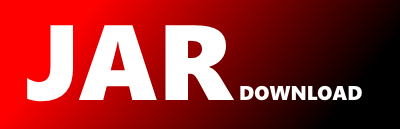
ome.xml.meta.MetadataConverter Maven / Gradle / Ivy
Show all versions of ome-xml Show documentation
/*
* #%L
* OME-XML Java library for working with OME-XML metadata structures.
* %%
* Copyright (C) 2006 - 2016 Open Microscopy Environment:
* - Massachusetts Institute of Technology
* - National Institutes of Health
* - University of Dundee
* - Board of Regents of the University of Wisconsin-Madison
* - Glencoe Software, Inc.
* %%
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDERS OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
* #L%
*/
package ome.xml.meta;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import ome.xml.model.*;
import ome.xml.model.enums.*;
import ome.xml.model.primitives.*;
import ome.units.quantity.ElectricPotential;
import ome.units.quantity.Frequency;
import ome.units.quantity.Length;
import ome.units.quantity.Power;
import ome.units.quantity.Pressure;
import ome.units.quantity.Temperature;
import ome.units.quantity.Time;
import ome.units.UNITS;
/**
* A utility class containing a method for piping a source
* {@link MetadataRetrieve} object into a destination {@link MetadataStore}.
*
* This technique allows conversion between two different storage media.
* For example, it can be used to convert an OMEROMetadataStore
* (OMERO's metadata store implementation) into an
* {@link ome.xml.meta.OMEXMLMetadataImpl}, thus generating OME-XML from
* information in an OMERO database.
*
* @author Curtis Rueden ctrueden at wisc.edu
*/
public final class MetadataConverter {
// -- Constructor --
/**
* Private constructor; all methods in MetadataConverter
* are static, so this should not be called.
*/
private MetadataConverter() { }
// -- MetadataConverter API methods --
/**
* Copies information from a metadata retrieval object
* (source) into a metadata store (destination).
*/
public static void convertMetadata(MetadataRetrieve src, MetadataStore dest) {
convertBooleanAnnotations(src, dest);
convertCommentAnnotations(src, dest);
convertDoubleAnnotations(src, dest);
convertFileAnnotations(src, dest);
convertListAnnotations(src, dest);
convertLongAnnotations(src, dest);
convertMapAnnotations(src, dest);
convertTagAnnotations(src, dest);
convertTermAnnotations(src, dest);
convertTimestampAnnotations(src, dest);
convertXMLAnnotations(src, dest);
convertROIs(src, dest);
List lightSourceIds = convertInstruments(src, dest);
convertExperimenters(src, dest);
convertExperimenterGroups(src, dest);
convertExperiments(src, dest);
convertImages(src, dest, lightSourceIds);
convertPlates(src, dest);
convertScreens(src, dest);
convertDatasets(src, dest);
convertProjects(src, dest);
convertFolders(src, dest);
convertRootAttributes(src, dest);
}
// -- Helper methods --
/**
* Convert all BooleanAnnotation attributes.
* @param src the MetadataRetrieve from which to copy
* @param dest the MetadataStore to which to copy
*/
private static void convertBooleanAnnotations(MetadataRetrieve src,
MetadataStore dest)
{
int booleanAnnotationCount = 0;
try {
booleanAnnotationCount = src.getBooleanAnnotationCount();
}
catch (NullPointerException e) { }
for (int i=0; i lightSourceIds) {
int imageCount = 0;
try {
imageCount = src.getImageCount();
}
catch (NullPointerException e) { }
for (int i=0; i map = src.getImagingEnvironmentMap(i);
dest.setImagingEnvironmentMap(map, i);
}
catch (NullPointerException e) { }
try {
Temperature temperature = src.getImagingEnvironmentTemperature(i);
dest.setImagingEnvironmentTemperature(temperature, i);
}
catch (NullPointerException e) { }
try {
String objectiveID = src.getObjectiveSettingsID(i);
if (objectiveID != null) {
dest.setObjectiveSettingsID(objectiveID, i);
try {
Double correction = src.getObjectiveSettingsCorrectionCollar(i);
dest.setObjectiveSettingsCorrectionCollar(correction, i);
}
catch (NullPointerException e) { }
try {
Medium medium = src.getObjectiveSettingsMedium(i);
dest.setObjectiveSettingsMedium(medium, i);
}
catch (NullPointerException e) { }
try {
Double refractiveIndex = src.getObjectiveSettingsRefractiveIndex(i);
dest.setObjectiveSettingsRefractiveIndex(refractiveIndex, i);
}
catch (NullPointerException e) { }
}
}
catch (NullPointerException e) { }
try {
String stageLabelName = src.getStageLabelName(i);
if (stageLabelName != null) {
dest.setStageLabelName(stageLabelName, i);
try {
final Length stageLabelX = src.getStageLabelX(i);
dest.setStageLabelX(stageLabelX, i);
}
catch (NullPointerException e) { }
try {
final Length stageLabelY = src.getStageLabelY(i);
dest.setStageLabelY(stageLabelY, i);
}
catch (NullPointerException e) { }
try {
final Length stageLabelZ = src.getStageLabelZ(i);
dest.setStageLabelZ(stageLabelZ, i);
}
catch (NullPointerException e) { }
}
}
catch (NullPointerException e) { }
try {
String pixelsID = src.getPixelsID(i);
dest.setPixelsID(pixelsID, i);
try {
DimensionOrder order = src.getPixelsDimensionOrder(i);
dest.setPixelsDimensionOrder(order, i);
}
catch (NullPointerException e) { }
try {
Length physicalSizeX = src.getPixelsPhysicalSizeX(i);
dest.setPixelsPhysicalSizeX(physicalSizeX, i);
}
catch (NullPointerException e) { }
try {
Length physicalSizeY = src.getPixelsPhysicalSizeY(i);
dest.setPixelsPhysicalSizeY(physicalSizeY, i);
}
catch (NullPointerException e) { }
try {
Length physicalSizeZ = src.getPixelsPhysicalSizeZ(i);
dest.setPixelsPhysicalSizeZ(physicalSizeZ, i);
}
catch (NullPointerException e) { }
try {
PositiveInteger sizeC = src.getPixelsSizeC(i);
dest.setPixelsSizeC(sizeC, i);
}
catch (NullPointerException e) { }
try {
PositiveInteger sizeT = src.getPixelsSizeT(i);
dest.setPixelsSizeT(sizeT, i);
}
catch (NullPointerException e) { }
try {
PositiveInteger sizeX = src.getPixelsSizeX(i);
dest.setPixelsSizeX(sizeX, i);
}
catch (NullPointerException e) { }
try {
PositiveInteger sizeY = src.getPixelsSizeY(i);
dest.setPixelsSizeY(sizeY, i);
}
catch (NullPointerException e) { }
try {
PositiveInteger sizeZ = src.getPixelsSizeZ(i);
dest.setPixelsSizeZ(sizeZ, i);
}
catch (NullPointerException e) { }
try {
Time timeIncrement = src.getPixelsTimeIncrement(i);
dest.setPixelsTimeIncrement(timeIncrement, i);
}
catch (NullPointerException e) { }
try {
PixelType type = src.getPixelsType(i);
dest.setPixelsType(type, i);
}
catch (NullPointerException e) { }
try {
Boolean bigEndian = src.getPixelsBigEndian(i);
dest.setPixelsBigEndian(bigEndian, i);
}
catch (NullPointerException e) { }
try {
Boolean interleaved = src.getPixelsInterleaved(i);
dest.setPixelsInterleaved(interleaved, i);
}
catch (NullPointerException e) { }
try {
PositiveInteger significantBits = src.getPixelsSignificantBits(i);
dest.setPixelsSignificantBits(significantBits, i);
}
catch (NullPointerException e) { }
int binDataCount = 0;
try {
binDataCount = src.getPixelsBinDataCount(i);
}
catch (NullPointerException e) { }
for (int q=0; q convertInstruments(MetadataRetrieve src,
MetadataStore dest)
{
List lightSourceIds = new ArrayList();
int instrumentCount = 0;
try {
instrumentCount = src.getInstrumentCount();
}
catch (NullPointerException e) { }
for (int i=0; i value = src.getMapAnnotationValue(i);
dest.setMapAnnotationValue(value, i);
}
catch (NullPointerException e) { }
try {
String annotator = src.getMapAnnotationAnnotator(i);
dest.setMapAnnotationAnnotator(annotator, i);
}
catch (NullPointerException e) { }
int annotationRefCount = 0;
try {
annotationRefCount = src.getMapAnnotationAnnotationCount(i);
}
catch (NullPointerException e) { }
for (int a=0; a lightSourceIds)
{
int lightSourceCount = 0;
try {
lightSourceCount = src.getLightSourceCount(instrumentIndex);
}
catch (NullPointerException e) { }
for (int lightSource=0; lightSource 0) {
lightSourceIds.add(id);
dest.setArcID(id, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) {
continue;
}
try {
String lotNumber = src.getArcLotNumber(instrumentIndex, lightSource);
if (lotNumber != null) {
dest.setArcLotNumber(lotNumber, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
try {
String manufacturer =
src.getArcManufacturer(instrumentIndex, lightSource);
if (manufacturer != null) {
dest.setArcManufacturer(manufacturer, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
try {
String model = src.getArcModel(instrumentIndex, lightSource);
if (model != null) {
dest.setArcModel(model, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
try {
Power power = src.getArcPower(instrumentIndex, lightSource);
if (power != null) {
dest.setArcPower(power, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
try {
String serialNumber =
src.getArcSerialNumber(instrumentIndex, lightSource);
if (serialNumber != null) {
dest.setArcSerialNumber(serialNumber, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
try {
ArcType arcType = src.getArcType(instrumentIndex, lightSource);
if (arcType != null) {
dest.setArcType(arcType, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
int lightSourceAnnotationRefCount = 0;
try {
lightSourceAnnotationRefCount = src.getLightSourceAnnotationRefCount(instrumentIndex, lightSource);
}
catch (NullPointerException e) { }
for (int r=0; r 0) {
lightSourceIds.add(id);
dest.setFilamentID(id, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) {
continue;
}
try {
String lotNumber =
src.getFilamentLotNumber(instrumentIndex, lightSource);
if (lotNumber != null) {
dest.setFilamentLotNumber(lotNumber, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
try {
String manufacturer =
src.getFilamentManufacturer(instrumentIndex, lightSource);
if (manufacturer != null) {
dest.setFilamentManufacturer(
manufacturer, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
try {
String model = src.getFilamentModel(instrumentIndex, lightSource);
if (model != null) {
dest.setFilamentModel(model, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
try {
Power power = src.getFilamentPower(instrumentIndex, lightSource);
if (power != null) {
dest.setFilamentPower(power, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
try {
String serialNumber =
src.getFilamentSerialNumber(instrumentIndex, lightSource);
if (serialNumber != null) {
dest.setFilamentSerialNumber(
serialNumber, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
try {
FilamentType filamentType =
src.getFilamentType(instrumentIndex, lightSource);
if (filamentType != null) {
dest.setFilamentType(filamentType, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
int lightSourceAnnotationRefCount = 0;
try {
lightSourceAnnotationRefCount = src.getLightSourceAnnotationRefCount(instrumentIndex, lightSource);
}
catch (NullPointerException e) { }
for (int r=0; r 0) {
lightSourceIds.add(id);
dest.setGenericExcitationSourceID(id, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) {
continue;
}
try {
List map =
src.getGenericExcitationSourceMap(instrumentIndex, lightSource);
dest.setGenericExcitationSourceMap(map, instrumentIndex, lightSource);
}
catch (NullPointerException e) { }
try {
String lotNumber = src.getGenericExcitationSourceLotNumber(
instrumentIndex, lightSource);
dest.setGenericExcitationSourceLotNumber(lotNumber,
instrumentIndex, lightSource);
}
catch (NullPointerException e) { }
try {
String manufacturer = src.getGenericExcitationSourceManufacturer(
instrumentIndex, lightSource);
dest.setGenericExcitationSourceManufacturer(manufacturer,
instrumentIndex, lightSource);
}
catch (NullPointerException e) { }
try {
String model =
src.getGenericExcitationSourceModel(instrumentIndex, lightSource);
dest.setGenericExcitationSourceModel(model,
instrumentIndex, lightSource);
}
catch (NullPointerException e) { }
try {
Power power =
src.getGenericExcitationSourcePower(instrumentIndex, lightSource);
dest.setGenericExcitationSourcePower(power,
instrumentIndex, lightSource);
}
catch (NullPointerException e) { }
try {
String serialNumber = src.getGenericExcitationSourceSerialNumber(
instrumentIndex, lightSource);
dest.setGenericExcitationSourceSerialNumber(serialNumber,
instrumentIndex, lightSource);
}
catch (NullPointerException e) { }
int lightSourceAnnotationRefCount = 0;
try {
lightSourceAnnotationRefCount = src.getLightSourceAnnotationRefCount(instrumentIndex, lightSource);
}
catch (NullPointerException e) { }
for (int r=0; r 0) {
lightSourceIds.add(id);
dest.setLaserID(id, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) {
continue;
}
try {
String lotNumber =
src.getLaserLotNumber(instrumentIndex, lightSource);
if (lotNumber != null) {
dest.setLaserLotNumber(lotNumber, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
try {
String manufacturer =
src.getLaserManufacturer(instrumentIndex, lightSource);
if (manufacturer != null) {
dest.setLaserManufacturer(
manufacturer, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
try {
String model = src.getLaserModel(instrumentIndex, lightSource);
if (model != null) {
dest.setLaserModel(model, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
try {
Power power = src.getLaserPower(instrumentIndex, lightSource);
if (power != null) {
dest.setLaserPower(power, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
try {
String serialNumber =
src.getLaserSerialNumber(instrumentIndex, lightSource);
if (serialNumber != null) {
dest.setLaserSerialNumber(
serialNumber, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
try {
LaserType laserType = src.getLaserType(instrumentIndex, lightSource);
if (laserType != null) {
dest.setLaserType(laserType, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
try {
PositiveInteger frequencyMultiplication =
src.getLaserFrequencyMultiplication(instrumentIndex, lightSource);
if (frequencyMultiplication != null) {
dest.setLaserFrequencyMultiplication(
frequencyMultiplication, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
try {
LaserMedium medium =
src.getLaserLaserMedium(instrumentIndex, lightSource);
if (medium != null) {
dest.setLaserLaserMedium(medium, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
try {
Boolean pockelCell =
src.getLaserPockelCell(instrumentIndex, lightSource);
if (pockelCell != null) {
dest.setLaserPockelCell(pockelCell, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
try {
Pulse pulse = src.getLaserPulse(instrumentIndex, lightSource);
if (pulse != null) {
dest.setLaserPulse(pulse, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
try {
String pump = src.getLaserPump(instrumentIndex, lightSource);
if (pump != null) {
dest.setLaserPump(pump, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
try {
Frequency repetitionRate =
src.getLaserRepetitionRate(instrumentIndex, lightSource);
if (repetitionRate != null) {
dest.setLaserRepetitionRate(
repetitionRate, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
try {
Boolean tuneable = src.getLaserTuneable(instrumentIndex, lightSource);
if (tuneable != null) {
dest.setLaserTuneable(tuneable, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
try {
Length wavelength =
src.getLaserWavelength(instrumentIndex, lightSource);
if (wavelength != null) {
dest.setLaserWavelength(wavelength, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
int lightSourceAnnotationRefCount = 0;
try {
lightSourceAnnotationRefCount = src.getLightSourceAnnotationRefCount(instrumentIndex, lightSource);
}
catch (NullPointerException e) { }
for (int r=0; r 0) {
lightSourceIds.add(id);
dest.setLightEmittingDiodeID(id, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) {
continue;
}
try {
String lotNumber =
src.getLightEmittingDiodeLotNumber(instrumentIndex, lightSource);
if (lotNumber != null) {
dest.setLightEmittingDiodeLotNumber(
lotNumber, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
try {
String manufacturer =
src.getLightEmittingDiodeManufacturer(instrumentIndex, lightSource);
if (manufacturer != null) {
dest.setLightEmittingDiodeManufacturer(
manufacturer, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
try {
String model =
src.getLightEmittingDiodeModel(instrumentIndex, lightSource);
if (model != null) {
dest.setLightEmittingDiodeModel(
model, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
try {
Power power =
src.getLightEmittingDiodePower(instrumentIndex, lightSource);
if (power != null) {
dest.setLightEmittingDiodePower(
power, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
try {
String serialNumber =
src.getLightEmittingDiodeSerialNumber(instrumentIndex, lightSource);
if (serialNumber != null) {
dest.setLightEmittingDiodeSerialNumber(
serialNumber, instrumentIndex, lightSource);
}
}
catch (NullPointerException e) { }
int lightSourceAnnotationRefCount = 0;
try {
lightSourceAnnotationRefCount = src.getLightSourceAnnotationRefCount(instrumentIndex, lightSource);
}
catch (NullPointerException e) { }
for (int r=0; r