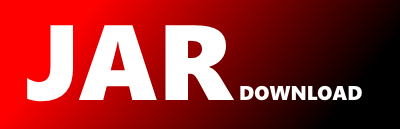
ome.xml.model.enums.UnitsLength Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ome-xml Show documentation
Show all versions of ome-xml Show documentation
OME XML model and metadata classes
/*
* #%L
* OME-XML Java library for working with OME-XML metadata structures.
* %%
* Copyright (C) 2006 - 2016 Open Microscopy Environment:
* - Massachusetts Institute of Technology
* - National Institutes of Health
* - University of Dundee
* - Board of Regents of the University of Wisconsin-Madison
* - Glencoe Software, Inc.
* %%
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDERS OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* The views and conclusions contained in the software and documentation are
* those of the authors and should not be interpreted as representing official
* policies, either expressed or implied, of any organization.
* #L%
*/
/*-----------------------------------------------------------------------------
*
* THIS IS AUTOMATICALLY GENERATED CODE. DO NOT MODIFY.
*
*-----------------------------------------------------------------------------
*/
package ome.xml.model.enums;
import ome.units.quantity.Angle;
import ome.units.quantity.ElectricPotential;
import ome.units.quantity.Frequency;
import ome.units.quantity.Length;
import ome.units.quantity.Power;
import ome.units.quantity.Pressure;
import ome.units.quantity.Temperature;
import ome.units.quantity.Time;
import ome.units.unit.Unit;
import ome.xml.model.enums.handlers.UnitsLengthEnumHandler;
import ome.xml.model.primitives.*;
public enum UnitsLength implements Enumeration
{
/** yottameter SI unit. */
YOTTAMETER("Ym"),
/** zettameter SI unit. */
ZETTAMETER("Zm"),
/** exameter SI unit. */
EXAMETER("Em"),
/** petameter SI unit. */
PETAMETER("Pm"),
/** terameter SI unit. */
TERAMETER("Tm"),
/** gigameter SI unit. */
GIGAMETER("Gm"),
/** megameter SI unit. */
MEGAMETER("Mm"),
/** kilometer SI unit. */
KILOMETER("km"),
/** hectometer SI unit. */
HECTOMETER("hm"),
/** decameter SI unit. */
DECAMETER("dam"),
/** meter SI unit. */
METER("m"),
/** decimeter SI unit. */
DECIMETER("dm"),
/** centimeter SI unit. */
CENTIMETER("cm"),
/** millimeter SI unit. */
MILLIMETER("mm"),
/** micrometer SI unit. */
MICROMETER("µm"),
/** nanometer SI unit. */
NANOMETER("nm"),
/** picometer SI unit. */
PICOMETER("pm"),
/** femtometer SI unit. */
FEMTOMETER("fm"),
/** attometer SI unit. */
ATTOMETER("am"),
/** zeptometer SI unit. */
ZEPTOMETER("zm"),
/** yoctometer SI unit. */
YOCTOMETER("ym"),
/** ångström SI-derived unit. */
ANGSTROM("Å"),
/** thou Imperial unit (or mil, 1/1000 inch). */
THOU("thou"),
/** line Imperial unit (1/12 inch). */
LINE("li"),
/** inch Imperial unit. */
INCH("in"),
/** foot Imperial unit. */
FOOT("ft"),
/** yard Imperial unit. */
YARD("yd"),
/** terrestrial mile Imperial unit. */
MILE("mi"),
/** astronomical unit SI-derived unit. The official term is ua as the SI standard assigned AU to absorbance unit. */
ASTRONOMICALUNIT("ua"),
/** light year. */
LIGHTYEAR("ly"),
/** parsec. */
PARSEC("pc"),
/** typography point Imperial-derived unit (1/72 inch). Use of this unit should be limited to font sizes. */
POINT("pt"),
/** pixel abstract unit. This is not convertible to any other length unit without a calibrated scaling factor. Its use should should be limited to ROI objects, and converted to an appropriate length units using the PhysicalSize units of the Image the ROI is attached to. */
PIXEL("pixel"),
/** reference frame abstract unit. This is not convertible to any other length unit without a scaling factor. Its use should be limited to uncalibrated stage positions, and converted to an appropriate length unit using a calibrated scaling factor. */
REFERENCEFRAME("reference frame");
private UnitsLength(String value)
{
this.value = value;
}
public static UnitsLength fromString(String value)
throws EnumerationException
{
if ("Ym".equals(value))
{
return YOTTAMETER;
}
if ("Zm".equals(value))
{
return ZETTAMETER;
}
if ("Em".equals(value))
{
return EXAMETER;
}
if ("Pm".equals(value))
{
return PETAMETER;
}
if ("Tm".equals(value))
{
return TERAMETER;
}
if ("Gm".equals(value))
{
return GIGAMETER;
}
if ("Mm".equals(value))
{
return MEGAMETER;
}
if ("km".equals(value))
{
return KILOMETER;
}
if ("hm".equals(value))
{
return HECTOMETER;
}
if ("dam".equals(value))
{
return DECAMETER;
}
if ("m".equals(value))
{
return METER;
}
if ("dm".equals(value))
{
return DECIMETER;
}
if ("cm".equals(value))
{
return CENTIMETER;
}
if ("mm".equals(value))
{
return MILLIMETER;
}
if ("µm".equals(value))
{
return MICROMETER;
}
if ("nm".equals(value))
{
return NANOMETER;
}
if ("pm".equals(value))
{
return PICOMETER;
}
if ("fm".equals(value))
{
return FEMTOMETER;
}
if ("am".equals(value))
{
return ATTOMETER;
}
if ("zm".equals(value))
{
return ZEPTOMETER;
}
if ("ym".equals(value))
{
return YOCTOMETER;
}
if ("Å".equals(value))
{
return ANGSTROM;
}
if ("thou".equals(value))
{
return THOU;
}
if ("li".equals(value))
{
return LINE;
}
if ("in".equals(value))
{
return INCH;
}
if ("ft".equals(value))
{
return FOOT;
}
if ("yd".equals(value))
{
return YARD;
}
if ("mi".equals(value))
{
return MILE;
}
if ("ua".equals(value))
{
return ASTRONOMICALUNIT;
}
if ("ly".equals(value))
{
return LIGHTYEAR;
}
if ("pc".equals(value))
{
return PARSEC;
}
if ("pt".equals(value))
{
return POINT;
}
if ("pixel".equals(value))
{
return PIXEL;
}
if ("reference frame".equals(value))
{
return REFERENCEFRAME;
}
String s = String.format("'%s' not a supported value of '%s'",
value, UnitsLength.class);
throw new EnumerationException(s);
}
public String getValue()
{
return value;
}
@Override
public String toString()
{
return value;
}
public static Length create(T newValue, UnitsLength newUnit)
{
Length theQuantity = null;
try
{
theQuantity = UnitsLengthEnumHandler.getQuantity(newValue, newUnit);
}
finally
{
return theQuantity;
}
}
public static Length create(T newValue, UnitsLength newUnit)
{
Length theQuantity = null;
try
{
theQuantity = UnitsLengthEnumHandler.getQuantity(newValue, newUnit);
}
finally
{
return theQuantity;
}
}
private final String value;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy