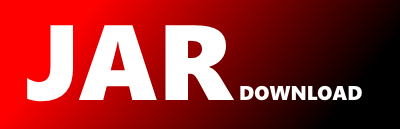
ome.xml.meta.MetadataRetrieve Maven / Gradle / Ivy
Show all versions of ome-xml Show documentation
/*
* #%L
* OME-XML Java library for working with OME-XML metadata structures.
* %%
* Copyright (C) 2006 - 2016 Open Microscopy Environment:
* - Massachusetts Institute of Technology
* - National Institutes of Health
* - University of Dundee
* - Board of Regents of the University of Wisconsin-Madison
* - Glencoe Software, Inc.
* %%
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDERS OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* The views and conclusions contained in the software and documentation are
* those of the authors and should not be interpreted as representing official
* policies, either expressed or implied, of any organization.
* #L%
*/
/*-----------------------------------------------------------------------------
*
* THIS IS AUTOMATICALLY GENERATED CODE. DO NOT MODIFY.
*
*-----------------------------------------------------------------------------
*/
package ome.xml.meta;
import java.util.List;
import ome.xml.model.*;
import ome.xml.model.enums.*;
import ome.xml.model.primitives.*;
import ome.units.quantity.Angle;
import ome.units.quantity.ElectricPotential;
import ome.units.quantity.Frequency;
import ome.units.quantity.Length;
import ome.units.quantity.Power;
import ome.units.quantity.Pressure;
import ome.units.quantity.Temperature;
import ome.units.quantity.Time;
import ome.units.unit.Unit;
/**
* A proxy whose responsibility it is to extract biological image data from a
* particular storage medium.
*
* The MetadataRetrieve
interface encompasses the metadata
* that any specific storage medium (file, relational database, etc.) should be
* expected to access from its backing data model.
*
*
The MetadataRetrieve
interface goes hand in hand with the
* MetadataStore
interface. Essentially,
* MetadataRetrieve
provides the "getter" methods for a storage
* medium, and MetadataStore
provides the "setter" methods.
*
*
Since it often makes sense for a storage medium to implement both
* interfaces, there is also an {@link IMetadata} interface encompassing
* both MetadataStore
and MetadataRetrieve
, which
* reduces the need to cast between object types.
*
*
See {@link ome.xml.meta.OMEXMLMetadata} for an example
* implementation.
*
* @author Melissa Linkert linkert at wisc.edu
* @author Curtis Rueden ctrueden at wisc.edu
*/
public interface MetadataRetrieve extends BaseMetadata {
// -- Entity counting (manual definitions) --
/**
* Get the number of links to a BooleanAnnotation.
*
* This method returns the number of references to the
* specified BooleanAnnotation held by all model objects.
*
* @param booleanAnnotationIndex the BooleanAnnotation index.
* @return the number of BooleanAnnotation links.
*/
int getBooleanAnnotationAnnotationCount(int booleanAnnotationIndex);
/**
* Get the number of links to a CommentAnnotation.
*
* This method returns the number of references to the
* specified CommentAnnotation held by all model objects.
*
* @param commentAnnotationIndex the CommentAnnotation index.
* @return the number of CommentAnnotation links.
*/
int getCommentAnnotationAnnotationCount(int commentAnnotationIndex);
/**
* Get the number of links to a DoubleAnnotation.
*
* This method returns the number of references to the
* specified DoubleAnnotation held by all model objects.
*
* @param doubleAnnotationIndex the DoubleAnnotation index.
* @return the number of DoubleAnnotation links.
*/
int getDoubleAnnotationAnnotationCount(int doubleAnnotationIndex);
/**
* Get the number of links to a FileAnnotation.
*
* This method returns the number of references to the
* specified FileAnnotation held by all model objects.
*
* @param fileAnnotationIndex the FileAnnotation index.
* @return the number of FileAnnotation links.
*/
int getFileAnnotationAnnotationCount(int fileAnnotationIndex);
/**
* Get the number of links to a ListAnnotation.
*
* This method returns the number of references to the
* specified ListAnnotation held by all model objects.
*
* @param listAnnotationIndex the ListAnnotation index.
* @return the number of ListAnnotation links.
*/
int getListAnnotationAnnotationCount(int listAnnotationIndex);
/**
* Get the number of links to a LongAnnotation.
*
* This method returns the number of references to the
* specified LongAnnotation held by all model objects.
*
* @param longAnnotationIndex the LongAnnotation index.
* @return the number of LongAnnotation links.
*/
int getLongAnnotationAnnotationCount(int longAnnotationIndex);
/**
* Get the number of links to a MapAnnotation.
*
* This method returns the number of references to the
* specified MapAnnotation held by all model objects.
*
* @param mapAnnotationIndex the MapAnnotation index.
* @return the number of MapAnnotation links.
*/
int getMapAnnotationAnnotationCount(int mapAnnotationIndex);
/**
* Get the number of links to a TagAnnotation.
*
* This method returns the number of references to the
* specified TagAnnotation held by all model objects.
*
* @param tagAnnotationIndex the TagAnnotation index.
* @return the number of TagAnnotation links.
*/
int getTagAnnotationAnnotationCount(int tagAnnotationIndex);
/**
* Get the number of links to a TermAnnotation.
*
* This method returns the number of references to the
* specified TermAnnotation held by all model objects.
*
* @param termAnnotationIndex the TermAnnotation index.
* @return the number of TermAnnotation links.
*/
int getTermAnnotationAnnotationCount(int termAnnotationIndex);
/**
* Get the number of links to a TimestampAnnotation.
*
* This method returns the number of references to the
* specified TimestampAnnotation held by all model objects.
*
* @param timestampAnnotationIndex the TimestampAnnotation index.
* @return the number of TimestampAnnotation links.
*/
int getTimestampAnnotationAnnotationCount(int timestampAnnotationIndex);
/**
* Get the number of links to an XMLAnnotation.
*
* This method returns the number of references to the
* specified XMLAnnotation held by all model objects.
*
* @param xmlAnnotationIndex the XMLAnnotation index.
* @return the number of XMLAnnotation links.
*/
int getXMLAnnotationAnnotationCount(int xmlAnnotationIndex);
/**
* Get the type of a LightSource.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the LightSource type.
*/
String getLightSourceType(int instrumentIndex, int lightSourceIndex);
/**
* Get the number of LightSource elements.
*
* @param instrumentIndex the Instrument index.
* @return the number of LightSource elements.
*/
int getLightSourceCount(int instrumentIndex);
/**
* Get the type of a Shape.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Shape type.
*/
String getShapeType(int ROIIndex, int shapeIndex);
/**
* Get the number of Shape elements.
*
* @param ROIIndex the ROI index.
* @return the number of Shape elements.
*/
int getShapeCount(int ROIIndex);
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the number of AnnotationRef elements in Channel.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the number of AnnotationRef elements.
*/
int getChannelAnnotationRefCount(int imageIndex, int channelIndex);
/**
* Get the number of AnnotationRef elements in Dataset.
*
* @param datasetIndex the Dataset index.
* @return the number of AnnotationRef elements.
*/
int getDatasetAnnotationRefCount(int datasetIndex);
/**
* Get the number of AnnotationRef elements in Detector.
*
* @param instrumentIndex the Instrument index.
* @param detectorIndex the Detector index.
* @return the number of AnnotationRef elements.
*/
int getDetectorAnnotationRefCount(int instrumentIndex, int detectorIndex);
/**
* Get the number of AnnotationRef elements in Dichroic.
*
* @param instrumentIndex the Instrument index.
* @param dichroicIndex the Dichroic index.
* @return the number of AnnotationRef elements.
*/
int getDichroicAnnotationRefCount(int instrumentIndex, int dichroicIndex);
/**
* Get the number of AnnotationRef elements in Experimenter.
*
* @param experimenterIndex the Experimenter index.
* @return the number of AnnotationRef elements.
*/
int getExperimenterAnnotationRefCount(int experimenterIndex);
/**
* Get the number of AnnotationRef elements in ExperimenterGroup.
*
* @param experimenterGroupIndex the ExperimenterGroup index.
* @return the number of AnnotationRef elements.
*/
int getExperimenterGroupAnnotationRefCount(int experimenterGroupIndex);
/**
* Get the number of AnnotationRef elements in Filter.
*
* @param instrumentIndex the Instrument index.
* @param filterIndex the Filter index.
* @return the number of AnnotationRef elements.
*/
int getFilterAnnotationRefCount(int instrumentIndex, int filterIndex);
/**
* Get the number of AnnotationRef elements in Folder.
*
* @param folderIndex the Folder index.
* @return the number of AnnotationRef elements.
*/
int getFolderAnnotationRefCount(int folderIndex);
/**
* Get the number of AnnotationRef elements in Image.
*
* @param imageIndex the Image index.
* @return the number of AnnotationRef elements.
*/
int getImageAnnotationRefCount(int imageIndex);
/**
* Get the number of AnnotationRef elements in Instrument.
*
* @param instrumentIndex the Instrument index.
* @return the number of AnnotationRef elements.
*/
int getInstrumentAnnotationRefCount(int instrumentIndex);
/**
* Get the number of AnnotationRef elements in LightPath.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the number of AnnotationRef elements.
*/
int getLightPathAnnotationRefCount(int imageIndex, int channelIndex);
/**
* Get the number of AnnotationRef elements in LightSource.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the number of AnnotationRef elements.
*/
int getLightSourceAnnotationRefCount(int instrumentIndex, int lightSourceIndex);
/**
* Get the number of AnnotationRef elements in Objective.
*
* @param instrumentIndex the Instrument index.
* @param objectiveIndex the Objective index.
* @return the number of AnnotationRef elements.
*/
int getObjectiveAnnotationRefCount(int instrumentIndex, int objectiveIndex);
/**
* Get the number of AnnotationRef elements in Plane.
*
* @param imageIndex the Image index.
* @param planeIndex the Plane index.
* @return the number of AnnotationRef elements.
*/
int getPlaneAnnotationRefCount(int imageIndex, int planeIndex);
/**
* Get the number of AnnotationRef elements in Plate.
*
* @param plateIndex the Plate index.
* @return the number of AnnotationRef elements.
*/
int getPlateAnnotationRefCount(int plateIndex);
/**
* Get the number of AnnotationRef elements in PlateAcquisition.
*
* @param plateIndex the Plate index.
* @param plateAcquisitionIndex the PlateAcquisition index.
* @return the number of AnnotationRef elements.
*/
int getPlateAcquisitionAnnotationRefCount(int plateIndex, int plateAcquisitionIndex);
/**
* Get the number of AnnotationRef elements in Project.
*
* @param projectIndex the Project index.
* @return the number of AnnotationRef elements.
*/
int getProjectAnnotationRefCount(int projectIndex);
/**
* Get the number of AnnotationRef elements in ROI.
*
* @param ROIIndex the ROI index.
* @return the number of AnnotationRef elements.
*/
int getROIAnnotationRefCount(int ROIIndex);
/**
* Get the number of AnnotationRef elements in Reagent.
*
* @param screenIndex the Screen index.
* @param reagentIndex the Reagent index.
* @return the number of AnnotationRef elements.
*/
int getReagentAnnotationRefCount(int screenIndex, int reagentIndex);
/**
* Get the number of AnnotationRef elements in Screen.
*
* @param screenIndex the Screen index.
* @return the number of AnnotationRef elements.
*/
int getScreenAnnotationRefCount(int screenIndex);
/**
* Get the number of AnnotationRef elements in Shape.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the number of AnnotationRef elements.
*/
int getShapeAnnotationRefCount(int ROIIndex, int shapeIndex);
/**
* Get the number of AnnotationRef elements in Well.
*
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
* @return the number of AnnotationRef elements.
*/
int getWellAnnotationRefCount(int plateIndex, int wellIndex);
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the number of BinData elements in Pixels.
*
* @param imageIndex the Image index.
* @return the number of BinData elements.
*/
int getPixelsBinDataCount(int imageIndex);
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the number of BooleanAnnotation elements.
*
* @return the number of BooleanAnnotation elements.
*/
int getBooleanAnnotationCount();
// -- Entity counting (code generated definitions) --
/**
* Get the number of Channel elements.
*
* @param imageIndex the Image index.
* @return the number of Channel elements.
*/
int getChannelCount(int imageIndex);
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the number of CommentAnnotation elements.
*
* @return the number of CommentAnnotation elements.
*/
int getCommentAnnotationCount();
// -- Entity counting (code generated definitions) --
/**
* Get the number of Dataset elements.
*
* @return the number of Dataset elements.
*/
int getDatasetCount();
// -- Entity counting (code generated definitions) --
/**
* Get the number of DatasetRef elements.
*
* @param projectIndex the Project index.
* @return the number of DatasetRef elements.
*/
int getDatasetRefCount(int projectIndex);
// -- Entity counting (code generated definitions) --
/**
* Get the number of Detector elements.
*
* @param instrumentIndex the Instrument index.
* @return the number of Detector elements.
*/
int getDetectorCount(int instrumentIndex);
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the number of Dichroic elements.
*
* @param instrumentIndex the Instrument index.
* @return the number of Dichroic elements.
*/
int getDichroicCount(int instrumentIndex);
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the number of DoubleAnnotation elements.
*
* @return the number of DoubleAnnotation elements.
*/
int getDoubleAnnotationCount();
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the number of EmissionFilterRef elements in FilterSet.
*
* @param instrumentIndex the Instrument index.
* @param filterSetIndex the FilterSet index.
* @return the number of EmissionFilterRef elements.
*/
int getFilterSetEmissionFilterRefCount(int instrumentIndex, int filterSetIndex);
/**
* Get the number of EmissionFilterRef elements in LightPath.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the number of EmissionFilterRef elements.
*/
int getLightPathEmissionFilterRefCount(int imageIndex, int channelIndex);
// -- Entity counting (code generated definitions) --
/**
* Get the number of ExcitationFilterRef elements in FilterSet.
*
* @param instrumentIndex the Instrument index.
* @param filterSetIndex the FilterSet index.
* @return the number of ExcitationFilterRef elements.
*/
int getFilterSetExcitationFilterRefCount(int instrumentIndex, int filterSetIndex);
/**
* Get the number of ExcitationFilterRef elements in LightPath.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the number of ExcitationFilterRef elements.
*/
int getLightPathExcitationFilterRefCount(int imageIndex, int channelIndex);
// -- Entity counting (code generated definitions) --
/**
* Get the number of Experiment elements.
*
* @return the number of Experiment elements.
*/
int getExperimentCount();
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the number of Experimenter elements.
*
* @return the number of Experimenter elements.
*/
int getExperimenterCount();
// -- Entity counting (code generated definitions) --
/**
* Get the number of ExperimenterGroup elements.
*
* @return the number of ExperimenterGroup elements.
*/
int getExperimenterGroupCount();
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the number of ExperimenterRef elements in ExperimenterGroup.
*
* @param experimenterGroupIndex the ExperimenterGroup index.
* @return the number of ExperimenterRef elements.
*/
int getExperimenterGroupExperimenterRefCount(int experimenterGroupIndex);
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the number of FileAnnotation elements.
*
* @return the number of FileAnnotation elements.
*/
int getFileAnnotationCount();
// -- Entity counting (code generated definitions) --
/**
* Get the number of Filter elements.
*
* @param instrumentIndex the Instrument index.
* @return the number of Filter elements.
*/
int getFilterCount(int instrumentIndex);
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the number of FilterSet elements.
*
* @param instrumentIndex the Instrument index.
* @return the number of FilterSet elements.
*/
int getFilterSetCount(int instrumentIndex);
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the number of Folder elements.
*
* @return the number of Folder elements.
*/
int getFolderCount();
// -- Entity counting (code generated definitions) --
/**
* Get the number of FolderRef elements.
*
* @param folderIndex the Folder index.
* @return the number of FolderRef elements.
*/
int getFolderRefCount(int folderIndex);
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the number of Image elements.
*
* @return the number of Image elements.
*/
int getImageCount();
// -- Entity counting (code generated definitions) --
/**
* Get the number of ImageRef elements in Dataset.
*
* @param datasetIndex the Dataset index.
* @return the number of ImageRef elements.
*/
int getDatasetImageRefCount(int datasetIndex);
/**
* Get the number of ImageRef elements in Folder.
*
* @param folderIndex the Folder index.
* @return the number of ImageRef elements.
*/
int getFolderImageRefCount(int folderIndex);
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the number of Instrument elements.
*
* @return the number of Instrument elements.
*/
int getInstrumentCount();
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the number of Leader elements.
*
* @param experimenterGroupIndex the ExperimenterGroup index.
* @return the number of Leader elements.
*/
int getLeaderCount(int experimenterGroupIndex);
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the number of LightSourceSettings elements in MicrobeamManipulation.
*
* @param experimentIndex the Experiment index.
* @param microbeamManipulationIndex the MicrobeamManipulation index.
* @return the number of LightSourceSettings elements.
*/
int getMicrobeamManipulationLightSourceSettingsCount(int experimentIndex, int microbeamManipulationIndex);
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the number of ListAnnotation elements.
*
* @return the number of ListAnnotation elements.
*/
int getListAnnotationCount();
// -- Entity counting (code generated definitions) --
/**
* Get the number of LongAnnotation elements.
*
* @return the number of LongAnnotation elements.
*/
int getLongAnnotationCount();
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the number of MapAnnotation elements.
*
* @return the number of MapAnnotation elements.
*/
int getMapAnnotationCount();
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the number of MicrobeamManipulation elements.
*
* @param experimentIndex the Experiment index.
* @return the number of MicrobeamManipulation elements.
*/
int getMicrobeamManipulationCount(int experimentIndex);
// -- Entity counting (code generated definitions) --
/**
* Get the number of MicrobeamManipulationRef elements.
*
* @param imageIndex the Image index.
* @return the number of MicrobeamManipulationRef elements.
*/
int getMicrobeamManipulationRefCount(int imageIndex);
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the number of Objective elements.
*
* @param instrumentIndex the Instrument index.
* @return the number of Objective elements.
*/
int getObjectiveCount(int instrumentIndex);
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the number of Plane elements.
*
* @param imageIndex the Image index.
* @return the number of Plane elements.
*/
int getPlaneCount(int imageIndex);
// -- Entity counting (code generated definitions) --
/**
* Get the number of Plate elements.
*
* @return the number of Plate elements.
*/
int getPlateCount();
// -- Entity counting (code generated definitions) --
/**
* Get the number of PlateAcquisition elements.
*
* @param plateIndex the Plate index.
* @return the number of PlateAcquisition elements.
*/
int getPlateAcquisitionCount(int plateIndex);
// -- Entity counting (code generated definitions) --
/**
* Get the number of PlateRef elements.
*
* @param screenIndex the Screen index.
* @return the number of PlateRef elements.
*/
int getPlateRefCount(int screenIndex);
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the number of Project elements.
*
* @return the number of Project elements.
*/
int getProjectCount();
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the number of ROI elements.
*
* @return the number of ROI elements.
*/
int getROICount();
// -- Entity counting (code generated definitions) --
/**
* Get the number of ROIRef elements in Folder.
*
* @param folderIndex the Folder index.
* @return the number of ROIRef elements.
*/
int getFolderROIRefCount(int folderIndex);
/**
* Get the number of ROIRef elements in Image.
*
* @param imageIndex the Image index.
* @return the number of ROIRef elements.
*/
int getImageROIRefCount(int imageIndex);
/**
* Get the number of ROIRef elements in MicrobeamManipulation.
*
* @param experimentIndex the Experiment index.
* @param microbeamManipulationIndex the MicrobeamManipulation index.
* @return the number of ROIRef elements.
*/
int getMicrobeamManipulationROIRefCount(int experimentIndex, int microbeamManipulationIndex);
// -- Entity counting (code generated definitions) --
/**
* Get the number of Reagent elements.
*
* @param screenIndex the Screen index.
* @return the number of Reagent elements.
*/
int getReagentCount(int screenIndex);
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the number of Screen elements.
*
* @return the number of Screen elements.
*/
int getScreenCount();
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the number of TagAnnotation elements.
*
* @return the number of TagAnnotation elements.
*/
int getTagAnnotationCount();
// -- Entity counting (code generated definitions) --
/**
* Get the number of TermAnnotation elements.
*
* @return the number of TermAnnotation elements.
*/
int getTermAnnotationCount();
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the number of TiffData elements.
*
* @param imageIndex the Image index.
* @return the number of TiffData elements.
*/
int getTiffDataCount(int imageIndex);
// -- Entity counting (code generated definitions) --
/**
* Get the number of TimestampAnnotation elements.
*
* @return the number of TimestampAnnotation elements.
*/
int getTimestampAnnotationCount();
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the text value of UUID.
*
* @param imageIndex the Image index.
* @param tiffDataIndex the TiffData index.
* @return the text value.
*/
String getUUIDValue(int imageIndex, int tiffDataIndex);
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
// -- Entity counting (code generated definitions) --
/**
* Get the number of Well elements.
*
* @param plateIndex the Plate index.
* @return the number of Well elements.
*/
int getWellCount(int plateIndex);
// -- Entity counting (code generated definitions) --
/**
* Get the number of WellSample elements.
*
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
* @return the number of WellSample elements.
*/
int getWellSampleCount(int plateIndex, int wellIndex);
// -- Entity counting (code generated definitions) --
/**
* Get the number of WellSampleRef elements.
*
* @param plateIndex the Plate index.
* @param plateAcquisitionIndex the PlateAcquisition index.
* @return the number of WellSampleRef elements.
*/
int getWellSampleRefCount(int plateIndex, int plateAcquisitionIndex);
// -- Entity counting (code generated definitions) --
/**
* Get the number of XMLAnnotation elements.
*
* @return the number of XMLAnnotation elements.
*/
int getXMLAnnotationCount();
// -- Entity retrieval (manual definitions) --
/**
* Get the values from a MapAnnotation.
*
* @param mapAnnotationIndex the MapAnnotation index.
* @return the MapAnnotation values.
*/
List getMapAnnotationValue(int mapAnnotationIndex);
/**
* Get the MapAnnotation values from a GenericExcitationSource.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the MapAnnotation values.
*/
List getGenericExcitationSourceMap(int instrumentIndex, int lightSourceIndex);
/**
* Get the MapAnnotation values from a ImagingEnvironment.
*
* @param imageIndex the Image index.
* @return the MapAnnotation values.
*/
List getImagingEnvironmentMap(int imageIndex);
/**
* Get the UUID associated with this collection of metadata.
*
* @return the UUID.
*/
/** Gets the UUID associated with this collection of metadata. */
String getUUID();
// -- Entity retrieval (code generated definitions) --
/**
* Get the AnnotationRef property of Arc.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getArcAnnotationRef(int instrumentIndex, int lightSourceIndex, int annotationRefIndex);
/**
* Get the ID property of Arc.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the ID property.
*/
String getArcID(int instrumentIndex, int lightSourceIndex);
/**
* Get the LotNumber property of Arc.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the LotNumber property.
*/
String getArcLotNumber(int instrumentIndex, int lightSourceIndex);
/**
* Get the Manufacturer property of Arc.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the Manufacturer property.
*/
String getArcManufacturer(int instrumentIndex, int lightSourceIndex);
/**
* Get the Model property of Arc.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the Model property.
*/
String getArcModel(int instrumentIndex, int lightSourceIndex);
/**
* Get the Power property of Arc.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the Power property.
*/
Power getArcPower(int instrumentIndex, int lightSourceIndex);
/**
* Get the SerialNumber property of Arc.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the SerialNumber property.
*/
String getArcSerialNumber(int instrumentIndex, int lightSourceIndex);
/**
* Get the Type property of Arc.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the Type property.
*/
ArcType getArcType(int instrumentIndex, int lightSourceIndex);
/**
* Get the Base64Binary property of BinData.
*
* @param fileAnnotationIndex the FileAnnotation index.
* @return the Base64Binary property.
*/
byte[] getBinaryFileBinData(int fileAnnotationIndex);
/**
* Get the Base64Binary property of BinData.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Base64Binary property.
*/
byte[] getMaskBinData(int ROIIndex, int shapeIndex);
/**
* Get the Base64Binary property of BinData.
*
* @param imageIndex the Image index.
* @param binDataIndex the BinData index.
* @return the Base64Binary property.
*/
byte[] getPixelsBinData(int imageIndex, int binDataIndex);
/**
* Get the BigEndian property of BinData.
*
* @param fileAnnotationIndex the FileAnnotation index.
* @return the BigEndian property.
*/
Boolean getBinaryFileBinDataBigEndian(int fileAnnotationIndex);
/**
* Get the BigEndian property of BinData.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the BigEndian property.
*/
Boolean getMaskBinDataBigEndian(int ROIIndex, int shapeIndex);
/**
* Get the BigEndian property of BinData.
*
* @param imageIndex the Image index.
* @param binDataIndex the BinData index.
* @return the BigEndian property.
*/
Boolean getPixelsBinDataBigEndian(int imageIndex, int binDataIndex);
/**
* Get the Compression property of BinData.
*
* @param fileAnnotationIndex the FileAnnotation index.
* @return the Compression property.
*/
Compression getBinaryFileBinDataCompression(int fileAnnotationIndex);
/**
* Get the Compression property of BinData.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Compression property.
*/
Compression getMaskBinDataCompression(int ROIIndex, int shapeIndex);
/**
* Get the Compression property of BinData.
*
* @param imageIndex the Image index.
* @param binDataIndex the BinData index.
* @return the Compression property.
*/
Compression getPixelsBinDataCompression(int imageIndex, int binDataIndex);
/**
* Get the Length property of BinData.
*
* @param fileAnnotationIndex the FileAnnotation index.
* @return the Length property.
*/
NonNegativeLong getBinaryFileBinDataLength(int fileAnnotationIndex);
/**
* Get the Length property of BinData.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Length property.
*/
NonNegativeLong getMaskBinDataLength(int ROIIndex, int shapeIndex);
/**
* Get the Length property of BinData.
*
* @param imageIndex the Image index.
* @param binDataIndex the BinData index.
* @return the Length property.
*/
NonNegativeLong getPixelsBinDataLength(int imageIndex, int binDataIndex);
/**
* Get the FileName property of BinaryFile.
*
* @param fileAnnotationIndex the FileAnnotation index.
* @return the FileName property.
*/
String getBinaryFileFileName(int fileAnnotationIndex);
/**
* Get the MIMEType property of BinaryFile.
*
* @param fileAnnotationIndex the FileAnnotation index.
* @return the MIMEType property.
*/
String getBinaryFileMIMEType(int fileAnnotationIndex);
/**
* Get the Size property of BinaryFile.
*
* @param fileAnnotationIndex the FileAnnotation index.
* @return the Size property.
*/
NonNegativeLong getBinaryFileSize(int fileAnnotationIndex);
/**
* Get the MetadataFile property of BinaryOnly.
*
* @return the MetadataFile property.
*/
String getBinaryOnlyMetadataFile();
/**
* Get the UUID property of BinaryOnly.
*
* @return the UUID property.
*/
String getBinaryOnlyUUID();
/**
* Get the AnnotationRef property of BooleanAnnotation.
*
* @param booleanAnnotationIndex the BooleanAnnotation index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getBooleanAnnotationAnnotationRef(int booleanAnnotationIndex, int annotationRefIndex);
/**
* Get the Annotator property of BooleanAnnotation.
*
* @param booleanAnnotationIndex the BooleanAnnotation index.
* @return the Annotator property.
*/
String getBooleanAnnotationAnnotator(int booleanAnnotationIndex);
/**
* Get the Description property of BooleanAnnotation.
*
* @param booleanAnnotationIndex the BooleanAnnotation index.
* @return the Description property.
*/
String getBooleanAnnotationDescription(int booleanAnnotationIndex);
/**
* Get the ID property of BooleanAnnotation.
*
* @param booleanAnnotationIndex the BooleanAnnotation index.
* @return the ID property.
*/
String getBooleanAnnotationID(int booleanAnnotationIndex);
/**
* Get the Namespace property of BooleanAnnotation.
*
* @param booleanAnnotationIndex the BooleanAnnotation index.
* @return the Namespace property.
*/
String getBooleanAnnotationNamespace(int booleanAnnotationIndex);
/**
* Get the Value property of BooleanAnnotation.
*
* @param booleanAnnotationIndex the BooleanAnnotation index.
* @return the Value property.
*/
Boolean getBooleanAnnotationValue(int booleanAnnotationIndex);
/**
* Get the AcquisitionMode property of Channel.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the AcquisitionMode property.
*/
AcquisitionMode getChannelAcquisitionMode(int imageIndex, int channelIndex);
/**
* Get the AnnotationRef property of Channel.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getChannelAnnotationRef(int imageIndex, int channelIndex, int annotationRefIndex);
/**
* Get the Color property of Channel.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the Color property.
*/
Color getChannelColor(int imageIndex, int channelIndex);
/**
* Get the ContrastMethod property of Channel.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the ContrastMethod property.
*/
ContrastMethod getChannelContrastMethod(int imageIndex, int channelIndex);
/**
* Get the EmissionWavelength property of Channel.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the EmissionWavelength property.
*/
Length getChannelEmissionWavelength(int imageIndex, int channelIndex);
/**
* Get the ExcitationWavelength property of Channel.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the ExcitationWavelength property.
*/
Length getChannelExcitationWavelength(int imageIndex, int channelIndex);
/**
* Get the FilterSetRef property of Channel.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the FilterSetRef property.
*/
String getChannelFilterSetRef(int imageIndex, int channelIndex);
/**
* Get the Fluor property of Channel.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the Fluor property.
*/
String getChannelFluor(int imageIndex, int channelIndex);
/**
* Get the ID property of Channel.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the ID property.
*/
String getChannelID(int imageIndex, int channelIndex);
/**
* Get the IlluminationType property of Channel.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the IlluminationType property.
*/
IlluminationType getChannelIlluminationType(int imageIndex, int channelIndex);
/**
* Get the NDFilter property of Channel.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the NDFilter property.
*/
Double getChannelNDFilter(int imageIndex, int channelIndex);
/**
* Get the Name property of Channel.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the Name property.
*/
String getChannelName(int imageIndex, int channelIndex);
/**
* Get the PinholeSize property of Channel.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the PinholeSize property.
*/
Length getChannelPinholeSize(int imageIndex, int channelIndex);
/**
* Get the PockelCellSetting property of Channel.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the PockelCellSetting property.
*/
Integer getChannelPockelCellSetting(int imageIndex, int channelIndex);
/**
* Get the SamplesPerPixel property of Channel.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the SamplesPerPixel property.
*/
PositiveInteger getChannelSamplesPerPixel(int imageIndex, int channelIndex);
/**
* Get the AnnotationRef property of CommentAnnotation.
*
* @param commentAnnotationIndex the CommentAnnotation index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getCommentAnnotationAnnotationRef(int commentAnnotationIndex, int annotationRefIndex);
/**
* Get the Annotator property of CommentAnnotation.
*
* @param commentAnnotationIndex the CommentAnnotation index.
* @return the Annotator property.
*/
String getCommentAnnotationAnnotator(int commentAnnotationIndex);
/**
* Get the Description property of CommentAnnotation.
*
* @param commentAnnotationIndex the CommentAnnotation index.
* @return the Description property.
*/
String getCommentAnnotationDescription(int commentAnnotationIndex);
/**
* Get the ID property of CommentAnnotation.
*
* @param commentAnnotationIndex the CommentAnnotation index.
* @return the ID property.
*/
String getCommentAnnotationID(int commentAnnotationIndex);
/**
* Get the Namespace property of CommentAnnotation.
*
* @param commentAnnotationIndex the CommentAnnotation index.
* @return the Namespace property.
*/
String getCommentAnnotationNamespace(int commentAnnotationIndex);
/**
* Get the Value property of CommentAnnotation.
*
* @param commentAnnotationIndex the CommentAnnotation index.
* @return the Value property.
*/
String getCommentAnnotationValue(int commentAnnotationIndex);
/**
* Get the AnnotationRef property of Dataset.
*
* @param datasetIndex the Dataset index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getDatasetAnnotationRef(int datasetIndex, int annotationRefIndex);
/**
* Get the Description property of Dataset.
*
* @param datasetIndex the Dataset index.
* @return the Description property.
*/
String getDatasetDescription(int datasetIndex);
/**
* Get the ExperimenterGroupRef property of Dataset.
*
* @param datasetIndex the Dataset index.
* @return the ExperimenterGroupRef property.
*/
String getDatasetExperimenterGroupRef(int datasetIndex);
/**
* Get the ExperimenterRef property of Dataset.
*
* @param datasetIndex the Dataset index.
* @return the ExperimenterRef property.
*/
String getDatasetExperimenterRef(int datasetIndex);
/**
* Get the ID property of Dataset.
*
* @param datasetIndex the Dataset index.
* @return the ID property.
*/
String getDatasetID(int datasetIndex);
/**
* Get the ImageRef property of Dataset.
*
* @param datasetIndex the Dataset index.
* @param imageRefIndex ImageRef index.
* @return the ImageRef property.
*/
String getDatasetImageRef(int datasetIndex, int imageRefIndex);
/**
* Get the Name property of Dataset.
*
* @param datasetIndex the Dataset index.
* @return the Name property.
*/
String getDatasetName(int datasetIndex);
/**
* Get the AmplificationGain property of Detector.
*
* @param instrumentIndex the Instrument index.
* @param detectorIndex the Detector index.
* @return the AmplificationGain property.
*/
Double getDetectorAmplificationGain(int instrumentIndex, int detectorIndex);
/**
* Get the AnnotationRef property of Detector.
*
* @param instrumentIndex the Instrument index.
* @param detectorIndex the Detector index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getDetectorAnnotationRef(int instrumentIndex, int detectorIndex, int annotationRefIndex);
/**
* Get the Gain property of Detector.
*
* @param instrumentIndex the Instrument index.
* @param detectorIndex the Detector index.
* @return the Gain property.
*/
Double getDetectorGain(int instrumentIndex, int detectorIndex);
/**
* Get the ID property of Detector.
*
* @param instrumentIndex the Instrument index.
* @param detectorIndex the Detector index.
* @return the ID property.
*/
String getDetectorID(int instrumentIndex, int detectorIndex);
/**
* Get the LotNumber property of Detector.
*
* @param instrumentIndex the Instrument index.
* @param detectorIndex the Detector index.
* @return the LotNumber property.
*/
String getDetectorLotNumber(int instrumentIndex, int detectorIndex);
/**
* Get the Manufacturer property of Detector.
*
* @param instrumentIndex the Instrument index.
* @param detectorIndex the Detector index.
* @return the Manufacturer property.
*/
String getDetectorManufacturer(int instrumentIndex, int detectorIndex);
/**
* Get the Model property of Detector.
*
* @param instrumentIndex the Instrument index.
* @param detectorIndex the Detector index.
* @return the Model property.
*/
String getDetectorModel(int instrumentIndex, int detectorIndex);
/**
* Get the Offset property of Detector.
*
* @param instrumentIndex the Instrument index.
* @param detectorIndex the Detector index.
* @return the Offset property.
*/
Double getDetectorOffset(int instrumentIndex, int detectorIndex);
/**
* Get the SerialNumber property of Detector.
*
* @param instrumentIndex the Instrument index.
* @param detectorIndex the Detector index.
* @return the SerialNumber property.
*/
String getDetectorSerialNumber(int instrumentIndex, int detectorIndex);
/**
* Get the Type property of Detector.
*
* @param instrumentIndex the Instrument index.
* @param detectorIndex the Detector index.
* @return the Type property.
*/
DetectorType getDetectorType(int instrumentIndex, int detectorIndex);
/**
* Get the Voltage property of Detector.
*
* @param instrumentIndex the Instrument index.
* @param detectorIndex the Detector index.
* @return the Voltage property.
*/
ElectricPotential getDetectorVoltage(int instrumentIndex, int detectorIndex);
/**
* Get the Zoom property of Detector.
*
* @param instrumentIndex the Instrument index.
* @param detectorIndex the Detector index.
* @return the Zoom property.
*/
Double getDetectorZoom(int instrumentIndex, int detectorIndex);
/**
* Get the Binning property of DetectorSettings.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the Binning property.
*/
Binning getDetectorSettingsBinning(int imageIndex, int channelIndex);
/**
* Get the Gain property of DetectorSettings.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the Gain property.
*/
Double getDetectorSettingsGain(int imageIndex, int channelIndex);
/**
* Get the ID property of DetectorSettings.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the ID property.
*/
String getDetectorSettingsID(int imageIndex, int channelIndex);
/**
* Get the Integration property of DetectorSettings.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the Integration property.
*/
PositiveInteger getDetectorSettingsIntegration(int imageIndex, int channelIndex);
/**
* Get the Offset property of DetectorSettings.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the Offset property.
*/
Double getDetectorSettingsOffset(int imageIndex, int channelIndex);
/**
* Get the ReadOutRate property of DetectorSettings.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the ReadOutRate property.
*/
Frequency getDetectorSettingsReadOutRate(int imageIndex, int channelIndex);
/**
* Get the Voltage property of DetectorSettings.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the Voltage property.
*/
ElectricPotential getDetectorSettingsVoltage(int imageIndex, int channelIndex);
/**
* Get the Zoom property of DetectorSettings.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the Zoom property.
*/
Double getDetectorSettingsZoom(int imageIndex, int channelIndex);
/**
* Get the AnnotationRef property of Dichroic.
*
* @param instrumentIndex the Instrument index.
* @param dichroicIndex the Dichroic index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getDichroicAnnotationRef(int instrumentIndex, int dichroicIndex, int annotationRefIndex);
/**
* Get the ID property of Dichroic.
*
* @param instrumentIndex the Instrument index.
* @param dichroicIndex the Dichroic index.
* @return the ID property.
*/
String getDichroicID(int instrumentIndex, int dichroicIndex);
/**
* Get the LotNumber property of Dichroic.
*
* @param instrumentIndex the Instrument index.
* @param dichroicIndex the Dichroic index.
* @return the LotNumber property.
*/
String getDichroicLotNumber(int instrumentIndex, int dichroicIndex);
/**
* Get the Manufacturer property of Dichroic.
*
* @param instrumentIndex the Instrument index.
* @param dichroicIndex the Dichroic index.
* @return the Manufacturer property.
*/
String getDichroicManufacturer(int instrumentIndex, int dichroicIndex);
/**
* Get the Model property of Dichroic.
*
* @param instrumentIndex the Instrument index.
* @param dichroicIndex the Dichroic index.
* @return the Model property.
*/
String getDichroicModel(int instrumentIndex, int dichroicIndex);
/**
* Get the SerialNumber property of Dichroic.
*
* @param instrumentIndex the Instrument index.
* @param dichroicIndex the Dichroic index.
* @return the SerialNumber property.
*/
String getDichroicSerialNumber(int instrumentIndex, int dichroicIndex);
/**
* Get the AnnotationRef property of DoubleAnnotation.
*
* @param doubleAnnotationIndex the DoubleAnnotation index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getDoubleAnnotationAnnotationRef(int doubleAnnotationIndex, int annotationRefIndex);
/**
* Get the Annotator property of DoubleAnnotation.
*
* @param doubleAnnotationIndex the DoubleAnnotation index.
* @return the Annotator property.
*/
String getDoubleAnnotationAnnotator(int doubleAnnotationIndex);
/**
* Get the Description property of DoubleAnnotation.
*
* @param doubleAnnotationIndex the DoubleAnnotation index.
* @return the Description property.
*/
String getDoubleAnnotationDescription(int doubleAnnotationIndex);
/**
* Get the ID property of DoubleAnnotation.
*
* @param doubleAnnotationIndex the DoubleAnnotation index.
* @return the ID property.
*/
String getDoubleAnnotationID(int doubleAnnotationIndex);
/**
* Get the Namespace property of DoubleAnnotation.
*
* @param doubleAnnotationIndex the DoubleAnnotation index.
* @return the Namespace property.
*/
String getDoubleAnnotationNamespace(int doubleAnnotationIndex);
/**
* Get the Value property of DoubleAnnotation.
*
* @param doubleAnnotationIndex the DoubleAnnotation index.
* @return the Value property.
*/
Double getDoubleAnnotationValue(int doubleAnnotationIndex);
/**
* Get the AnnotationRef property of Ellipse.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getEllipseAnnotationRef(int ROIIndex, int shapeIndex, int annotationRefIndex);
/**
* Get the FillColor property of Ellipse.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FillColor property.
*/
Color getEllipseFillColor(int ROIIndex, int shapeIndex);
/**
* Get the FillRule property of Ellipse.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FillRule property.
*/
FillRule getEllipseFillRule(int ROIIndex, int shapeIndex);
/**
* Get the FontFamily property of Ellipse.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FontFamily property.
*/
FontFamily getEllipseFontFamily(int ROIIndex, int shapeIndex);
/**
* Get the FontSize property of Ellipse.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FontSize property.
*/
Length getEllipseFontSize(int ROIIndex, int shapeIndex);
/**
* Get the FontStyle property of Ellipse.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FontStyle property.
*/
FontStyle getEllipseFontStyle(int ROIIndex, int shapeIndex);
/**
* Get the ID property of Ellipse.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the ID property.
*/
String getEllipseID(int ROIIndex, int shapeIndex);
/**
* Get the Locked property of Ellipse.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Locked property.
*/
Boolean getEllipseLocked(int ROIIndex, int shapeIndex);
/**
* Get the RadiusX property of Ellipse.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the RadiusX property.
*/
Double getEllipseRadiusX(int ROIIndex, int shapeIndex);
/**
* Get the RadiusY property of Ellipse.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the RadiusY property.
*/
Double getEllipseRadiusY(int ROIIndex, int shapeIndex);
/**
* Get the StrokeColor property of Ellipse.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the StrokeColor property.
*/
Color getEllipseStrokeColor(int ROIIndex, int shapeIndex);
/**
* Get the StrokeDashArray property of Ellipse.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the StrokeDashArray property.
*/
String getEllipseStrokeDashArray(int ROIIndex, int shapeIndex);
/**
* Get the StrokeWidth property of Ellipse.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the StrokeWidth property.
*/
Length getEllipseStrokeWidth(int ROIIndex, int shapeIndex);
/**
* Get the Text property of Ellipse.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Text property.
*/
String getEllipseText(int ROIIndex, int shapeIndex);
/**
* Get the TheC property of Ellipse.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the TheC property.
*/
NonNegativeInteger getEllipseTheC(int ROIIndex, int shapeIndex);
/**
* Get the TheT property of Ellipse.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the TheT property.
*/
NonNegativeInteger getEllipseTheT(int ROIIndex, int shapeIndex);
/**
* Get the TheZ property of Ellipse.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the TheZ property.
*/
NonNegativeInteger getEllipseTheZ(int ROIIndex, int shapeIndex);
/**
* Get the Transform property of Ellipse.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Transform property.
*/
AffineTransform getEllipseTransform(int ROIIndex, int shapeIndex);
/**
* Get the X property of Ellipse.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the X property.
*/
Double getEllipseX(int ROIIndex, int shapeIndex);
/**
* Get the Y property of Ellipse.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Y property.
*/
Double getEllipseY(int ROIIndex, int shapeIndex);
/**
* Get the Description property of Experiment.
*
* @param experimentIndex the Experiment index.
* @return the Description property.
*/
String getExperimentDescription(int experimentIndex);
/**
* Get the ExperimenterRef property of Experiment.
*
* @param experimentIndex the Experiment index.
* @return the ExperimenterRef property.
*/
String getExperimentExperimenterRef(int experimentIndex);
/**
* Get the ID property of Experiment.
*
* @param experimentIndex the Experiment index.
* @return the ID property.
*/
String getExperimentID(int experimentIndex);
/**
* Get the Type property of Experiment.
*
* @param experimentIndex the Experiment index.
* @return the Type property.
*/
ExperimentType getExperimentType(int experimentIndex);
/**
* Get the AnnotationRef property of Experimenter.
*
* @param experimenterIndex the Experimenter index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getExperimenterAnnotationRef(int experimenterIndex, int annotationRefIndex);
/**
* Get the Email property of Experimenter.
*
* @param experimenterIndex the Experimenter index.
* @return the Email property.
*/
String getExperimenterEmail(int experimenterIndex);
/**
* Get the FirstName property of Experimenter.
*
* @param experimenterIndex the Experimenter index.
* @return the FirstName property.
*/
String getExperimenterFirstName(int experimenterIndex);
/**
* Get the ID property of Experimenter.
*
* @param experimenterIndex the Experimenter index.
* @return the ID property.
*/
String getExperimenterID(int experimenterIndex);
/**
* Get the Institution property of Experimenter.
*
* @param experimenterIndex the Experimenter index.
* @return the Institution property.
*/
String getExperimenterInstitution(int experimenterIndex);
/**
* Get the LastName property of Experimenter.
*
* @param experimenterIndex the Experimenter index.
* @return the LastName property.
*/
String getExperimenterLastName(int experimenterIndex);
/**
* Get the MiddleName property of Experimenter.
*
* @param experimenterIndex the Experimenter index.
* @return the MiddleName property.
*/
String getExperimenterMiddleName(int experimenterIndex);
/**
* Get the UserName property of Experimenter.
*
* @param experimenterIndex the Experimenter index.
* @return the UserName property.
*/
String getExperimenterUserName(int experimenterIndex);
/**
* Get the AnnotationRef property of ExperimenterGroup.
*
* @param experimenterGroupIndex the ExperimenterGroup index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getExperimenterGroupAnnotationRef(int experimenterGroupIndex, int annotationRefIndex);
/**
* Get the Description property of ExperimenterGroup.
*
* @param experimenterGroupIndex the ExperimenterGroup index.
* @return the Description property.
*/
String getExperimenterGroupDescription(int experimenterGroupIndex);
/**
* Get the ExperimenterRef property of ExperimenterGroup.
*
* @param experimenterGroupIndex the ExperimenterGroup index.
* @param experimenterRefIndex ExperimenterRef index.
* @return the ExperimenterRef property.
*/
String getExperimenterGroupExperimenterRef(int experimenterGroupIndex, int experimenterRefIndex);
/**
* Get the ID property of ExperimenterGroup.
*
* @param experimenterGroupIndex the ExperimenterGroup index.
* @return the ID property.
*/
String getExperimenterGroupID(int experimenterGroupIndex);
/**
* Get the Leader property of ExperimenterGroup.
*
* @param experimenterGroupIndex the ExperimenterGroup index.
* @param leaderIndex Leader index.
* @return the Leader property.
*/
String getExperimenterGroupLeader(int experimenterGroupIndex, int leaderIndex);
/**
* Get the Name property of ExperimenterGroup.
*
* @param experimenterGroupIndex the ExperimenterGroup index.
* @return the Name property.
*/
String getExperimenterGroupName(int experimenterGroupIndex);
/**
* Get the AnnotationRef property of Filament.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getFilamentAnnotationRef(int instrumentIndex, int lightSourceIndex, int annotationRefIndex);
/**
* Get the ID property of Filament.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the ID property.
*/
String getFilamentID(int instrumentIndex, int lightSourceIndex);
/**
* Get the LotNumber property of Filament.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the LotNumber property.
*/
String getFilamentLotNumber(int instrumentIndex, int lightSourceIndex);
/**
* Get the Manufacturer property of Filament.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the Manufacturer property.
*/
String getFilamentManufacturer(int instrumentIndex, int lightSourceIndex);
/**
* Get the Model property of Filament.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the Model property.
*/
String getFilamentModel(int instrumentIndex, int lightSourceIndex);
/**
* Get the Power property of Filament.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the Power property.
*/
Power getFilamentPower(int instrumentIndex, int lightSourceIndex);
/**
* Get the SerialNumber property of Filament.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the SerialNumber property.
*/
String getFilamentSerialNumber(int instrumentIndex, int lightSourceIndex);
/**
* Get the Type property of Filament.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the Type property.
*/
FilamentType getFilamentType(int instrumentIndex, int lightSourceIndex);
/**
* Get the AnnotationRef property of FileAnnotation.
*
* @param fileAnnotationIndex the FileAnnotation index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getFileAnnotationAnnotationRef(int fileAnnotationIndex, int annotationRefIndex);
/**
* Get the Annotator property of FileAnnotation.
*
* @param fileAnnotationIndex the FileAnnotation index.
* @return the Annotator property.
*/
String getFileAnnotationAnnotator(int fileAnnotationIndex);
/**
* Get the Description property of FileAnnotation.
*
* @param fileAnnotationIndex the FileAnnotation index.
* @return the Description property.
*/
String getFileAnnotationDescription(int fileAnnotationIndex);
/**
* Get the ID property of FileAnnotation.
*
* @param fileAnnotationIndex the FileAnnotation index.
* @return the ID property.
*/
String getFileAnnotationID(int fileAnnotationIndex);
/**
* Get the Namespace property of FileAnnotation.
*
* @param fileAnnotationIndex the FileAnnotation index.
* @return the Namespace property.
*/
String getFileAnnotationNamespace(int fileAnnotationIndex);
/**
* Get the AnnotationRef property of Filter.
*
* @param instrumentIndex the Instrument index.
* @param filterIndex the Filter index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getFilterAnnotationRef(int instrumentIndex, int filterIndex, int annotationRefIndex);
/**
* Get the FilterWheel property of Filter.
*
* @param instrumentIndex the Instrument index.
* @param filterIndex the Filter index.
* @return the FilterWheel property.
*/
String getFilterFilterWheel(int instrumentIndex, int filterIndex);
/**
* Get the ID property of Filter.
*
* @param instrumentIndex the Instrument index.
* @param filterIndex the Filter index.
* @return the ID property.
*/
String getFilterID(int instrumentIndex, int filterIndex);
/**
* Get the LotNumber property of Filter.
*
* @param instrumentIndex the Instrument index.
* @param filterIndex the Filter index.
* @return the LotNumber property.
*/
String getFilterLotNumber(int instrumentIndex, int filterIndex);
/**
* Get the Manufacturer property of Filter.
*
* @param instrumentIndex the Instrument index.
* @param filterIndex the Filter index.
* @return the Manufacturer property.
*/
String getFilterManufacturer(int instrumentIndex, int filterIndex);
/**
* Get the Model property of Filter.
*
* @param instrumentIndex the Instrument index.
* @param filterIndex the Filter index.
* @return the Model property.
*/
String getFilterModel(int instrumentIndex, int filterIndex);
/**
* Get the SerialNumber property of Filter.
*
* @param instrumentIndex the Instrument index.
* @param filterIndex the Filter index.
* @return the SerialNumber property.
*/
String getFilterSerialNumber(int instrumentIndex, int filterIndex);
/**
* Get the Type property of Filter.
*
* @param instrumentIndex the Instrument index.
* @param filterIndex the Filter index.
* @return the Type property.
*/
FilterType getFilterType(int instrumentIndex, int filterIndex);
/**
* Get the DichroicRef property of FilterSet.
*
* @param instrumentIndex the Instrument index.
* @param filterSetIndex the FilterSet index.
* @return the DichroicRef property.
*/
String getFilterSetDichroicRef(int instrumentIndex, int filterSetIndex);
/**
* Get the EmissionFilterRef property of FilterSet.
*
* @param instrumentIndex the Instrument index.
* @param filterSetIndex the FilterSet index.
* @param emissionFilterRefIndex EmissionFilterRef index.
* @return the EmissionFilterRef property.
*/
String getFilterSetEmissionFilterRef(int instrumentIndex, int filterSetIndex, int emissionFilterRefIndex);
/**
* Get the ExcitationFilterRef property of FilterSet.
*
* @param instrumentIndex the Instrument index.
* @param filterSetIndex the FilterSet index.
* @param excitationFilterRefIndex ExcitationFilterRef index.
* @return the ExcitationFilterRef property.
*/
String getFilterSetExcitationFilterRef(int instrumentIndex, int filterSetIndex, int excitationFilterRefIndex);
/**
* Get the ID property of FilterSet.
*
* @param instrumentIndex the Instrument index.
* @param filterSetIndex the FilterSet index.
* @return the ID property.
*/
String getFilterSetID(int instrumentIndex, int filterSetIndex);
/**
* Get the LotNumber property of FilterSet.
*
* @param instrumentIndex the Instrument index.
* @param filterSetIndex the FilterSet index.
* @return the LotNumber property.
*/
String getFilterSetLotNumber(int instrumentIndex, int filterSetIndex);
/**
* Get the Manufacturer property of FilterSet.
*
* @param instrumentIndex the Instrument index.
* @param filterSetIndex the FilterSet index.
* @return the Manufacturer property.
*/
String getFilterSetManufacturer(int instrumentIndex, int filterSetIndex);
/**
* Get the Model property of FilterSet.
*
* @param instrumentIndex the Instrument index.
* @param filterSetIndex the FilterSet index.
* @return the Model property.
*/
String getFilterSetModel(int instrumentIndex, int filterSetIndex);
/**
* Get the SerialNumber property of FilterSet.
*
* @param instrumentIndex the Instrument index.
* @param filterSetIndex the FilterSet index.
* @return the SerialNumber property.
*/
String getFilterSetSerialNumber(int instrumentIndex, int filterSetIndex);
/**
* Get the AnnotationRef property of Folder.
*
* @param folderIndex the Folder index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getFolderAnnotationRef(int folderIndex, int annotationRefIndex);
/**
* Get the Description property of Folder.
*
* @param folderIndex the Folder index.
* @return the Description property.
*/
String getFolderDescription(int folderIndex);
/**
* Get the FolderRef property of Folder.
*
* @param folderIndex the Folder index.
* @param folderRefIndex FolderRef index.
* @return the FolderRef property.
*/
String getFolderFolderRef(int folderIndex, int folderRefIndex);
/**
* Get the ID property of Folder.
*
* @param folderIndex the Folder index.
* @return the ID property.
*/
String getFolderID(int folderIndex);
/**
* Get the ImageRef property of Folder.
*
* @param folderIndex the Folder index.
* @param imageRefIndex ImageRef index.
* @return the ImageRef property.
*/
String getFolderImageRef(int folderIndex, int imageRefIndex);
/**
* Get the Name property of Folder.
*
* @param folderIndex the Folder index.
* @return the Name property.
*/
String getFolderName(int folderIndex);
/**
* Get the ROIRef property of Folder.
*
* @param folderIndex the Folder index.
* @param ROIRefIndex ROIRef index.
* @return the ROIRef property.
*/
String getFolderROIRef(int folderIndex, int ROIRefIndex);
/**
* Get the AnnotationRef property of GenericExcitationSource.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getGenericExcitationSourceAnnotationRef(int instrumentIndex, int lightSourceIndex, int annotationRefIndex);
/**
* Get the ID property of GenericExcitationSource.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the ID property.
*/
String getGenericExcitationSourceID(int instrumentIndex, int lightSourceIndex);
/**
* Get the LotNumber property of GenericExcitationSource.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the LotNumber property.
*/
String getGenericExcitationSourceLotNumber(int instrumentIndex, int lightSourceIndex);
/**
* Get the Manufacturer property of GenericExcitationSource.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the Manufacturer property.
*/
String getGenericExcitationSourceManufacturer(int instrumentIndex, int lightSourceIndex);
/**
* Get the Model property of GenericExcitationSource.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the Model property.
*/
String getGenericExcitationSourceModel(int instrumentIndex, int lightSourceIndex);
/**
* Get the Power property of GenericExcitationSource.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the Power property.
*/
Power getGenericExcitationSourcePower(int instrumentIndex, int lightSourceIndex);
/**
* Get the SerialNumber property of GenericExcitationSource.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the SerialNumber property.
*/
String getGenericExcitationSourceSerialNumber(int instrumentIndex, int lightSourceIndex);
/**
* Get the AcquisitionDate property of Image.
*
* @param imageIndex the Image index.
* @return the AcquisitionDate property.
*/
Timestamp getImageAcquisitionDate(int imageIndex);
/**
* Get the AnnotationRef property of Image.
*
* @param imageIndex the Image index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getImageAnnotationRef(int imageIndex, int annotationRefIndex);
/**
* Get the Description property of Image.
*
* @param imageIndex the Image index.
* @return the Description property.
*/
String getImageDescription(int imageIndex);
/**
* Get the ExperimentRef property of Image.
*
* @param imageIndex the Image index.
* @return the ExperimentRef property.
*/
String getImageExperimentRef(int imageIndex);
/**
* Get the ExperimenterGroupRef property of Image.
*
* @param imageIndex the Image index.
* @return the ExperimenterGroupRef property.
*/
String getImageExperimenterGroupRef(int imageIndex);
/**
* Get the ExperimenterRef property of Image.
*
* @param imageIndex the Image index.
* @return the ExperimenterRef property.
*/
String getImageExperimenterRef(int imageIndex);
/**
* Get the ID property of Image.
*
* @param imageIndex the Image index.
* @return the ID property.
*/
String getImageID(int imageIndex);
/**
* Get the InstrumentRef property of Image.
*
* @param imageIndex the Image index.
* @return the InstrumentRef property.
*/
String getImageInstrumentRef(int imageIndex);
/**
* Get the MicrobeamManipulationRef property of Image.
*
* @param imageIndex the Image index.
* @param microbeamManipulationRefIndex MicrobeamManipulationRef index.
* @return the MicrobeamManipulationRef property.
*/
String getImageMicrobeamManipulationRef(int imageIndex, int microbeamManipulationRefIndex);
/**
* Get the Name property of Image.
*
* @param imageIndex the Image index.
* @return the Name property.
*/
String getImageName(int imageIndex);
/**
* Get the ROIRef property of Image.
*
* @param imageIndex the Image index.
* @param ROIRefIndex ROIRef index.
* @return the ROIRef property.
*/
String getImageROIRef(int imageIndex, int ROIRefIndex);
/**
* Get the AirPressure property of ImagingEnvironment.
*
* @param imageIndex the Image index.
* @return the AirPressure property.
*/
Pressure getImagingEnvironmentAirPressure(int imageIndex);
/**
* Get the CO2Percent property of ImagingEnvironment.
*
* @param imageIndex the Image index.
* @return the CO2Percent property.
*/
PercentFraction getImagingEnvironmentCO2Percent(int imageIndex);
/**
* Get the Humidity property of ImagingEnvironment.
*
* @param imageIndex the Image index.
* @return the Humidity property.
*/
PercentFraction getImagingEnvironmentHumidity(int imageIndex);
/**
* Get the Temperature property of ImagingEnvironment.
*
* @param imageIndex the Image index.
* @return the Temperature property.
*/
Temperature getImagingEnvironmentTemperature(int imageIndex);
/**
* Get the AnnotationRef property of Instrument.
*
* @param instrumentIndex the Instrument index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getInstrumentAnnotationRef(int instrumentIndex, int annotationRefIndex);
/**
* Get the ID property of Instrument.
*
* @param instrumentIndex the Instrument index.
* @return the ID property.
*/
String getInstrumentID(int instrumentIndex);
/**
* Get the AnnotationRef property of Label.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getLabelAnnotationRef(int ROIIndex, int shapeIndex, int annotationRefIndex);
/**
* Get the FillColor property of Label.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FillColor property.
*/
Color getLabelFillColor(int ROIIndex, int shapeIndex);
/**
* Get the FillRule property of Label.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FillRule property.
*/
FillRule getLabelFillRule(int ROIIndex, int shapeIndex);
/**
* Get the FontFamily property of Label.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FontFamily property.
*/
FontFamily getLabelFontFamily(int ROIIndex, int shapeIndex);
/**
* Get the FontSize property of Label.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FontSize property.
*/
Length getLabelFontSize(int ROIIndex, int shapeIndex);
/**
* Get the FontStyle property of Label.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FontStyle property.
*/
FontStyle getLabelFontStyle(int ROIIndex, int shapeIndex);
/**
* Get the ID property of Label.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the ID property.
*/
String getLabelID(int ROIIndex, int shapeIndex);
/**
* Get the Locked property of Label.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Locked property.
*/
Boolean getLabelLocked(int ROIIndex, int shapeIndex);
/**
* Get the StrokeColor property of Label.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the StrokeColor property.
*/
Color getLabelStrokeColor(int ROIIndex, int shapeIndex);
/**
* Get the StrokeDashArray property of Label.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the StrokeDashArray property.
*/
String getLabelStrokeDashArray(int ROIIndex, int shapeIndex);
/**
* Get the StrokeWidth property of Label.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the StrokeWidth property.
*/
Length getLabelStrokeWidth(int ROIIndex, int shapeIndex);
/**
* Get the Text property of Label.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Text property.
*/
String getLabelText(int ROIIndex, int shapeIndex);
/**
* Get the TheC property of Label.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the TheC property.
*/
NonNegativeInteger getLabelTheC(int ROIIndex, int shapeIndex);
/**
* Get the TheT property of Label.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the TheT property.
*/
NonNegativeInteger getLabelTheT(int ROIIndex, int shapeIndex);
/**
* Get the TheZ property of Label.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the TheZ property.
*/
NonNegativeInteger getLabelTheZ(int ROIIndex, int shapeIndex);
/**
* Get the Transform property of Label.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Transform property.
*/
AffineTransform getLabelTransform(int ROIIndex, int shapeIndex);
/**
* Get the X property of Label.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the X property.
*/
Double getLabelX(int ROIIndex, int shapeIndex);
/**
* Get the Y property of Label.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Y property.
*/
Double getLabelY(int ROIIndex, int shapeIndex);
/**
* Get the AnnotationRef property of Laser.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getLaserAnnotationRef(int instrumentIndex, int lightSourceIndex, int annotationRefIndex);
/**
* Get the FrequencyMultiplication property of Laser.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the FrequencyMultiplication property.
*/
PositiveInteger getLaserFrequencyMultiplication(int instrumentIndex, int lightSourceIndex);
/**
* Get the ID property of Laser.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the ID property.
*/
String getLaserID(int instrumentIndex, int lightSourceIndex);
/**
* Get the LaserMedium property of Laser.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the LaserMedium property.
*/
LaserMedium getLaserLaserMedium(int instrumentIndex, int lightSourceIndex);
/**
* Get the LotNumber property of Laser.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the LotNumber property.
*/
String getLaserLotNumber(int instrumentIndex, int lightSourceIndex);
/**
* Get the Manufacturer property of Laser.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the Manufacturer property.
*/
String getLaserManufacturer(int instrumentIndex, int lightSourceIndex);
/**
* Get the Model property of Laser.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the Model property.
*/
String getLaserModel(int instrumentIndex, int lightSourceIndex);
/**
* Get the PockelCell property of Laser.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the PockelCell property.
*/
Boolean getLaserPockelCell(int instrumentIndex, int lightSourceIndex);
/**
* Get the Power property of Laser.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the Power property.
*/
Power getLaserPower(int instrumentIndex, int lightSourceIndex);
/**
* Get the Pulse property of Laser.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the Pulse property.
*/
Pulse getLaserPulse(int instrumentIndex, int lightSourceIndex);
/**
* Get the Pump property of Laser.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the Pump property.
*/
String getLaserPump(int instrumentIndex, int lightSourceIndex);
/**
* Get the RepetitionRate property of Laser.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the RepetitionRate property.
*/
Frequency getLaserRepetitionRate(int instrumentIndex, int lightSourceIndex);
/**
* Get the SerialNumber property of Laser.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the SerialNumber property.
*/
String getLaserSerialNumber(int instrumentIndex, int lightSourceIndex);
/**
* Get the Tuneable property of Laser.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the Tuneable property.
*/
Boolean getLaserTuneable(int instrumentIndex, int lightSourceIndex);
/**
* Get the Type property of Laser.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the Type property.
*/
LaserType getLaserType(int instrumentIndex, int lightSourceIndex);
/**
* Get the Wavelength property of Laser.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the Wavelength property.
*/
Length getLaserWavelength(int instrumentIndex, int lightSourceIndex);
/**
* Get the AnnotationRef property of LightEmittingDiode.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getLightEmittingDiodeAnnotationRef(int instrumentIndex, int lightSourceIndex, int annotationRefIndex);
/**
* Get the ID property of LightEmittingDiode.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the ID property.
*/
String getLightEmittingDiodeID(int instrumentIndex, int lightSourceIndex);
/**
* Get the LotNumber property of LightEmittingDiode.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the LotNumber property.
*/
String getLightEmittingDiodeLotNumber(int instrumentIndex, int lightSourceIndex);
/**
* Get the Manufacturer property of LightEmittingDiode.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the Manufacturer property.
*/
String getLightEmittingDiodeManufacturer(int instrumentIndex, int lightSourceIndex);
/**
* Get the Model property of LightEmittingDiode.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the Model property.
*/
String getLightEmittingDiodeModel(int instrumentIndex, int lightSourceIndex);
/**
* Get the Power property of LightEmittingDiode.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the Power property.
*/
Power getLightEmittingDiodePower(int instrumentIndex, int lightSourceIndex);
/**
* Get the SerialNumber property of LightEmittingDiode.
*
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @return the SerialNumber property.
*/
String getLightEmittingDiodeSerialNumber(int instrumentIndex, int lightSourceIndex);
/**
* Get the AnnotationRef property of LightPath.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getLightPathAnnotationRef(int imageIndex, int channelIndex, int annotationRefIndex);
/**
* Get the DichroicRef property of LightPath.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the DichroicRef property.
*/
String getLightPathDichroicRef(int imageIndex, int channelIndex);
/**
* Get the EmissionFilterRef property of LightPath.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @param emissionFilterRefIndex EmissionFilterRef index.
* @return the EmissionFilterRef property.
*/
String getLightPathEmissionFilterRef(int imageIndex, int channelIndex, int emissionFilterRefIndex);
/**
* Get the ExcitationFilterRef property of LightPath.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @param excitationFilterRefIndex ExcitationFilterRef index.
* @return the ExcitationFilterRef property.
*/
String getLightPathExcitationFilterRef(int imageIndex, int channelIndex, int excitationFilterRefIndex);
/**
* Get the Attenuation property of LightSourceSettings.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the Attenuation property.
*/
PercentFraction getChannelLightSourceSettingsAttenuation(int imageIndex, int channelIndex);
/**
* Get the Attenuation property of LightSourceSettings.
*
* @param experimentIndex the Experiment index.
* @param microbeamManipulationIndex the MicrobeamManipulation index.
* @param lightSourceSettingsIndex the LightSourceSettings index.
* @return the Attenuation property.
*/
PercentFraction getMicrobeamManipulationLightSourceSettingsAttenuation(int experimentIndex, int microbeamManipulationIndex, int lightSourceSettingsIndex);
/**
* Get the ID property of LightSourceSettings.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the ID property.
*/
String getChannelLightSourceSettingsID(int imageIndex, int channelIndex);
/**
* Get the ID property of LightSourceSettings.
*
* @param experimentIndex the Experiment index.
* @param microbeamManipulationIndex the MicrobeamManipulation index.
* @param lightSourceSettingsIndex the LightSourceSettings index.
* @return the ID property.
*/
String getMicrobeamManipulationLightSourceSettingsID(int experimentIndex, int microbeamManipulationIndex, int lightSourceSettingsIndex);
/**
* Get the Wavelength property of LightSourceSettings.
*
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @return the Wavelength property.
*/
Length getChannelLightSourceSettingsWavelength(int imageIndex, int channelIndex);
/**
* Get the Wavelength property of LightSourceSettings.
*
* @param experimentIndex the Experiment index.
* @param microbeamManipulationIndex the MicrobeamManipulation index.
* @param lightSourceSettingsIndex the LightSourceSettings index.
* @return the Wavelength property.
*/
Length getMicrobeamManipulationLightSourceSettingsWavelength(int experimentIndex, int microbeamManipulationIndex, int lightSourceSettingsIndex);
/**
* Get the AnnotationRef property of Line.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getLineAnnotationRef(int ROIIndex, int shapeIndex, int annotationRefIndex);
/**
* Get the FillColor property of Line.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FillColor property.
*/
Color getLineFillColor(int ROIIndex, int shapeIndex);
/**
* Get the FillRule property of Line.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FillRule property.
*/
FillRule getLineFillRule(int ROIIndex, int shapeIndex);
/**
* Get the FontFamily property of Line.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FontFamily property.
*/
FontFamily getLineFontFamily(int ROIIndex, int shapeIndex);
/**
* Get the FontSize property of Line.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FontSize property.
*/
Length getLineFontSize(int ROIIndex, int shapeIndex);
/**
* Get the FontStyle property of Line.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FontStyle property.
*/
FontStyle getLineFontStyle(int ROIIndex, int shapeIndex);
/**
* Get the ID property of Line.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the ID property.
*/
String getLineID(int ROIIndex, int shapeIndex);
/**
* Get the Locked property of Line.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Locked property.
*/
Boolean getLineLocked(int ROIIndex, int shapeIndex);
/**
* Get the MarkerEnd property of Line.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the MarkerEnd property.
*/
Marker getLineMarkerEnd(int ROIIndex, int shapeIndex);
/**
* Get the MarkerStart property of Line.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the MarkerStart property.
*/
Marker getLineMarkerStart(int ROIIndex, int shapeIndex);
/**
* Get the StrokeColor property of Line.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the StrokeColor property.
*/
Color getLineStrokeColor(int ROIIndex, int shapeIndex);
/**
* Get the StrokeDashArray property of Line.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the StrokeDashArray property.
*/
String getLineStrokeDashArray(int ROIIndex, int shapeIndex);
/**
* Get the StrokeWidth property of Line.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the StrokeWidth property.
*/
Length getLineStrokeWidth(int ROIIndex, int shapeIndex);
/**
* Get the Text property of Line.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Text property.
*/
String getLineText(int ROIIndex, int shapeIndex);
/**
* Get the TheC property of Line.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the TheC property.
*/
NonNegativeInteger getLineTheC(int ROIIndex, int shapeIndex);
/**
* Get the TheT property of Line.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the TheT property.
*/
NonNegativeInteger getLineTheT(int ROIIndex, int shapeIndex);
/**
* Get the TheZ property of Line.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the TheZ property.
*/
NonNegativeInteger getLineTheZ(int ROIIndex, int shapeIndex);
/**
* Get the Transform property of Line.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Transform property.
*/
AffineTransform getLineTransform(int ROIIndex, int shapeIndex);
/**
* Get the X1 property of Line.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the X1 property.
*/
Double getLineX1(int ROIIndex, int shapeIndex);
/**
* Get the X2 property of Line.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the X2 property.
*/
Double getLineX2(int ROIIndex, int shapeIndex);
/**
* Get the Y1 property of Line.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Y1 property.
*/
Double getLineY1(int ROIIndex, int shapeIndex);
/**
* Get the Y2 property of Line.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Y2 property.
*/
Double getLineY2(int ROIIndex, int shapeIndex);
/**
* Get the AnnotationRef property of ListAnnotation.
*
* @param listAnnotationIndex the ListAnnotation index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getListAnnotationAnnotationRef(int listAnnotationIndex, int annotationRefIndex);
/**
* Get the Annotator property of ListAnnotation.
*
* @param listAnnotationIndex the ListAnnotation index.
* @return the Annotator property.
*/
String getListAnnotationAnnotator(int listAnnotationIndex);
/**
* Get the Description property of ListAnnotation.
*
* @param listAnnotationIndex the ListAnnotation index.
* @return the Description property.
*/
String getListAnnotationDescription(int listAnnotationIndex);
/**
* Get the ID property of ListAnnotation.
*
* @param listAnnotationIndex the ListAnnotation index.
* @return the ID property.
*/
String getListAnnotationID(int listAnnotationIndex);
/**
* Get the Namespace property of ListAnnotation.
*
* @param listAnnotationIndex the ListAnnotation index.
* @return the Namespace property.
*/
String getListAnnotationNamespace(int listAnnotationIndex);
/**
* Get the AnnotationRef property of LongAnnotation.
*
* @param longAnnotationIndex the LongAnnotation index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getLongAnnotationAnnotationRef(int longAnnotationIndex, int annotationRefIndex);
/**
* Get the Annotator property of LongAnnotation.
*
* @param longAnnotationIndex the LongAnnotation index.
* @return the Annotator property.
*/
String getLongAnnotationAnnotator(int longAnnotationIndex);
/**
* Get the Description property of LongAnnotation.
*
* @param longAnnotationIndex the LongAnnotation index.
* @return the Description property.
*/
String getLongAnnotationDescription(int longAnnotationIndex);
/**
* Get the ID property of LongAnnotation.
*
* @param longAnnotationIndex the LongAnnotation index.
* @return the ID property.
*/
String getLongAnnotationID(int longAnnotationIndex);
/**
* Get the Namespace property of LongAnnotation.
*
* @param longAnnotationIndex the LongAnnotation index.
* @return the Namespace property.
*/
String getLongAnnotationNamespace(int longAnnotationIndex);
/**
* Get the Value property of LongAnnotation.
*
* @param longAnnotationIndex the LongAnnotation index.
* @return the Value property.
*/
Long getLongAnnotationValue(int longAnnotationIndex);
/**
* Get the AnnotationRef property of MapAnnotation.
*
* @param mapAnnotationIndex the MapAnnotation index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getMapAnnotationAnnotationRef(int mapAnnotationIndex, int annotationRefIndex);
/**
* Get the Annotator property of MapAnnotation.
*
* @param mapAnnotationIndex the MapAnnotation index.
* @return the Annotator property.
*/
String getMapAnnotationAnnotator(int mapAnnotationIndex);
/**
* Get the Description property of MapAnnotation.
*
* @param mapAnnotationIndex the MapAnnotation index.
* @return the Description property.
*/
String getMapAnnotationDescription(int mapAnnotationIndex);
/**
* Get the ID property of MapAnnotation.
*
* @param mapAnnotationIndex the MapAnnotation index.
* @return the ID property.
*/
String getMapAnnotationID(int mapAnnotationIndex);
/**
* Get the Namespace property of MapAnnotation.
*
* @param mapAnnotationIndex the MapAnnotation index.
* @return the Namespace property.
*/
String getMapAnnotationNamespace(int mapAnnotationIndex);
/**
* Get the AnnotationRef property of Mask.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getMaskAnnotationRef(int ROIIndex, int shapeIndex, int annotationRefIndex);
/**
* Get the FillColor property of Mask.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FillColor property.
*/
Color getMaskFillColor(int ROIIndex, int shapeIndex);
/**
* Get the FillRule property of Mask.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FillRule property.
*/
FillRule getMaskFillRule(int ROIIndex, int shapeIndex);
/**
* Get the FontFamily property of Mask.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FontFamily property.
*/
FontFamily getMaskFontFamily(int ROIIndex, int shapeIndex);
/**
* Get the FontSize property of Mask.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FontSize property.
*/
Length getMaskFontSize(int ROIIndex, int shapeIndex);
/**
* Get the FontStyle property of Mask.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FontStyle property.
*/
FontStyle getMaskFontStyle(int ROIIndex, int shapeIndex);
/**
* Get the Height property of Mask.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Height property.
*/
Double getMaskHeight(int ROIIndex, int shapeIndex);
/**
* Get the ID property of Mask.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the ID property.
*/
String getMaskID(int ROIIndex, int shapeIndex);
/**
* Get the Locked property of Mask.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Locked property.
*/
Boolean getMaskLocked(int ROIIndex, int shapeIndex);
/**
* Get the StrokeColor property of Mask.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the StrokeColor property.
*/
Color getMaskStrokeColor(int ROIIndex, int shapeIndex);
/**
* Get the StrokeDashArray property of Mask.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the StrokeDashArray property.
*/
String getMaskStrokeDashArray(int ROIIndex, int shapeIndex);
/**
* Get the StrokeWidth property of Mask.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the StrokeWidth property.
*/
Length getMaskStrokeWidth(int ROIIndex, int shapeIndex);
/**
* Get the Text property of Mask.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Text property.
*/
String getMaskText(int ROIIndex, int shapeIndex);
/**
* Get the TheC property of Mask.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the TheC property.
*/
NonNegativeInteger getMaskTheC(int ROIIndex, int shapeIndex);
/**
* Get the TheT property of Mask.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the TheT property.
*/
NonNegativeInteger getMaskTheT(int ROIIndex, int shapeIndex);
/**
* Get the TheZ property of Mask.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the TheZ property.
*/
NonNegativeInteger getMaskTheZ(int ROIIndex, int shapeIndex);
/**
* Get the Transform property of Mask.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Transform property.
*/
AffineTransform getMaskTransform(int ROIIndex, int shapeIndex);
/**
* Get the Width property of Mask.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Width property.
*/
Double getMaskWidth(int ROIIndex, int shapeIndex);
/**
* Get the X property of Mask.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the X property.
*/
Double getMaskX(int ROIIndex, int shapeIndex);
/**
* Get the Y property of Mask.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Y property.
*/
Double getMaskY(int ROIIndex, int shapeIndex);
/**
* Get the Description property of MicrobeamManipulation.
*
* @param experimentIndex the Experiment index.
* @param microbeamManipulationIndex the MicrobeamManipulation index.
* @return the Description property.
*/
String getMicrobeamManipulationDescription(int experimentIndex, int microbeamManipulationIndex);
/**
* Get the ExperimenterRef property of MicrobeamManipulation.
*
* @param experimentIndex the Experiment index.
* @param microbeamManipulationIndex the MicrobeamManipulation index.
* @return the ExperimenterRef property.
*/
String getMicrobeamManipulationExperimenterRef(int experimentIndex, int microbeamManipulationIndex);
/**
* Get the ID property of MicrobeamManipulation.
*
* @param experimentIndex the Experiment index.
* @param microbeamManipulationIndex the MicrobeamManipulation index.
* @return the ID property.
*/
String getMicrobeamManipulationID(int experimentIndex, int microbeamManipulationIndex);
/**
* Get the ROIRef property of MicrobeamManipulation.
*
* @param experimentIndex the Experiment index.
* @param microbeamManipulationIndex the MicrobeamManipulation index.
* @param ROIRefIndex ROIRef index.
* @return the ROIRef property.
*/
String getMicrobeamManipulationROIRef(int experimentIndex, int microbeamManipulationIndex, int ROIRefIndex);
/**
* Get the Type property of MicrobeamManipulation.
*
* @param experimentIndex the Experiment index.
* @param microbeamManipulationIndex the MicrobeamManipulation index.
* @return the Type property.
*/
MicrobeamManipulationType getMicrobeamManipulationType(int experimentIndex, int microbeamManipulationIndex);
/**
* Get the LotNumber property of Microscope.
*
* @param instrumentIndex the Instrument index.
* @return the LotNumber property.
*/
String getMicroscopeLotNumber(int instrumentIndex);
/**
* Get the Manufacturer property of Microscope.
*
* @param instrumentIndex the Instrument index.
* @return the Manufacturer property.
*/
String getMicroscopeManufacturer(int instrumentIndex);
/**
* Get the Model property of Microscope.
*
* @param instrumentIndex the Instrument index.
* @return the Model property.
*/
String getMicroscopeModel(int instrumentIndex);
/**
* Get the SerialNumber property of Microscope.
*
* @param instrumentIndex the Instrument index.
* @return the SerialNumber property.
*/
String getMicroscopeSerialNumber(int instrumentIndex);
/**
* Get the Type property of Microscope.
*
* @param instrumentIndex the Instrument index.
* @return the Type property.
*/
MicroscopeType getMicroscopeType(int instrumentIndex);
/**
* Get the AnnotationRef property of Objective.
*
* @param instrumentIndex the Instrument index.
* @param objectiveIndex the Objective index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getObjectiveAnnotationRef(int instrumentIndex, int objectiveIndex, int annotationRefIndex);
/**
* Get the CalibratedMagnification property of Objective.
*
* @param instrumentIndex the Instrument index.
* @param objectiveIndex the Objective index.
* @return the CalibratedMagnification property.
*/
Double getObjectiveCalibratedMagnification(int instrumentIndex, int objectiveIndex);
/**
* Get the Correction property of Objective.
*
* @param instrumentIndex the Instrument index.
* @param objectiveIndex the Objective index.
* @return the Correction property.
*/
Correction getObjectiveCorrection(int instrumentIndex, int objectiveIndex);
/**
* Get the ID property of Objective.
*
* @param instrumentIndex the Instrument index.
* @param objectiveIndex the Objective index.
* @return the ID property.
*/
String getObjectiveID(int instrumentIndex, int objectiveIndex);
/**
* Get the Immersion property of Objective.
*
* @param instrumentIndex the Instrument index.
* @param objectiveIndex the Objective index.
* @return the Immersion property.
*/
Immersion getObjectiveImmersion(int instrumentIndex, int objectiveIndex);
/**
* Get the Iris property of Objective.
*
* @param instrumentIndex the Instrument index.
* @param objectiveIndex the Objective index.
* @return the Iris property.
*/
Boolean getObjectiveIris(int instrumentIndex, int objectiveIndex);
/**
* Get the LensNA property of Objective.
*
* @param instrumentIndex the Instrument index.
* @param objectiveIndex the Objective index.
* @return the LensNA property.
*/
Double getObjectiveLensNA(int instrumentIndex, int objectiveIndex);
/**
* Get the LotNumber property of Objective.
*
* @param instrumentIndex the Instrument index.
* @param objectiveIndex the Objective index.
* @return the LotNumber property.
*/
String getObjectiveLotNumber(int instrumentIndex, int objectiveIndex);
/**
* Get the Manufacturer property of Objective.
*
* @param instrumentIndex the Instrument index.
* @param objectiveIndex the Objective index.
* @return the Manufacturer property.
*/
String getObjectiveManufacturer(int instrumentIndex, int objectiveIndex);
/**
* Get the Model property of Objective.
*
* @param instrumentIndex the Instrument index.
* @param objectiveIndex the Objective index.
* @return the Model property.
*/
String getObjectiveModel(int instrumentIndex, int objectiveIndex);
/**
* Get the NominalMagnification property of Objective.
*
* @param instrumentIndex the Instrument index.
* @param objectiveIndex the Objective index.
* @return the NominalMagnification property.
*/
Double getObjectiveNominalMagnification(int instrumentIndex, int objectiveIndex);
/**
* Get the SerialNumber property of Objective.
*
* @param instrumentIndex the Instrument index.
* @param objectiveIndex the Objective index.
* @return the SerialNumber property.
*/
String getObjectiveSerialNumber(int instrumentIndex, int objectiveIndex);
/**
* Get the WorkingDistance property of Objective.
*
* @param instrumentIndex the Instrument index.
* @param objectiveIndex the Objective index.
* @return the WorkingDistance property.
*/
Length getObjectiveWorkingDistance(int instrumentIndex, int objectiveIndex);
/**
* Get the CorrectionCollar property of ObjectiveSettings.
*
* @param imageIndex the Image index.
* @return the CorrectionCollar property.
*/
Double getObjectiveSettingsCorrectionCollar(int imageIndex);
/**
* Get the ID property of ObjectiveSettings.
*
* @param imageIndex the Image index.
* @return the ID property.
*/
String getObjectiveSettingsID(int imageIndex);
/**
* Get the Medium property of ObjectiveSettings.
*
* @param imageIndex the Image index.
* @return the Medium property.
*/
Medium getObjectiveSettingsMedium(int imageIndex);
/**
* Get the RefractiveIndex property of ObjectiveSettings.
*
* @param imageIndex the Image index.
* @return the RefractiveIndex property.
*/
Double getObjectiveSettingsRefractiveIndex(int imageIndex);
/**
* Get the BigEndian property of Pixels.
*
* @param imageIndex the Image index.
* @return the BigEndian property.
*/
Boolean getPixelsBigEndian(int imageIndex);
/**
* Get the DimensionOrder property of Pixels.
*
* @param imageIndex the Image index.
* @return the DimensionOrder property.
*/
DimensionOrder getPixelsDimensionOrder(int imageIndex);
/**
* Get the ID property of Pixels.
*
* @param imageIndex the Image index.
* @return the ID property.
*/
String getPixelsID(int imageIndex);
/**
* Get the Interleaved property of Pixels.
*
* @param imageIndex the Image index.
* @return the Interleaved property.
*/
Boolean getPixelsInterleaved(int imageIndex);
/**
* Get the PhysicalSizeX property of Pixels.
*
* @param imageIndex the Image index.
* @return the PhysicalSizeX property.
*/
Length getPixelsPhysicalSizeX(int imageIndex);
/**
* Get the PhysicalSizeY property of Pixels.
*
* @param imageIndex the Image index.
* @return the PhysicalSizeY property.
*/
Length getPixelsPhysicalSizeY(int imageIndex);
/**
* Get the PhysicalSizeZ property of Pixels.
*
* @param imageIndex the Image index.
* @return the PhysicalSizeZ property.
*/
Length getPixelsPhysicalSizeZ(int imageIndex);
/**
* Get the SignificantBits property of Pixels.
*
* @param imageIndex the Image index.
* @return the SignificantBits property.
*/
PositiveInteger getPixelsSignificantBits(int imageIndex);
/**
* Get the SizeC property of Pixels.
*
* @param imageIndex the Image index.
* @return the SizeC property.
*/
PositiveInteger getPixelsSizeC(int imageIndex);
/**
* Get the SizeT property of Pixels.
*
* @param imageIndex the Image index.
* @return the SizeT property.
*/
PositiveInteger getPixelsSizeT(int imageIndex);
/**
* Get the SizeX property of Pixels.
*
* @param imageIndex the Image index.
* @return the SizeX property.
*/
PositiveInteger getPixelsSizeX(int imageIndex);
/**
* Get the SizeY property of Pixels.
*
* @param imageIndex the Image index.
* @return the SizeY property.
*/
PositiveInteger getPixelsSizeY(int imageIndex);
/**
* Get the SizeZ property of Pixels.
*
* @param imageIndex the Image index.
* @return the SizeZ property.
*/
PositiveInteger getPixelsSizeZ(int imageIndex);
/**
* Get the TimeIncrement property of Pixels.
*
* @param imageIndex the Image index.
* @return the TimeIncrement property.
*/
Time getPixelsTimeIncrement(int imageIndex);
/**
* Get the Type property of Pixels.
*
* @param imageIndex the Image index.
* @return the Type property.
*/
PixelType getPixelsType(int imageIndex);
/**
* Get the AnnotationRef property of Plane.
*
* @param imageIndex the Image index.
* @param planeIndex the Plane index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getPlaneAnnotationRef(int imageIndex, int planeIndex, int annotationRefIndex);
/**
* Get the DeltaT property of Plane.
*
* @param imageIndex the Image index.
* @param planeIndex the Plane index.
* @return the DeltaT property.
*/
Time getPlaneDeltaT(int imageIndex, int planeIndex);
/**
* Get the ExposureTime property of Plane.
*
* @param imageIndex the Image index.
* @param planeIndex the Plane index.
* @return the ExposureTime property.
*/
Time getPlaneExposureTime(int imageIndex, int planeIndex);
/**
* Get the HashSHA1 property of Plane.
*
* @param imageIndex the Image index.
* @param planeIndex the Plane index.
* @return the HashSHA1 property.
*/
String getPlaneHashSHA1(int imageIndex, int planeIndex);
/**
* Get the PositionX property of Plane.
*
* @param imageIndex the Image index.
* @param planeIndex the Plane index.
* @return the PositionX property.
*/
Length getPlanePositionX(int imageIndex, int planeIndex);
/**
* Get the PositionY property of Plane.
*
* @param imageIndex the Image index.
* @param planeIndex the Plane index.
* @return the PositionY property.
*/
Length getPlanePositionY(int imageIndex, int planeIndex);
/**
* Get the PositionZ property of Plane.
*
* @param imageIndex the Image index.
* @param planeIndex the Plane index.
* @return the PositionZ property.
*/
Length getPlanePositionZ(int imageIndex, int planeIndex);
/**
* Get the TheC property of Plane.
*
* @param imageIndex the Image index.
* @param planeIndex the Plane index.
* @return the TheC property.
*/
NonNegativeInteger getPlaneTheC(int imageIndex, int planeIndex);
/**
* Get the TheT property of Plane.
*
* @param imageIndex the Image index.
* @param planeIndex the Plane index.
* @return the TheT property.
*/
NonNegativeInteger getPlaneTheT(int imageIndex, int planeIndex);
/**
* Get the TheZ property of Plane.
*
* @param imageIndex the Image index.
* @param planeIndex the Plane index.
* @return the TheZ property.
*/
NonNegativeInteger getPlaneTheZ(int imageIndex, int planeIndex);
/**
* Get the AnnotationRef property of Plate.
*
* @param plateIndex the Plate index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getPlateAnnotationRef(int plateIndex, int annotationRefIndex);
/**
* Get the ColumnNamingConvention property of Plate.
*
* @param plateIndex the Plate index.
* @return the ColumnNamingConvention property.
*/
NamingConvention getPlateColumnNamingConvention(int plateIndex);
/**
* Get the Columns property of Plate.
*
* @param plateIndex the Plate index.
* @return the Columns property.
*/
PositiveInteger getPlateColumns(int plateIndex);
/**
* Get the Description property of Plate.
*
* @param plateIndex the Plate index.
* @return the Description property.
*/
String getPlateDescription(int plateIndex);
/**
* Get the ExternalIdentifier property of Plate.
*
* @param plateIndex the Plate index.
* @return the ExternalIdentifier property.
*/
String getPlateExternalIdentifier(int plateIndex);
/**
* Get the FieldIndex property of Plate.
*
* @param plateIndex the Plate index.
* @return the FieldIndex property.
*/
NonNegativeInteger getPlateFieldIndex(int plateIndex);
/**
* Get the ID property of Plate.
*
* @param plateIndex the Plate index.
* @return the ID property.
*/
String getPlateID(int plateIndex);
/**
* Get the Name property of Plate.
*
* @param plateIndex the Plate index.
* @return the Name property.
*/
String getPlateName(int plateIndex);
/**
* Get the RowNamingConvention property of Plate.
*
* @param plateIndex the Plate index.
* @return the RowNamingConvention property.
*/
NamingConvention getPlateRowNamingConvention(int plateIndex);
/**
* Get the Rows property of Plate.
*
* @param plateIndex the Plate index.
* @return the Rows property.
*/
PositiveInteger getPlateRows(int plateIndex);
/**
* Get the Status property of Plate.
*
* @param plateIndex the Plate index.
* @return the Status property.
*/
String getPlateStatus(int plateIndex);
/**
* Get the WellOriginX property of Plate.
*
* @param plateIndex the Plate index.
* @return the WellOriginX property.
*/
Length getPlateWellOriginX(int plateIndex);
/**
* Get the WellOriginY property of Plate.
*
* @param plateIndex the Plate index.
* @return the WellOriginY property.
*/
Length getPlateWellOriginY(int plateIndex);
/**
* Get the AnnotationRef property of PlateAcquisition.
*
* @param plateIndex the Plate index.
* @param plateAcquisitionIndex the PlateAcquisition index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getPlateAcquisitionAnnotationRef(int plateIndex, int plateAcquisitionIndex, int annotationRefIndex);
/**
* Get the Description property of PlateAcquisition.
*
* @param plateIndex the Plate index.
* @param plateAcquisitionIndex the PlateAcquisition index.
* @return the Description property.
*/
String getPlateAcquisitionDescription(int plateIndex, int plateAcquisitionIndex);
/**
* Get the EndTime property of PlateAcquisition.
*
* @param plateIndex the Plate index.
* @param plateAcquisitionIndex the PlateAcquisition index.
* @return the EndTime property.
*/
Timestamp getPlateAcquisitionEndTime(int plateIndex, int plateAcquisitionIndex);
/**
* Get the ID property of PlateAcquisition.
*
* @param plateIndex the Plate index.
* @param plateAcquisitionIndex the PlateAcquisition index.
* @return the ID property.
*/
String getPlateAcquisitionID(int plateIndex, int plateAcquisitionIndex);
/**
* Get the MaximumFieldCount property of PlateAcquisition.
*
* @param plateIndex the Plate index.
* @param plateAcquisitionIndex the PlateAcquisition index.
* @return the MaximumFieldCount property.
*/
PositiveInteger getPlateAcquisitionMaximumFieldCount(int plateIndex, int plateAcquisitionIndex);
/**
* Get the Name property of PlateAcquisition.
*
* @param plateIndex the Plate index.
* @param plateAcquisitionIndex the PlateAcquisition index.
* @return the Name property.
*/
String getPlateAcquisitionName(int plateIndex, int plateAcquisitionIndex);
/**
* Get the StartTime property of PlateAcquisition.
*
* @param plateIndex the Plate index.
* @param plateAcquisitionIndex the PlateAcquisition index.
* @return the StartTime property.
*/
Timestamp getPlateAcquisitionStartTime(int plateIndex, int plateAcquisitionIndex);
/**
* Get the WellSampleRef property of PlateAcquisition.
*
* @param plateIndex the Plate index.
* @param plateAcquisitionIndex the PlateAcquisition index.
* @param wellSampleRefIndex WellSampleRef index.
* @return the WellSampleRef property.
*/
String getPlateAcquisitionWellSampleRef(int plateIndex, int plateAcquisitionIndex, int wellSampleRefIndex);
/**
* Get the AnnotationRef property of Point.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getPointAnnotationRef(int ROIIndex, int shapeIndex, int annotationRefIndex);
/**
* Get the FillColor property of Point.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FillColor property.
*/
Color getPointFillColor(int ROIIndex, int shapeIndex);
/**
* Get the FillRule property of Point.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FillRule property.
*/
FillRule getPointFillRule(int ROIIndex, int shapeIndex);
/**
* Get the FontFamily property of Point.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FontFamily property.
*/
FontFamily getPointFontFamily(int ROIIndex, int shapeIndex);
/**
* Get the FontSize property of Point.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FontSize property.
*/
Length getPointFontSize(int ROIIndex, int shapeIndex);
/**
* Get the FontStyle property of Point.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FontStyle property.
*/
FontStyle getPointFontStyle(int ROIIndex, int shapeIndex);
/**
* Get the ID property of Point.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the ID property.
*/
String getPointID(int ROIIndex, int shapeIndex);
/**
* Get the Locked property of Point.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Locked property.
*/
Boolean getPointLocked(int ROIIndex, int shapeIndex);
/**
* Get the StrokeColor property of Point.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the StrokeColor property.
*/
Color getPointStrokeColor(int ROIIndex, int shapeIndex);
/**
* Get the StrokeDashArray property of Point.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the StrokeDashArray property.
*/
String getPointStrokeDashArray(int ROIIndex, int shapeIndex);
/**
* Get the StrokeWidth property of Point.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the StrokeWidth property.
*/
Length getPointStrokeWidth(int ROIIndex, int shapeIndex);
/**
* Get the Text property of Point.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Text property.
*/
String getPointText(int ROIIndex, int shapeIndex);
/**
* Get the TheC property of Point.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the TheC property.
*/
NonNegativeInteger getPointTheC(int ROIIndex, int shapeIndex);
/**
* Get the TheT property of Point.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the TheT property.
*/
NonNegativeInteger getPointTheT(int ROIIndex, int shapeIndex);
/**
* Get the TheZ property of Point.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the TheZ property.
*/
NonNegativeInteger getPointTheZ(int ROIIndex, int shapeIndex);
/**
* Get the Transform property of Point.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Transform property.
*/
AffineTransform getPointTransform(int ROIIndex, int shapeIndex);
/**
* Get the X property of Point.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the X property.
*/
Double getPointX(int ROIIndex, int shapeIndex);
/**
* Get the Y property of Point.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Y property.
*/
Double getPointY(int ROIIndex, int shapeIndex);
/**
* Get the AnnotationRef property of Polygon.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getPolygonAnnotationRef(int ROIIndex, int shapeIndex, int annotationRefIndex);
/**
* Get the FillColor property of Polygon.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FillColor property.
*/
Color getPolygonFillColor(int ROIIndex, int shapeIndex);
/**
* Get the FillRule property of Polygon.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FillRule property.
*/
FillRule getPolygonFillRule(int ROIIndex, int shapeIndex);
/**
* Get the FontFamily property of Polygon.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FontFamily property.
*/
FontFamily getPolygonFontFamily(int ROIIndex, int shapeIndex);
/**
* Get the FontSize property of Polygon.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FontSize property.
*/
Length getPolygonFontSize(int ROIIndex, int shapeIndex);
/**
* Get the FontStyle property of Polygon.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FontStyle property.
*/
FontStyle getPolygonFontStyle(int ROIIndex, int shapeIndex);
/**
* Get the ID property of Polygon.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the ID property.
*/
String getPolygonID(int ROIIndex, int shapeIndex);
/**
* Get the Locked property of Polygon.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Locked property.
*/
Boolean getPolygonLocked(int ROIIndex, int shapeIndex);
/**
* Get the Points property of Polygon.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Points property.
*/
String getPolygonPoints(int ROIIndex, int shapeIndex);
/**
* Get the StrokeColor property of Polygon.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the StrokeColor property.
*/
Color getPolygonStrokeColor(int ROIIndex, int shapeIndex);
/**
* Get the StrokeDashArray property of Polygon.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the StrokeDashArray property.
*/
String getPolygonStrokeDashArray(int ROIIndex, int shapeIndex);
/**
* Get the StrokeWidth property of Polygon.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the StrokeWidth property.
*/
Length getPolygonStrokeWidth(int ROIIndex, int shapeIndex);
/**
* Get the Text property of Polygon.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Text property.
*/
String getPolygonText(int ROIIndex, int shapeIndex);
/**
* Get the TheC property of Polygon.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the TheC property.
*/
NonNegativeInteger getPolygonTheC(int ROIIndex, int shapeIndex);
/**
* Get the TheT property of Polygon.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the TheT property.
*/
NonNegativeInteger getPolygonTheT(int ROIIndex, int shapeIndex);
/**
* Get the TheZ property of Polygon.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the TheZ property.
*/
NonNegativeInteger getPolygonTheZ(int ROIIndex, int shapeIndex);
/**
* Get the Transform property of Polygon.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Transform property.
*/
AffineTransform getPolygonTransform(int ROIIndex, int shapeIndex);
/**
* Get the AnnotationRef property of Polyline.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getPolylineAnnotationRef(int ROIIndex, int shapeIndex, int annotationRefIndex);
/**
* Get the FillColor property of Polyline.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FillColor property.
*/
Color getPolylineFillColor(int ROIIndex, int shapeIndex);
/**
* Get the FillRule property of Polyline.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FillRule property.
*/
FillRule getPolylineFillRule(int ROIIndex, int shapeIndex);
/**
* Get the FontFamily property of Polyline.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FontFamily property.
*/
FontFamily getPolylineFontFamily(int ROIIndex, int shapeIndex);
/**
* Get the FontSize property of Polyline.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FontSize property.
*/
Length getPolylineFontSize(int ROIIndex, int shapeIndex);
/**
* Get the FontStyle property of Polyline.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FontStyle property.
*/
FontStyle getPolylineFontStyle(int ROIIndex, int shapeIndex);
/**
* Get the ID property of Polyline.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the ID property.
*/
String getPolylineID(int ROIIndex, int shapeIndex);
/**
* Get the Locked property of Polyline.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Locked property.
*/
Boolean getPolylineLocked(int ROIIndex, int shapeIndex);
/**
* Get the MarkerEnd property of Polyline.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the MarkerEnd property.
*/
Marker getPolylineMarkerEnd(int ROIIndex, int shapeIndex);
/**
* Get the MarkerStart property of Polyline.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the MarkerStart property.
*/
Marker getPolylineMarkerStart(int ROIIndex, int shapeIndex);
/**
* Get the Points property of Polyline.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Points property.
*/
String getPolylinePoints(int ROIIndex, int shapeIndex);
/**
* Get the StrokeColor property of Polyline.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the StrokeColor property.
*/
Color getPolylineStrokeColor(int ROIIndex, int shapeIndex);
/**
* Get the StrokeDashArray property of Polyline.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the StrokeDashArray property.
*/
String getPolylineStrokeDashArray(int ROIIndex, int shapeIndex);
/**
* Get the StrokeWidth property of Polyline.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the StrokeWidth property.
*/
Length getPolylineStrokeWidth(int ROIIndex, int shapeIndex);
/**
* Get the Text property of Polyline.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Text property.
*/
String getPolylineText(int ROIIndex, int shapeIndex);
/**
* Get the TheC property of Polyline.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the TheC property.
*/
NonNegativeInteger getPolylineTheC(int ROIIndex, int shapeIndex);
/**
* Get the TheT property of Polyline.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the TheT property.
*/
NonNegativeInteger getPolylineTheT(int ROIIndex, int shapeIndex);
/**
* Get the TheZ property of Polyline.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the TheZ property.
*/
NonNegativeInteger getPolylineTheZ(int ROIIndex, int shapeIndex);
/**
* Get the Transform property of Polyline.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Transform property.
*/
AffineTransform getPolylineTransform(int ROIIndex, int shapeIndex);
/**
* Get the AnnotationRef property of Project.
*
* @param projectIndex the Project index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getProjectAnnotationRef(int projectIndex, int annotationRefIndex);
/**
* Get the DatasetRef property of Project.
*
* @param projectIndex the Project index.
* @param datasetRefIndex DatasetRef index.
* @return the DatasetRef property.
*/
String getProjectDatasetRef(int projectIndex, int datasetRefIndex);
/**
* Get the Description property of Project.
*
* @param projectIndex the Project index.
* @return the Description property.
*/
String getProjectDescription(int projectIndex);
/**
* Get the ExperimenterGroupRef property of Project.
*
* @param projectIndex the Project index.
* @return the ExperimenterGroupRef property.
*/
String getProjectExperimenterGroupRef(int projectIndex);
/**
* Get the ExperimenterRef property of Project.
*
* @param projectIndex the Project index.
* @return the ExperimenterRef property.
*/
String getProjectExperimenterRef(int projectIndex);
/**
* Get the ID property of Project.
*
* @param projectIndex the Project index.
* @return the ID property.
*/
String getProjectID(int projectIndex);
/**
* Get the Name property of Project.
*
* @param projectIndex the Project index.
* @return the Name property.
*/
String getProjectName(int projectIndex);
/**
* Get the AnnotationRef property of ROI.
*
* @param ROIIndex the ROI index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getROIAnnotationRef(int ROIIndex, int annotationRefIndex);
/**
* Get the Description property of ROI.
*
* @param ROIIndex the ROI index.
* @return the Description property.
*/
String getROIDescription(int ROIIndex);
/**
* Get the ID property of ROI.
*
* @param ROIIndex the ROI index.
* @return the ID property.
*/
String getROIID(int ROIIndex);
/**
* Get the Name property of ROI.
*
* @param ROIIndex the ROI index.
* @return the Name property.
*/
String getROIName(int ROIIndex);
/**
* Get the AnnotationRef property of Reagent.
*
* @param screenIndex the Screen index.
* @param reagentIndex the Reagent index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getReagentAnnotationRef(int screenIndex, int reagentIndex, int annotationRefIndex);
/**
* Get the Description property of Reagent.
*
* @param screenIndex the Screen index.
* @param reagentIndex the Reagent index.
* @return the Description property.
*/
String getReagentDescription(int screenIndex, int reagentIndex);
/**
* Get the ID property of Reagent.
*
* @param screenIndex the Screen index.
* @param reagentIndex the Reagent index.
* @return the ID property.
*/
String getReagentID(int screenIndex, int reagentIndex);
/**
* Get the Name property of Reagent.
*
* @param screenIndex the Screen index.
* @param reagentIndex the Reagent index.
* @return the Name property.
*/
String getReagentName(int screenIndex, int reagentIndex);
/**
* Get the ReagentIdentifier property of Reagent.
*
* @param screenIndex the Screen index.
* @param reagentIndex the Reagent index.
* @return the ReagentIdentifier property.
*/
String getReagentReagentIdentifier(int screenIndex, int reagentIndex);
/**
* Get the AnnotationRef property of Rectangle.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getRectangleAnnotationRef(int ROIIndex, int shapeIndex, int annotationRefIndex);
/**
* Get the FillColor property of Rectangle.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FillColor property.
*/
Color getRectangleFillColor(int ROIIndex, int shapeIndex);
/**
* Get the FillRule property of Rectangle.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FillRule property.
*/
FillRule getRectangleFillRule(int ROIIndex, int shapeIndex);
/**
* Get the FontFamily property of Rectangle.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FontFamily property.
*/
FontFamily getRectangleFontFamily(int ROIIndex, int shapeIndex);
/**
* Get the FontSize property of Rectangle.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FontSize property.
*/
Length getRectangleFontSize(int ROIIndex, int shapeIndex);
/**
* Get the FontStyle property of Rectangle.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the FontStyle property.
*/
FontStyle getRectangleFontStyle(int ROIIndex, int shapeIndex);
/**
* Get the Height property of Rectangle.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Height property.
*/
Double getRectangleHeight(int ROIIndex, int shapeIndex);
/**
* Get the ID property of Rectangle.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the ID property.
*/
String getRectangleID(int ROIIndex, int shapeIndex);
/**
* Get the Locked property of Rectangle.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Locked property.
*/
Boolean getRectangleLocked(int ROIIndex, int shapeIndex);
/**
* Get the StrokeColor property of Rectangle.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the StrokeColor property.
*/
Color getRectangleStrokeColor(int ROIIndex, int shapeIndex);
/**
* Get the StrokeDashArray property of Rectangle.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the StrokeDashArray property.
*/
String getRectangleStrokeDashArray(int ROIIndex, int shapeIndex);
/**
* Get the StrokeWidth property of Rectangle.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the StrokeWidth property.
*/
Length getRectangleStrokeWidth(int ROIIndex, int shapeIndex);
/**
* Get the Text property of Rectangle.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Text property.
*/
String getRectangleText(int ROIIndex, int shapeIndex);
/**
* Get the TheC property of Rectangle.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the TheC property.
*/
NonNegativeInteger getRectangleTheC(int ROIIndex, int shapeIndex);
/**
* Get the TheT property of Rectangle.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the TheT property.
*/
NonNegativeInteger getRectangleTheT(int ROIIndex, int shapeIndex);
/**
* Get the TheZ property of Rectangle.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the TheZ property.
*/
NonNegativeInteger getRectangleTheZ(int ROIIndex, int shapeIndex);
/**
* Get the Transform property of Rectangle.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Transform property.
*/
AffineTransform getRectangleTransform(int ROIIndex, int shapeIndex);
/**
* Get the Width property of Rectangle.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Width property.
*/
Double getRectangleWidth(int ROIIndex, int shapeIndex);
/**
* Get the X property of Rectangle.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the X property.
*/
Double getRectangleX(int ROIIndex, int shapeIndex);
/**
* Get the Y property of Rectangle.
*
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @return the Y property.
*/
Double getRectangleY(int ROIIndex, int shapeIndex);
/**
* Get the RightsHeld property of Rights.
*
* @return the RightsHeld property.
*/
String getRightsRightsHeld();
/**
* Get the RightsHolder property of Rights.
*
* @return the RightsHolder property.
*/
String getRightsRightsHolder();
/**
* Get the AnnotationRef property of Screen.
*
* @param screenIndex the Screen index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getScreenAnnotationRef(int screenIndex, int annotationRefIndex);
/**
* Get the Description property of Screen.
*
* @param screenIndex the Screen index.
* @return the Description property.
*/
String getScreenDescription(int screenIndex);
/**
* Get the ID property of Screen.
*
* @param screenIndex the Screen index.
* @return the ID property.
*/
String getScreenID(int screenIndex);
/**
* Get the Name property of Screen.
*
* @param screenIndex the Screen index.
* @return the Name property.
*/
String getScreenName(int screenIndex);
/**
* Get the PlateRef property of Screen.
*
* @param screenIndex the Screen index.
* @param plateRefIndex PlateRef index.
* @return the PlateRef property.
*/
String getScreenPlateRef(int screenIndex, int plateRefIndex);
/**
* Get the ProtocolDescription property of Screen.
*
* @param screenIndex the Screen index.
* @return the ProtocolDescription property.
*/
String getScreenProtocolDescription(int screenIndex);
/**
* Get the ProtocolIdentifier property of Screen.
*
* @param screenIndex the Screen index.
* @return the ProtocolIdentifier property.
*/
String getScreenProtocolIdentifier(int screenIndex);
/**
* Get the ReagentSetDescription property of Screen.
*
* @param screenIndex the Screen index.
* @return the ReagentSetDescription property.
*/
String getScreenReagentSetDescription(int screenIndex);
/**
* Get the ReagentSetIdentifier property of Screen.
*
* @param screenIndex the Screen index.
* @return the ReagentSetIdentifier property.
*/
String getScreenReagentSetIdentifier(int screenIndex);
/**
* Get the Type property of Screen.
*
* @param screenIndex the Screen index.
* @return the Type property.
*/
String getScreenType(int screenIndex);
/**
* Get the Name property of StageLabel.
*
* @param imageIndex the Image index.
* @return the Name property.
*/
String getStageLabelName(int imageIndex);
/**
* Get the X property of StageLabel.
*
* @param imageIndex the Image index.
* @return the X property.
*/
Length getStageLabelX(int imageIndex);
/**
* Get the Y property of StageLabel.
*
* @param imageIndex the Image index.
* @return the Y property.
*/
Length getStageLabelY(int imageIndex);
/**
* Get the Z property of StageLabel.
*
* @param imageIndex the Image index.
* @return the Z property.
*/
Length getStageLabelZ(int imageIndex);
/**
* Get the AnnotationRef property of TagAnnotation.
*
* @param tagAnnotationIndex the TagAnnotation index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getTagAnnotationAnnotationRef(int tagAnnotationIndex, int annotationRefIndex);
/**
* Get the Annotator property of TagAnnotation.
*
* @param tagAnnotationIndex the TagAnnotation index.
* @return the Annotator property.
*/
String getTagAnnotationAnnotator(int tagAnnotationIndex);
/**
* Get the Description property of TagAnnotation.
*
* @param tagAnnotationIndex the TagAnnotation index.
* @return the Description property.
*/
String getTagAnnotationDescription(int tagAnnotationIndex);
/**
* Get the ID property of TagAnnotation.
*
* @param tagAnnotationIndex the TagAnnotation index.
* @return the ID property.
*/
String getTagAnnotationID(int tagAnnotationIndex);
/**
* Get the Namespace property of TagAnnotation.
*
* @param tagAnnotationIndex the TagAnnotation index.
* @return the Namespace property.
*/
String getTagAnnotationNamespace(int tagAnnotationIndex);
/**
* Get the Value property of TagAnnotation.
*
* @param tagAnnotationIndex the TagAnnotation index.
* @return the Value property.
*/
String getTagAnnotationValue(int tagAnnotationIndex);
/**
* Get the AnnotationRef property of TermAnnotation.
*
* @param termAnnotationIndex the TermAnnotation index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getTermAnnotationAnnotationRef(int termAnnotationIndex, int annotationRefIndex);
/**
* Get the Annotator property of TermAnnotation.
*
* @param termAnnotationIndex the TermAnnotation index.
* @return the Annotator property.
*/
String getTermAnnotationAnnotator(int termAnnotationIndex);
/**
* Get the Description property of TermAnnotation.
*
* @param termAnnotationIndex the TermAnnotation index.
* @return the Description property.
*/
String getTermAnnotationDescription(int termAnnotationIndex);
/**
* Get the ID property of TermAnnotation.
*
* @param termAnnotationIndex the TermAnnotation index.
* @return the ID property.
*/
String getTermAnnotationID(int termAnnotationIndex);
/**
* Get the Namespace property of TermAnnotation.
*
* @param termAnnotationIndex the TermAnnotation index.
* @return the Namespace property.
*/
String getTermAnnotationNamespace(int termAnnotationIndex);
/**
* Get the Value property of TermAnnotation.
*
* @param termAnnotationIndex the TermAnnotation index.
* @return the Value property.
*/
String getTermAnnotationValue(int termAnnotationIndex);
/**
* Get the FirstC property of TiffData.
*
* @param imageIndex the Image index.
* @param tiffDataIndex the TiffData index.
* @return the FirstC property.
*/
NonNegativeInteger getTiffDataFirstC(int imageIndex, int tiffDataIndex);
/**
* Get the FirstT property of TiffData.
*
* @param imageIndex the Image index.
* @param tiffDataIndex the TiffData index.
* @return the FirstT property.
*/
NonNegativeInteger getTiffDataFirstT(int imageIndex, int tiffDataIndex);
/**
* Get the FirstZ property of TiffData.
*
* @param imageIndex the Image index.
* @param tiffDataIndex the TiffData index.
* @return the FirstZ property.
*/
NonNegativeInteger getTiffDataFirstZ(int imageIndex, int tiffDataIndex);
/**
* Get the IFD property of TiffData.
*
* @param imageIndex the Image index.
* @param tiffDataIndex the TiffData index.
* @return the IFD property.
*/
NonNegativeInteger getTiffDataIFD(int imageIndex, int tiffDataIndex);
/**
* Get the PlaneCount property of TiffData.
*
* @param imageIndex the Image index.
* @param tiffDataIndex the TiffData index.
* @return the PlaneCount property.
*/
NonNegativeInteger getTiffDataPlaneCount(int imageIndex, int tiffDataIndex);
/**
* Get the AnnotationRef property of TimestampAnnotation.
*
* @param timestampAnnotationIndex the TimestampAnnotation index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getTimestampAnnotationAnnotationRef(int timestampAnnotationIndex, int annotationRefIndex);
/**
* Get the Annotator property of TimestampAnnotation.
*
* @param timestampAnnotationIndex the TimestampAnnotation index.
* @return the Annotator property.
*/
String getTimestampAnnotationAnnotator(int timestampAnnotationIndex);
/**
* Get the Description property of TimestampAnnotation.
*
* @param timestampAnnotationIndex the TimestampAnnotation index.
* @return the Description property.
*/
String getTimestampAnnotationDescription(int timestampAnnotationIndex);
/**
* Get the ID property of TimestampAnnotation.
*
* @param timestampAnnotationIndex the TimestampAnnotation index.
* @return the ID property.
*/
String getTimestampAnnotationID(int timestampAnnotationIndex);
/**
* Get the Namespace property of TimestampAnnotation.
*
* @param timestampAnnotationIndex the TimestampAnnotation index.
* @return the Namespace property.
*/
String getTimestampAnnotationNamespace(int timestampAnnotationIndex);
/**
* Get the Value property of TimestampAnnotation.
*
* @param timestampAnnotationIndex the TimestampAnnotation index.
* @return the Value property.
*/
Timestamp getTimestampAnnotationValue(int timestampAnnotationIndex);
/**
* Get the CutIn property of TransmittanceRange.
*
* @param instrumentIndex the Instrument index.
* @param filterIndex the Filter index.
* @return the CutIn property.
*/
Length getTransmittanceRangeCutIn(int instrumentIndex, int filterIndex);
/**
* Get the CutInTolerance property of TransmittanceRange.
*
* @param instrumentIndex the Instrument index.
* @param filterIndex the Filter index.
* @return the CutInTolerance property.
*/
Length getTransmittanceRangeCutInTolerance(int instrumentIndex, int filterIndex);
/**
* Get the CutOut property of TransmittanceRange.
*
* @param instrumentIndex the Instrument index.
* @param filterIndex the Filter index.
* @return the CutOut property.
*/
Length getTransmittanceRangeCutOut(int instrumentIndex, int filterIndex);
/**
* Get the CutOutTolerance property of TransmittanceRange.
*
* @param instrumentIndex the Instrument index.
* @param filterIndex the Filter index.
* @return the CutOutTolerance property.
*/
Length getTransmittanceRangeCutOutTolerance(int instrumentIndex, int filterIndex);
/**
* Get the Transmittance property of TransmittanceRange.
*
* @param instrumentIndex the Instrument index.
* @param filterIndex the Filter index.
* @return the Transmittance property.
*/
PercentFraction getTransmittanceRangeTransmittance(int instrumentIndex, int filterIndex);
/**
* Get the FileName property of UUID.
*
* @param imageIndex the Image index.
* @param tiffDataIndex the TiffData index.
* @return the FileName property.
*/
String getUUIDFileName(int imageIndex, int tiffDataIndex);
/**
* Get the AnnotationRef property of Well.
*
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getWellAnnotationRef(int plateIndex, int wellIndex, int annotationRefIndex);
/**
* Get the Color property of Well.
*
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
* @return the Color property.
*/
Color getWellColor(int plateIndex, int wellIndex);
/**
* Get the Column property of Well.
*
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
* @return the Column property.
*/
NonNegativeInteger getWellColumn(int plateIndex, int wellIndex);
/**
* Get the ExternalDescription property of Well.
*
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
* @return the ExternalDescription property.
*/
String getWellExternalDescription(int plateIndex, int wellIndex);
/**
* Get the ExternalIdentifier property of Well.
*
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
* @return the ExternalIdentifier property.
*/
String getWellExternalIdentifier(int plateIndex, int wellIndex);
/**
* Get the ID property of Well.
*
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
* @return the ID property.
*/
String getWellID(int plateIndex, int wellIndex);
/**
* Get the ReagentRef property of Well.
*
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
* @return the ReagentRef property.
*/
String getWellReagentRef(int plateIndex, int wellIndex);
/**
* Get the Row property of Well.
*
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
* @return the Row property.
*/
NonNegativeInteger getWellRow(int plateIndex, int wellIndex);
/**
* Get the Type property of Well.
*
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
* @return the Type property.
*/
String getWellType(int plateIndex, int wellIndex);
/**
* Get the ID property of WellSample.
*
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
* @param wellSampleIndex the WellSample index.
* @return the ID property.
*/
String getWellSampleID(int plateIndex, int wellIndex, int wellSampleIndex);
/**
* Get the ImageRef property of WellSample.
*
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
* @param wellSampleIndex the WellSample index.
* @return the ImageRef property.
*/
String getWellSampleImageRef(int plateIndex, int wellIndex, int wellSampleIndex);
/**
* Get the Index property of WellSample.
*
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
* @param wellSampleIndex the WellSample index.
* @return the Index property.
*/
NonNegativeInteger getWellSampleIndex(int plateIndex, int wellIndex, int wellSampleIndex);
/**
* Get the PositionX property of WellSample.
*
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
* @param wellSampleIndex the WellSample index.
* @return the PositionX property.
*/
Length getWellSamplePositionX(int plateIndex, int wellIndex, int wellSampleIndex);
/**
* Get the PositionY property of WellSample.
*
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
* @param wellSampleIndex the WellSample index.
* @return the PositionY property.
*/
Length getWellSamplePositionY(int plateIndex, int wellIndex, int wellSampleIndex);
/**
* Get the Timepoint property of WellSample.
*
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
* @param wellSampleIndex the WellSample index.
* @return the Timepoint property.
*/
Timestamp getWellSampleTimepoint(int plateIndex, int wellIndex, int wellSampleIndex);
/**
* Get the AnnotationRef property of XMLAnnotation.
*
* @param XMLAnnotationIndex the XMLAnnotation index.
* @param annotationRefIndex AnnotationRef index.
* @return the AnnotationRef property.
*/
String getXMLAnnotationAnnotationRef(int XMLAnnotationIndex, int annotationRefIndex);
/**
* Get the Annotator property of XMLAnnotation.
*
* @param XMLAnnotationIndex the XMLAnnotation index.
* @return the Annotator property.
*/
String getXMLAnnotationAnnotator(int XMLAnnotationIndex);
/**
* Get the Description property of XMLAnnotation.
*
* @param XMLAnnotationIndex the XMLAnnotation index.
* @return the Description property.
*/
String getXMLAnnotationDescription(int XMLAnnotationIndex);
/**
* Get the ID property of XMLAnnotation.
*
* @param XMLAnnotationIndex the XMLAnnotation index.
* @return the ID property.
*/
String getXMLAnnotationID(int XMLAnnotationIndex);
/**
* Get the Namespace property of XMLAnnotation.
*
* @param XMLAnnotationIndex the XMLAnnotation index.
* @return the Namespace property.
*/
String getXMLAnnotationNamespace(int XMLAnnotationIndex);
/**
* Get the Value property of XMLAnnotation.
*
* @param XMLAnnotationIndex the XMLAnnotation index.
* @return the Value property.
*/
String getXMLAnnotationValue(int XMLAnnotationIndex);
}