append("/10/").append(escapedText(billInfo.getInvoiceNumber()));
if (billInfo.getInvoiceDate() != null)
sb.append("/11/").append(s1Date(billInfo.getInvoiceDate()));
if (billInfo.getCustomerReference() != null)
sb.append("/20/").append(escapedText(billInfo.getCustomerReference()));
if (billInfo.getVatNumber() != null)
sb.append("/30/").append(escapedText(billInfo.getVatNumber()));
if (billInfo.getVatDate() != null) {
sb.append("/31/").append(s1Date(billInfo.getVatDate()));
} else if (billInfo.getVatStartDate() != null && billInfo.getVatEndDate() != null) {
sb.append("/31/")
.append(s1Date(billInfo.getVatStartDate()))
.append(s1Date(billInfo.getVatEndDate()));
}
if (billInfo.getVatRate() != null) {
sb.append("/32/").append(s1Number(billInfo.getVatRate()));
} else if (billInfo.getVatRateDetails() != null && !billInfo.getVatRateDetails().isEmpty()) {
sb.append("/32/");
appendRateDetailTupleList(sb, billInfo.getVatRateDetails());
}
if (billInfo.getVatImportTaxes() != null && !billInfo.getVatImportTaxes().isEmpty()) {
sb.append("/33/");
appendRateDetailTupleList(sb, billInfo.getVatImportTaxes());
}
if (billInfo.getPaymentConditions() != null && !billInfo.getPaymentConditions().isEmpty()) {
sb.append("/40/");
appendConditionTupleList(sb, billInfo.getPaymentConditions());
}
return sb.length() > 4 ? sb.toString() : null;
}
private static String escapedText(String text) {
return text.replace("\\", "\\\\").replace("/", "\\/");
}
private static final DateTimeFormatter SWICO_DATE_FORMAT
= DateTimeFormatter.ofPattern("yyMMdd", Locale.UK);
private static String s1Date(LocalDate date) {
return date.format(SWICO_DATE_FORMAT);
}
private static final DecimalFormat SWICO_NUMBER_FORMAT
= new DecimalFormat("0.###", new DecimalFormatSymbols(Locale.UK));
private static String s1Number(BigDecimal num) {
return SWICO_NUMBER_FORMAT.format(num);
}
private static void appendRateDetailTupleList(StringBuilder sb, List list) {
boolean isFirst = true;
for (RateDetail e : list) {
if (!isFirst)
sb.append(";");
else
isFirst = false;
sb.append(s1Number(e.getRate())).append(":").append(s1Number(e.getAmount()));
}
}
private static void appendConditionTupleList(StringBuilder sb, List list) {
boolean isFirst = true;
for (PaymentCondition e : list) {
if (!isFirst)
sb.append(";");
else
isFirst = false;
sb.append(s1Number(e.getDiscount())).append(":").append(e.getDays());
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy