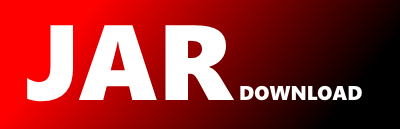
ome.xml.model.Shape Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ome-xml Show documentation
Show all versions of ome-xml Show documentation
OME XML model and metadata classes
/*
* #%L
* OME-XML Java library for working with OME-XML metadata structures.
* %%
* Copyright (C) 2006 - 2016 Open Microscopy Environment:
* - Massachusetts Institute of Technology
* - National Institutes of Health
* - University of Dundee
* - Board of Regents of the University of Wisconsin-Madison
* - Glencoe Software, Inc.
* %%
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDERS OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* The views and conclusions contained in the software and documentation are
* those of the authors and should not be interpreted as representing official
* policies, either expressed or implied, of any organization.
* #L%
*/
/*-----------------------------------------------------------------------------
*
* THIS IS AUTOMATICALLY GENERATED CODE. DO NOT MODIFY.
*
*-----------------------------------------------------------------------------
*/
package ome.xml.model;
import java.util.ArrayList;
import java.util.List;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import ome.units.quantity.Angle;
import ome.units.quantity.ElectricPotential;
import ome.units.quantity.Frequency;
import ome.units.quantity.Length;
import ome.units.quantity.Power;
import ome.units.quantity.Pressure;
import ome.units.quantity.Temperature;
import ome.units.quantity.Time;
import ome.units.unit.Unit;
import ome.xml.model.enums.*;
import ome.xml.model.enums.handlers.*;
import ome.xml.model.primitives.*;
public abstract class Shape extends AbstractOMEModelObject
{
// Base: -- Name: Shape -- Type: Shape -- modelBaseType: AbstractOMEModelObject -- langBaseType: Object
// -- Constants --
public static final String NAMESPACE = "http://www.openmicroscopy.org/Schemas/OME/2016-06";
/** Logger for this class. */
private static final Logger LOGGER =
LoggerFactory.getLogger(Shape.class);
// -- Instance variables --
// FillColor property
private Color fillColor;
// FillRule property
private FillRule fillRule;
// StrokeColor property
private Color strokeColor;
// StrokeWidth property
private Length strokeWidth;
// StrokeDashArray property
private String strokeDashArray;
// Text property
private String text;
// FontFamily property
private FontFamily fontFamily;
// FontSize property
private Length fontSize;
// FontStyle property
private FontStyle fontStyle;
// Locked property
private Boolean locked;
// ID property
private String id;
// TheZ property
private NonNegativeInteger theZ;
// TheT property
private NonNegativeInteger theT;
// TheC property
private NonNegativeInteger theC;
// Transform property
private AffineTransform transform;
// AnnotationRef reference (occurs more than once)
private List annotationLinks = new ReferenceList();
// Union_BackReference back reference
private Union union;
// -- Constructors --
/** Default constructor. */
public Shape()
{
}
/**
* Constructs Shape recursively from an XML DOM tree.
* @param element Root of the XML DOM tree to construct a model object
* graph from.
* @param model Handler for the OME model which keeps track of instances
* and references seen during object population.
* @throws EnumerationException If there is an error instantiating an
* enumeration during model object creation.
*/
public Shape(Element element, OMEModel model)
throws EnumerationException
{
update(element, model);
}
/** Copy constructor. */
public Shape(Shape orig)
{
fillColor = orig.fillColor;
fillRule = orig.fillRule;
strokeColor = orig.strokeColor;
strokeWidth = orig.strokeWidth;
strokeDashArray = orig.strokeDashArray;
text = orig.text;
fontFamily = orig.fontFamily;
fontSize = orig.fontSize;
fontStyle = orig.fontStyle;
locked = orig.locked;
id = orig.id;
theZ = orig.theZ;
theT = orig.theT;
theC = orig.theC;
transform = orig.transform;
annotationLinks = orig.annotationLinks;
union = orig.union;
}
// -- Custom content from Shape specific template --
// -- OMEModelObject API methods --
/**
* Updates Shape recursively from an XML DOM tree. NOTE: No
* properties are removed, only added or updated.
* @param element Root of the XML DOM tree to construct a model object
* graph from.
* @param model Handler for the OME model which keeps track of instances
* and references seen during object population.
* @throws EnumerationException If there is an error instantiating an
* enumeration during model object creation.
*/
public void update(Element element, OMEModel model)
throws EnumerationException
{
super.update(element, model);
String tagName = element.getTagName();
if (element.hasAttribute("FillColor"))
{
// Attribute property FillColor
setFillColor(Color.valueOf(
element.getAttribute("FillColor")));
}
if (element.hasAttribute("FillRule"))
{
// Attribute property which is an enumeration FillRule
setFillRule(FillRule.fromString(
element.getAttribute("FillRule")));
}
if (element.hasAttribute("StrokeColor"))
{
// Attribute property StrokeColor
setStrokeColor(Color.valueOf(
element.getAttribute("StrokeColor")));
}
if (element.hasAttribute("StrokeWidth"))
{
// Attribute property StrokeWidth with unit companion StrokeWidthUnit
String unitSymbol = element.getAttribute("StrokeWidthUnit");
if ((unitSymbol == null) || (unitSymbol.isEmpty()))
{
// Use default value specified in the xsd model
unitSymbol = getStrokeWidthUnitXsdDefault();
}
UnitsLength modelUnit =
UnitsLength.fromString(unitSymbol);
Double baseValue = Double.valueOf(
element.getAttribute("StrokeWidth"));
if (baseValue != null)
{
setStrokeWidth(UnitsLengthEnumHandler.getQuantity(baseValue, modelUnit));
}
}
if (element.hasAttribute("StrokeDashArray"))
{
// Attribute property StrokeDashArray
setStrokeDashArray(String.valueOf(
element.getAttribute("StrokeDashArray")));
}
if (element.hasAttribute("Text"))
{
// Attribute property Text
setText(String.valueOf(
element.getAttribute("Text")));
}
if (element.hasAttribute("FontFamily"))
{
// Attribute property which is an enumeration FontFamily
setFontFamily(FontFamily.fromString(
element.getAttribute("FontFamily")));
}
if (element.hasAttribute("FontSize"))
{
// Attribute property FontSize with unit companion FontSizeUnit
String unitSymbol = element.getAttribute("FontSizeUnit");
if ((unitSymbol == null) || (unitSymbol.isEmpty()))
{
// Use default value specified in the xsd model
unitSymbol = getFontSizeUnitXsdDefault();
}
UnitsLength modelUnit =
UnitsLength.fromString(unitSymbol);
NonNegativeInteger baseValue = NonNegativeInteger.valueOf(
element.getAttribute("FontSize"));
if (baseValue != null)
{
setFontSize(UnitsLengthEnumHandler.getQuantity(baseValue, modelUnit));
}
}
if (element.hasAttribute("FontStyle"))
{
// Attribute property which is an enumeration FontStyle
setFontStyle(FontStyle.fromString(
element.getAttribute("FontStyle")));
}
if (element.hasAttribute("Locked"))
{
// Attribute property Locked
setLocked(Boolean.valueOf(
element.getAttribute("Locked")));
}
if (!element.hasAttribute("ID") && getID() == null)
{
// TODO: Should be its own exception
throw new RuntimeException(String.format(
"Shape missing required ID property."));
}
if (element.hasAttribute("ID"))
{
// ID property
setID(String.valueOf(
element.getAttribute("ID")));
// Adding this model object to the model handler
model.addModelObject(getID(), this);
}
if (element.hasAttribute("TheZ"))
{
// Attribute property TheZ
setTheZ(NonNegativeInteger.valueOf(
element.getAttribute("TheZ")));
}
if (element.hasAttribute("TheT"))
{
// Attribute property TheT
setTheT(NonNegativeInteger.valueOf(
element.getAttribute("TheT")));
}
if (element.hasAttribute("TheC"))
{
// Attribute property TheC
setTheC(NonNegativeInteger.valueOf(
element.getAttribute("TheC")));
}
List Transform_nodeList =
getChildrenByTagName(element, "Transform");
if (Transform_nodeList.size() > 1)
{
// TODO: Should be its own Exception
throw new RuntimeException(String.format(
"Transform node list size %d != 1",
Transform_nodeList.size()));
}
else if (Transform_nodeList.size() != 0)
{
// Element property Transform which is complex (has
// sub-elements)
setTransform(new AffineTransform(
(Element) Transform_nodeList.get(0), model));
}
// Element reference AnnotationRef
List AnnotationRef_nodeList =
getChildrenByTagName(element, "AnnotationRef");
for (Element AnnotationRef_element : AnnotationRef_nodeList)
{
AnnotationRef annotationLinks_reference = new AnnotationRef();
annotationLinks_reference.setID(AnnotationRef_element.getAttribute("ID"));
model.addReference(this, annotationLinks_reference);
}
}
// -- Shape API methods --
public boolean link(Reference reference, OMEModelObject o)
{
if (reference instanceof AnnotationRef)
{
Annotation o_casted = (Annotation) o;
o_casted.linkShape(this);
annotationLinks.add(o_casted);
return true;
}
return super.link(reference, o);
}
// Property FillColor
public Color getFillColor()
{
return fillColor;
}
public void setFillColor(Color fillColor)
{
this.fillColor = fillColor;
}
// Property FillRule
public FillRule getFillRule()
{
return fillRule;
}
public void setFillRule(FillRule fillRule)
{
this.fillRule = fillRule;
}
// Property StrokeColor
public Color getStrokeColor()
{
return strokeColor;
}
public void setStrokeColor(Color strokeColor)
{
this.strokeColor = strokeColor;
}
// Property StrokeWidth with unit companion StrokeWidthUnit
public Length getStrokeWidth()
{
return strokeWidth;
}
public void setStrokeWidth(Length strokeWidth)
{
this.strokeWidth = strokeWidth;
}
// Property StrokeWidthUnit is a unit companion
public static String getStrokeWidthUnitXsdDefault()
{
return "pixel";
}
// Property StrokeDashArray
public String getStrokeDashArray()
{
return strokeDashArray;
}
public void setStrokeDashArray(String strokeDashArray)
{
this.strokeDashArray = strokeDashArray;
}
// Property Text
public String getText()
{
return text;
}
public void setText(String text)
{
this.text = text;
}
// Property FontFamily
public FontFamily getFontFamily()
{
return fontFamily;
}
public void setFontFamily(FontFamily fontFamily)
{
this.fontFamily = fontFamily;
}
// Property FontSize with unit companion FontSizeUnit
public Length getFontSize()
{
return fontSize;
}
public void setFontSize(Length fontSize)
{
this.fontSize = fontSize;
}
// Property FontSizeUnit is a unit companion
public static String getFontSizeUnitXsdDefault()
{
return "pt";
}
// Property FontStyle
public FontStyle getFontStyle()
{
return fontStyle;
}
public void setFontStyle(FontStyle fontStyle)
{
this.fontStyle = fontStyle;
}
// Property Locked
public Boolean getLocked()
{
return locked;
}
public void setLocked(Boolean locked)
{
this.locked = locked;
}
// Property ID
public String getID()
{
return id;
}
public void setID(String id)
{
this.id = id;
}
// Property TheZ
public NonNegativeInteger getTheZ()
{
return theZ;
}
public void setTheZ(NonNegativeInteger theZ)
{
this.theZ = theZ;
}
// Property TheT
public NonNegativeInteger getTheT()
{
return theT;
}
public void setTheT(NonNegativeInteger theT)
{
this.theT = theT;
}
// Property TheC
public NonNegativeInteger getTheC()
{
return theC;
}
public void setTheC(NonNegativeInteger theC)
{
this.theC = theC;
}
// Property Transform
public AffineTransform getTransform()
{
return transform;
}
public void setTransform(AffineTransform transform)
{
this.transform = transform;
}
// Reference which occurs more than once
public int sizeOfLinkedAnnotationList()
{
return annotationLinks.size();
}
public List copyLinkedAnnotationList()
{
return new ArrayList(annotationLinks);
}
public Annotation getLinkedAnnotation(int index)
{
return annotationLinks.get(index);
}
public Annotation setLinkedAnnotation(int index, Annotation o)
{
return annotationLinks.set(index, o);
}
public boolean linkAnnotation(Annotation o)
{
o.linkShape(this);
return annotationLinks.add(o);
}
public boolean unlinkAnnotation(Annotation o)
{
o.unlinkShape(this);
return annotationLinks.remove(o);
}
// Property Union_BackReference
public Union getUnion()
{
return union;
}
public void setUnion(Union union_BackReference)
{
this.union = union_BackReference;
}
public Element asXMLElement(Document document)
{
return asXMLElement(document, null);
}
public Element asXMLElement(Document document, Element Shape_element)
{
// Creating XML block for Shape
if (Shape_element == null)
{
Shape_element =
document.createElementNS(NAMESPACE, "Shape");
}
// Ensure any base annotations add their Elements first
super.asXMLElement(document, Shape_element);
if (fillColor != null)
{
// Attribute property FillColor
Shape_element.setAttribute("FillColor", fillColor.toString());
}
if (fillRule != null)
{
// Attribute property FillRule
Shape_element.setAttribute("FillRule", fillRule.toString());
}
if (strokeColor != null)
{
// Attribute property StrokeColor
Shape_element.setAttribute("StrokeColor", strokeColor.toString());
}
if (strokeWidth != null)
{
// Attribute property StrokeWidth with units companion prop.unitsCompanion.name
if (strokeWidth.value() != null)
{
Shape_element.setAttribute("StrokeWidth", strokeWidth.value().toString());
}
if (strokeWidth.unit() != null)
{
try
{
UnitsLength enumUnits = UnitsLength.fromString(strokeWidth.unit().getSymbol());
Shape_element.setAttribute("StrokeWidthUnit", enumUnits.toString());
} catch (EnumerationException e)
{
LOGGER.debug("Unable to create xml for Shape:StrokeWidthUnit: {}", e.toString());
}
}
}
if (strokeDashArray != null)
{
// Attribute property StrokeDashArray
Shape_element.setAttribute("StrokeDashArray", strokeDashArray.toString());
}
if (text != null)
{
// Attribute property Text
Shape_element.setAttribute("Text", text.toString());
}
if (fontFamily != null)
{
// Attribute property FontFamily
Shape_element.setAttribute("FontFamily", fontFamily.toString());
}
if (fontSize != null)
{
// Attribute property FontSize with units companion prop.unitsCompanion.name
if (fontSize.value() != null)
{
Shape_element.setAttribute("FontSize", Long.toString(fontSize.value().longValue()));
}
if (fontSize.unit() != null)
{
try
{
UnitsLength enumUnits = UnitsLength.fromString(fontSize.unit().getSymbol());
Shape_element.setAttribute("FontSizeUnit", enumUnits.toString());
} catch (EnumerationException e)
{
LOGGER.debug("Unable to create xml for Shape:FontSizeUnit: {}", e.toString());
}
}
}
if (fontStyle != null)
{
// Attribute property FontStyle
Shape_element.setAttribute("FontStyle", fontStyle.toString());
}
if (locked != null)
{
// Attribute property Locked
Shape_element.setAttribute("Locked", locked.toString());
}
if (id != null)
{
// Attribute property ID
Shape_element.setAttribute("ID", id.toString());
}
if (theZ != null)
{
// Attribute property TheZ
Shape_element.setAttribute("TheZ", theZ.toString());
}
if (theT != null)
{
// Attribute property TheT
Shape_element.setAttribute("TheT", theT.toString());
}
if (theC != null)
{
// Attribute property TheC
Shape_element.setAttribute("TheC", theC.toString());
}
if (transform != null)
{
// Element property Transform which is complex (has
// sub-elements)
Element child =
document.createElementNS(NAMESPACE, "Transform");
transform.asXMLElement(document, child);
Shape_element.appendChild(child);
}
if (annotationLinks != null)
{
// Reference property AnnotationRef which occurs more than once
for (Annotation annotationLinks_value : annotationLinks)
{
AnnotationRef o = new AnnotationRef();
o.setID(annotationLinks_value.getID());
Element child =
document.createElementNS(NAMESPACE, "AnnotationRef");
o.asXMLElement(document, child);
Shape_element.appendChild(child);
}
}
if (union != null)
{
// *** IGNORING *** Skipped back reference Union_BackReference
}
return Shape_element;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy