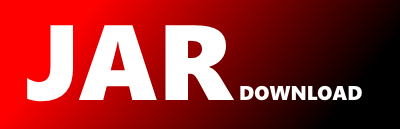
net.codecrete.qrbill.generator.ValidationMessage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qrbill-generator Show documentation
Show all versions of qrbill-generator Show documentation
Java library for generating Swiss QR bills
//
// Swiss QR Bill Generator
// Copyright (c) 2017 Manuel Bleichenbacher
// Licensed under MIT License
// https://opensource.org/licenses/MIT
//
package net.codecrete.qrbill.generator;
import java.io.Serializable;
/**
* QR bill validation message.
*/
public class ValidationMessage implements Serializable {
private static final long serialVersionUID = -243381506848710081L;
/**
* Type of validation message
*/
public enum Type {
/**
* Warning.
*
* A warning does not prevent the QR bill from being generated. Warnings usually
* indicate that data was truncated or otherwise modified.
*
*/
WARNING,
/**
* Error.
*
* Errors prevent the QR bill from being generated.
*
*/
ERROR
}
private Type type;
private String field;
private String messageKey;
private String[] messageParameters;
/**
* Constructs a new validation message.
*/
public ValidationMessage() {
}
/**
* Constructs a new validation message with the given values.
*
* @param type the message type
* @param field the affect field
* @param messageKey the language-neutral key of the message
*/
public ValidationMessage(Type type, String field, String messageKey) {
this.type = type;
this.field = field;
this.messageKey = messageKey;
}
/**
* Constructs a new validation message with the given values.
*
* @param type the message type
* @param field the affect field
* @param messageKey the language-neutral key of the message
* @param messageParameters variable text parts that will be inserted into the
* localized message
*/
public ValidationMessage(Type type, String field, String messageKey, String[] messageParameters) {
this.type = type;
this.field = field;
this.messageKey = messageKey;
this.messageParameters = messageParameters;
}
/**
* Gets the type of message
*
* @return the message type
*/
public Type getType() {
return type;
}
/**
* Sets the type of message
*
* @param type message type
*/
public void setType(Type type) {
this.type = type;
}
/**
* Gets the name of the affected field.
*
* All field names are available as constants in {@link Bill}. Examples are:
* "account", "creditor.street"
*
*
* @return the field name
*/
public String getField() {
return field;
}
/**
* Sets the name of the affected field.
*
* All field names are available as constants in {@link Bill}. Examples are:
* "account", "creditor.street"
*
*
* @param field the field name
*/
public void setField(String field) {
this.field = field;
}
/**
* Gets the language neutral key of the message.
*
* @return the message key
*/
public String getMessageKey() {
return messageKey;
}
/**
* Sets the language neutral key of the message.
*
* @param messageKey the message key
*/
public void setMessageKey(String messageKey) {
this.messageKey = messageKey;
}
/**
* Gets additional message parameters (text) that are inserted into the
* localized message.
*
* @return the additional message parameters
*/
public String[] getMessageParameters() {
return messageParameters;
}
/**
* Sets additional message parameters (text) that are inserted into the
* localized message.
*
* @param messageParameters the additional message parameters
*/
public void setMessageParameters(String[] messageParameters) {
this.messageParameters = messageParameters;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy