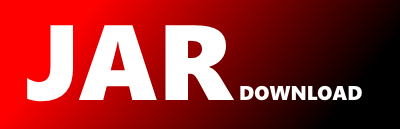
com.kscs.jaxb2.contract.test.Address Maven / Gradle / Ivy
//
// Diese Datei wurde mit der Eclipse Implementation of JAXB, v4.0.2 generiert
// Siehe https://eclipse-ee4j.github.io/jaxb-ri
// Änderungen an dieser Datei gehen bei einer Neukompilierung des Quellschemas verloren.
//
package com.kscs.jaxb2.contract.test;
import java.util.HashMap;
import java.util.Map;
import javax.xml.datatype.XMLGregorianCalendar;
import javax.xml.namespace.QName;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import com.kscs.util.jaxb.PropertyVisitor;
import com.kscs.util.jaxb.SingleProperty;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlAttribute;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlRootElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
/**
* Java-Klasse für anonymous complex type.
*
*
Das folgende Schemafragment gibt den erwarteten Content an, der in dieser Klasse enthalten ist.
*
*
{@code
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"street",
"city"
})
@XmlRootElement(name = "address")
public class Address implements Cloneable
{
@XmlElement(required = true)
protected String street;
@XmlElement(required = true)
protected City city;
@XmlAttribute(name = "created-at")
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar createdAt;
protected transient Address.Modifier __cachedModifier__;
/**
* Ruft den Wert der street-Eigenschaft ab.
*
* @return
* possible object is
* {@link String }
*
*/
public String getStreet() {
return street;
}
/**
* Legt den Wert der street-Eigenschaft fest.
*
* @param value
* allowed object is
* {@link String }
*
*/
protected void setStreet(String value) {
this.street = value;
}
/**
* Ruft den Wert der city-Eigenschaft ab.
*
* @return
* possible object is
* {@link City }
*
*/
public City getCity() {
return city;
}
/**
* Legt den Wert der city-Eigenschaft fest.
*
* @param value
* allowed object is
* {@link City }
*
*/
protected void setCity(City value) {
this.city = value;
}
/**
* Ruft den Wert der createdAt-Eigenschaft ab.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getCreatedAt() {
return createdAt;
}
/**
* Legt den Wert der createdAt-Eigenschaft fest.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
protected void setCreatedAt(XMLGregorianCalendar value) {
this.createdAt = value;
}
@Override
public Address clone() {
final Address _newObject;
try {
_newObject = ((Address) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
_newObject.city = ((this.city == null)?null:this.city.clone());
_newObject.createdAt = ((this.createdAt == null)?null:((XMLGregorianCalendar) this.createdAt.clone()));
return _newObject;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
public<_B >void copyTo(final Address.Builder<_B> _other) {
_other.street = this.street;
_other.city = ((this.city == null)?null:this.city.newCopyBuilder(_other));
_other.createdAt = ((this.createdAt == null)?null:((XMLGregorianCalendar) this.createdAt.clone()));
}
public<_B >Address.Builder<_B> newCopyBuilder(final _B _parentBuilder) {
return new Address.Builder<_B>(_parentBuilder, this, true);
}
public Address.Builder newCopyBuilder() {
return newCopyBuilder(null);
}
public static Address.Builder builder() {
return new Address.Builder<>(null, null, false);
}
public static<_B >Address.Builder<_B> copyOf(final Address _other) {
final Address.Builder<_B> _newBuilder = new Address.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
public<_B >void copyTo(final Address.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final PropertyTree streetPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("street"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(streetPropertyTree!= null):((streetPropertyTree == null)||(!streetPropertyTree.isLeaf())))) {
_other.street = this.street;
}
final PropertyTree cityPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("city"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(cityPropertyTree!= null):((cityPropertyTree == null)||(!cityPropertyTree.isLeaf())))) {
_other.city = ((this.city == null)?null:this.city.newCopyBuilder(_other, cityPropertyTree, _propertyTreeUse));
}
final PropertyTree createdAtPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("createdAt"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(createdAtPropertyTree!= null):((createdAtPropertyTree == null)||(!createdAtPropertyTree.isLeaf())))) {
_other.createdAt = ((this.createdAt == null)?null:((XMLGregorianCalendar) this.createdAt.clone()));
}
}
public<_B >Address.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return new Address.Builder<_B>(_parentBuilder, this, true, _propertyTree, _propertyTreeUse);
}
public Address.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return newCopyBuilder(null, _propertyTree, _propertyTreeUse);
}
public static<_B >Address.Builder<_B> copyOf(final Address _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Address.Builder<_B> _newBuilder = new Address.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
public static Address.Builder copyExcept(final Address _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
public static Address.Builder copyOnly(final Address _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
public Address.Modifier modifier() {
if (null == this.__cachedModifier__) {
this.__cachedModifier__ = new Address.Modifier();
}
return ((Address.Modifier) this.__cachedModifier__);
}
public Address visit(final PropertyVisitor _visitor_) {
_visitor_.visit(this);
_visitor_.visit(new SingleProperty<>(Address.PropInfo.street, this));
if (_visitor_.visit(new SingleProperty<>(Address.PropInfo.city, this))&&(this.city!= null)) {
this.city.visit(_visitor_);
}
_visitor_.visit(new SingleProperty<>(Address.PropInfo.createdAt, this));
return this;
}
public static class Builder<_B >implements Buildable
{
protected final _B _parentBuilder;
protected final Address _storedValue;
private String street;
private City.Builder> city;
private XMLGregorianCalendar createdAt;
public Builder(final _B _parentBuilder, final Address _other, final boolean _copy) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
this.street = _other.street;
this.city = ((_other.city == null)?null:_other.city.newCopyBuilder(this));
this.createdAt = ((_other.createdAt == null)?null:((XMLGregorianCalendar) _other.createdAt.clone()));
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public Builder(final _B _parentBuilder, final Address _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
final PropertyTree streetPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("street"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(streetPropertyTree!= null):((streetPropertyTree == null)||(!streetPropertyTree.isLeaf())))) {
this.street = _other.street;
}
final PropertyTree cityPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("city"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(cityPropertyTree!= null):((cityPropertyTree == null)||(!cityPropertyTree.isLeaf())))) {
this.city = ((_other.city == null)?null:_other.city.newCopyBuilder(this, cityPropertyTree, _propertyTreeUse));
}
final PropertyTree createdAtPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("createdAt"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(createdAtPropertyTree!= null):((createdAtPropertyTree == null)||(!createdAtPropertyTree.isLeaf())))) {
this.createdAt = ((_other.createdAt == null)?null:((XMLGregorianCalendar) _other.createdAt.clone()));
}
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public _B end() {
return this._parentBuilder;
}
protected<_P extends Address >_P init(final _P _product) {
_product.street = this.street;
_product.city = ((this.city == null)?null:this.city.build());
_product.createdAt = this.createdAt;
return _product;
}
/**
* Setzt den neuen Wert der Eigenschaft "street" (Vorher zugewiesener Wert wird
* ersetzt)
*
* @param street
* Neuer Wert der Eigenschaft "street".
*/
public Address.Builder<_B> withStreet(final String street) {
this.street = street;
return this;
}
/**
* Setzt den neuen Wert der Eigenschaft "city" (Vorher zugewiesener Wert wird
* ersetzt)
*
* @param city
* Neuer Wert der Eigenschaft "city".
*/
public Address.Builder<_B> withCity(final City city) {
this.city = ((city == null)?null:new City.Builder<>(this, city, false));
return this;
}
/**
* Erzeugt den vorhandenen Builder oder einen neuen "Builder" zum Zusammenbauen des
* Wertes der Eigenschaft "city".
* Mit {@link com.kscs.jaxb2.contract.test.City.Builder#end()} geht es zurück zum
* aktuellen Builder.
*
* @return
* Ein neuer "Builder" zum Zusammenbauen des Wertes der Eigenschaft "city".
* Mit {@link com.kscs.jaxb2.contract.test.City.Builder#end()} geht es zurück zum
* aktuellen Builder.
*/
public City.Builder extends Address.Builder<_B>> withCity() {
if (this.city!= null) {
return this.city;
}
return this.city = new City.Builder<>(this, null, false);
}
/**
* Setzt den neuen Wert der Eigenschaft "createdAt" (Vorher zugewiesener Wert wird
* ersetzt)
*
* @param createdAt
* Neuer Wert der Eigenschaft "createdAt".
*/
public Address.Builder<_B> withCreatedAt(final XMLGregorianCalendar createdAt) {
this.createdAt = createdAt;
return this;
}
@Override
public Address build() {
if (_storedValue == null) {
return this.init(new Address());
} else {
return ((Address) _storedValue);
}
}
public Address.Builder<_B> copyOf(final Address _other) {
_other.copyTo(this);
return this;
}
public Address.Builder<_B> copyOf(final Address.Builder _other) {
return copyOf(_other.build());
}
}
public class Modifier {
public void setStreet(final String street) {
Address.this.setStreet(street);
}
public void setCity(final City city) {
Address.this.setCity(city);
}
public void setCreatedAt(final XMLGregorianCalendar createdAt) {
Address.this.setCreatedAt(createdAt);
}
}
public static class PropInfo {
public static final transient SinglePropertyInfo street = new SinglePropertyInfo("street", Address.class, String.class, false, null, new QName("http://www.kscs.com/jaxb2/contract/test", "street"), new QName("http://www.w3.org/2001/XMLSchema", "string"), false) {
@Override
public String get(final Address _instance_) {
return ((_instance_ == null)?null:_instance_.street);
}
@Override
public void set(final Address _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.street = _value_;
}
}
}
;
public static final transient SinglePropertyInfo city = new SinglePropertyInfo("city", Address.class, City.class, false, null, new QName("http://www.kscs.com/jaxb2/contract/test", "city"), new QName("http://www.kscs.com/jaxb2/contract/test", "city"), false) {
@Override
public City get(final Address _instance_) {
return ((_instance_ == null)?null:_instance_.city);
}
@Override
public void set(final Address _instance_, final City _value_) {
if (_instance_!= null) {
_instance_.city = _value_;
}
}
}
;
public static final transient SinglePropertyInfo createdAt = new SinglePropertyInfo("createdAt", Address.class, XMLGregorianCalendar.class, false, null, new QName("", "created-at"), new QName("http://www.w3.org/2001/XMLSchema", "dateTime"), true) {
@Override
public XMLGregorianCalendar get(final Address _instance_) {
return ((_instance_ == null)?null:_instance_.createdAt);
}
@Override
public void set(final Address _instance_, final XMLGregorianCalendar _value_) {
if (_instance_!= null) {
_instance_.createdAt = _value_;
}
}
}
;
}
public static class Select
extends Address.Selector
{
Select() {
super(null, null, null);
}
public static Address.Select _root() {
return new Address.Select();
}
}
public static class Selector , TParent >
extends com.kscs.util.jaxb.Selector
{
private com.kscs.util.jaxb.Selector> street = null;
private City.Selector> city = null;
private com.kscs.util.jaxb.Selector> createdAt = null;
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap<>();
products.putAll(super.buildChildren());
if (this.street!= null) {
products.put("street", this.street.init());
}
if (this.city!= null) {
products.put("city", this.city.init());
}
if (this.createdAt!= null) {
products.put("createdAt", this.createdAt.init());
}
return products;
}
public com.kscs.util.jaxb.Selector> street() {
return ((this.street == null)?this.street = new com.kscs.util.jaxb.Selector<>(this._root, this, "street"):this.street);
}
public City.Selector> city() {
return ((this.city == null)?this.city = new City.Selector<>(this._root, this, "city"):this.city);
}
public com.kscs.util.jaxb.Selector> createdAt() {
return ((this.createdAt == null)?this.createdAt = new com.kscs.util.jaxb.Selector<>(this._root, this, "createdAt"):this.createdAt);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy