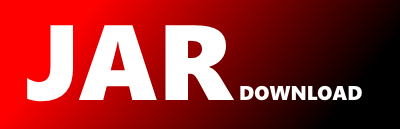
com.kscs.jaxb2.contract.test.Blacksmith Maven / Gradle / Ivy
//
// Diese Datei wurde mit der Eclipse Implementation of JAXB, v4.0.2 generiert
// Siehe https://eclipse-ee4j.github.io/jaxb-ri
// Änderungen an dieser Datei gehen bei einer Neukompilierung des Quellschemas verloren.
//
package com.kscs.jaxb2.contract.test;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.xml.namespace.QName;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.CollectionProperty;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import com.kscs.util.jaxb.PropertyVisitor;
import com.kscs.util.jaxb.SingleProperty;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
/**
* Java-Klasse für blacksmith complex type.
*
*
Das folgende Schemafragment gibt den erwarteten Content an, der in dieser Klasse enthalten ist.
*
*
{@code
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "blacksmith", propOrder = {
"tools",
"anvil"
})
public class Blacksmith
extends Worker
implements Cloneable
{
protected List tools;
@XmlElement(required = true)
protected String anvil;
protected transient List tools_RO = null;
/**
* Ruft den Wert der anvil-Eigenschaft ab.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAnvil() {
return anvil;
}
/**
* Legt den Wert der anvil-Eigenschaft fest.
*
* @param value
* allowed object is
* {@link String }
*
*/
protected void setAnvil(String value) {
this.anvil = value;
}
@Override
public Blacksmith clone() {
final Blacksmith _newObject;
_newObject = ((Blacksmith) super.clone());
_newObject.tools = ((this.tools == null)?null:new ArrayList<>(this.tools));
_newObject.tools_RO = ((tools == null)?null:Collections.unmodifiableList(_newObject.tools));
return _newObject;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
public<_B >void copyTo(final Blacksmith.Builder<_B> _other) {
super.copyTo(_other);
if (this.tools == null) {
_other.tools = null;
} else {
_other.tools = new ArrayList<>();
for (String _item: this.tools) {
_other.tools.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
_other.anvil = this.anvil;
}
@Override
public<_B >Blacksmith.Builder<_B> newCopyBuilder(final _B _parentBuilder) {
return new Blacksmith.Builder<_B>(_parentBuilder, this, true);
}
@Override
public Blacksmith.Builder newCopyBuilder() {
return newCopyBuilder(null);
}
public static Blacksmith.Builder builder() {
return new Blacksmith.Builder<>(null, null, false);
}
public static<_B >Blacksmith.Builder<_B> copyOf(final Person _other) {
final Blacksmith.Builder<_B> _newBuilder = new Blacksmith.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
public static<_B >Blacksmith.Builder<_B> copyOf(final Worker _other) {
final Blacksmith.Builder<_B> _newBuilder = new Blacksmith.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
public static<_B >Blacksmith.Builder<_B> copyOf(final Blacksmith _other) {
final Blacksmith.Builder<_B> _newBuilder = new Blacksmith.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
public<_B >void copyTo(final Blacksmith.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
super.copyTo(_other, _propertyTree, _propertyTreeUse);
final PropertyTree toolsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("tools"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(toolsPropertyTree!= null):((toolsPropertyTree == null)||(!toolsPropertyTree.isLeaf())))) {
if (this.tools == null) {
_other.tools = null;
} else {
_other.tools = new ArrayList<>();
for (String _item: this.tools) {
_other.tools.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
}
final PropertyTree anvilPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("anvil"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(anvilPropertyTree!= null):((anvilPropertyTree == null)||(!anvilPropertyTree.isLeaf())))) {
_other.anvil = this.anvil;
}
}
@Override
public<_B >Blacksmith.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return new Blacksmith.Builder<_B>(_parentBuilder, this, true, _propertyTree, _propertyTreeUse);
}
@Override
public Blacksmith.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return newCopyBuilder(null, _propertyTree, _propertyTreeUse);
}
public static<_B >Blacksmith.Builder<_B> copyOf(final Person _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Blacksmith.Builder<_B> _newBuilder = new Blacksmith.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
public static<_B >Blacksmith.Builder<_B> copyOf(final Worker _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Blacksmith.Builder<_B> _newBuilder = new Blacksmith.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
public static<_B >Blacksmith.Builder<_B> copyOf(final Blacksmith _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Blacksmith.Builder<_B> _newBuilder = new Blacksmith.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
public static Blacksmith.Builder copyExcept(final Person _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
public static Blacksmith.Builder copyExcept(final Worker _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
public static Blacksmith.Builder copyExcept(final Blacksmith _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
public static Blacksmith.Builder copyOnly(final Person _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
public static Blacksmith.Builder copyOnly(final Worker _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
public static Blacksmith.Builder copyOnly(final Blacksmith _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
public List getTools() {
if (this.tools == null) {
this.tools = new ArrayList<>();
}
if (this.tools_RO == null) {
this.tools_RO = ((tools == null)?null:Collections.unmodifiableList(this.tools));
}
return this.tools_RO;
}
public Blacksmith.Modifier modifier() {
if (null == this.__cachedModifier__) {
this.__cachedModifier__ = new Blacksmith.Modifier();
}
return ((Blacksmith.Modifier) this.__cachedModifier__);
}
public Blacksmith visit(final PropertyVisitor _visitor_) {
super.visit(_visitor_);
_visitor_.visit(new CollectionProperty<>(Blacksmith.PropInfo.tools, this));
_visitor_.visit(new SingleProperty<>(Blacksmith.PropInfo.anvil, this));
return this;
}
public static class Builder<_B >
extends Worker.Builder<_B>
implements Buildable
{
private List tools;
private String anvil;
public Builder(final _B _parentBuilder, final Blacksmith _other, final boolean _copy) {
super(_parentBuilder, _other, _copy);
if (_other!= null) {
if (_other.tools == null) {
this.tools = null;
} else {
this.tools = new ArrayList<>();
for (String _item: _other.tools) {
this.tools.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
this.anvil = _other.anvil;
}
}
public Builder(final _B _parentBuilder, final Blacksmith _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
super(_parentBuilder, _other, _copy, _propertyTree, _propertyTreeUse);
if (_other!= null) {
final PropertyTree toolsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("tools"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(toolsPropertyTree!= null):((toolsPropertyTree == null)||(!toolsPropertyTree.isLeaf())))) {
if (_other.tools == null) {
this.tools = null;
} else {
this.tools = new ArrayList<>();
for (String _item: _other.tools) {
this.tools.add(((_item == null)?null:new Buildable.PrimitiveBuildable(_item)));
}
}
}
final PropertyTree anvilPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("anvil"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(anvilPropertyTree!= null):((anvilPropertyTree == null)||(!anvilPropertyTree.isLeaf())))) {
this.anvil = _other.anvil;
}
}
}
protected<_P extends Blacksmith >_P init(final _P _product) {
if (this.tools!= null) {
final List tools = new ArrayList<>(this.tools.size());
for (Buildable _item: this.tools) {
tools.add(((String) _item.build()));
}
_product.tools = tools;
}
_product.tools_RO = ((tools == null)?null:Collections.unmodifiableList(_product.tools));
_product.anvil = this.anvil;
return super.init(_product);
}
/**
* Fügt Werte zur Eigenschaft "tools" hinzu.
*
* @param tools
* Werte, die zur Eigenschaft "tools" hinzugefügt werden.
*/
public Blacksmith.Builder<_B> addTools(final Iterable extends String> tools) {
if (tools!= null) {
if (this.tools == null) {
this.tools = new ArrayList<>();
}
for (String _item: tools) {
this.tools.add(new Buildable.PrimitiveBuildable(_item));
}
}
return this;
}
/**
* Setzt den neuen Wert der Eigenschaft "tools" (Vorher zugewiesener Wert wird
* ersetzt)
*
* @param tools
* Neuer Wert der Eigenschaft "tools".
*/
public Blacksmith.Builder<_B> withTools(final Iterable extends String> tools) {
if (this.tools!= null) {
this.tools.clear();
}
return addTools(tools);
}
/**
* Fügt Werte zur Eigenschaft "tools" hinzu.
*
* @param tools
* Werte, die zur Eigenschaft "tools" hinzugefügt werden.
*/
public Blacksmith.Builder<_B> addTools(String... tools) {
addTools(Arrays.asList(tools));
return this;
}
/**
* Setzt den neuen Wert der Eigenschaft "tools" (Vorher zugewiesener Wert wird
* ersetzt)
*
* @param tools
* Neuer Wert der Eigenschaft "tools".
*/
public Blacksmith.Builder<_B> withTools(String... tools) {
withTools(Arrays.asList(tools));
return this;
}
/**
* Setzt den neuen Wert der Eigenschaft "anvil" (Vorher zugewiesener Wert wird
* ersetzt)
*
* @param anvil
* Neuer Wert der Eigenschaft "anvil".
*/
public Blacksmith.Builder<_B> withAnvil(final String anvil) {
this.anvil = anvil;
return this;
}
/**
* Setzt den neuen Wert der Eigenschaft "salary" (Vorher zugewiesener Wert wird
* ersetzt)
*
* @param salary
* Neuer Wert der Eigenschaft "salary".
*/
@Override
public Blacksmith.Builder<_B> withSalary(final BigDecimal salary) {
super.withSalary(salary);
return this;
}
/**
* Setzt den neuen Wert der Eigenschaft "company" (Vorher zugewiesener Wert wird
* ersetzt)
*
* @param company
* Neuer Wert der Eigenschaft "company".
*/
@Override
public Blacksmith.Builder<_B> withCompany(final String company) {
super.withCompany(company);
return this;
}
/**
* Setzt den neuen Wert der Eigenschaft "name" (Vorher zugewiesener Wert wird
* ersetzt)
*
* @param name
* Neuer Wert der Eigenschaft "name".
*/
@Override
public Blacksmith.Builder<_B> withName(final String name) {
super.withName(name);
return this;
}
/**
* Setzt den neuen Wert der Eigenschaft "address" (Vorher zugewiesener Wert wird
* ersetzt)
*
* @param address
* Neuer Wert der Eigenschaft "address".
*/
@Override
public Blacksmith.Builder<_B> withAddress(final Address address) {
super.withAddress(address);
return this;
}
/**
* Erzeugt den vorhandenen Builder oder einen neuen "Builder" zum Zusammenbauen des
* Wertes der Eigenschaft "address".
* Mit {@link com.kscs.jaxb2.contract.test.Address.Builder#end()} geht es zurück
* zum aktuellen Builder.
*
* @return
* Ein neuer "Builder" zum Zusammenbauen des Wertes der Eigenschaft "address".
* Mit {@link com.kscs.jaxb2.contract.test.Address.Builder#end()} geht es zurück
* zum aktuellen Builder.
*/
public Address.Builder extends Blacksmith.Builder<_B>> withAddress() {
return ((Address.Builder extends Blacksmith.Builder<_B>> ) super.withAddress());
}
/**
* Setzt den neuen Wert der Eigenschaft "phoneNumber" (Vorher zugewiesener Wert
* wird ersetzt)
*
* @param phoneNumber
* Neuer Wert der Eigenschaft "phoneNumber".
*/
@Override
public Blacksmith.Builder<_B> withPhoneNumber(final long phoneNumber) {
super.withPhoneNumber(phoneNumber);
return this;
}
@Override
public Blacksmith build() {
if (_storedValue == null) {
return this.init(new Blacksmith());
} else {
return ((Blacksmith) _storedValue);
}
}
public Blacksmith.Builder<_B> copyOf(final Blacksmith _other) {
_other.copyTo(this);
return this;
}
public Blacksmith.Builder<_B> copyOf(final Blacksmith.Builder _other) {
return copyOf(_other.build());
}
}
public class Modifier
extends Worker.Modifier
{
public List getTools() {
if (Blacksmith.this.tools == null) {
Blacksmith.this.tools = new ArrayList<>();
}
return Blacksmith.this.tools;
}
public void setAnvil(final String anvil) {
Blacksmith.this.setAnvil(anvil);
}
}
public static class PropInfo {
public static final transient CollectionPropertyInfo tools = new CollectionPropertyInfo("tools", Blacksmith.class, String.class, true, null, new QName("http://www.kscs.com/jaxb2/contract/test", "tools"), new QName("http://www.w3.org/2001/XMLSchema", "string"), false) {
@Override
public List get(final Blacksmith _instance_) {
return ((_instance_ == null)?null:_instance_.tools);
}
@Override
public void set(final Blacksmith _instance_, final List _value_) {
if (_instance_!= null) {
_instance_.tools = _value_;
}
}
}
;
public static final transient SinglePropertyInfo anvil = new SinglePropertyInfo("anvil", Blacksmith.class, String.class, false, null, new QName("http://www.kscs.com/jaxb2/contract/test", "anvil"), new QName("http://www.w3.org/2001/XMLSchema", "string"), false) {
@Override
public String get(final Blacksmith _instance_) {
return ((_instance_ == null)?null:_instance_.anvil);
}
@Override
public void set(final Blacksmith _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.anvil = _value_;
}
}
}
;
}
public static class Select
extends Blacksmith.Selector
{
Select() {
super(null, null, null);
}
public static Blacksmith.Select _root() {
return new Blacksmith.Select();
}
}
public static class Selector , TParent >
extends Worker.Selector
{
private com.kscs.util.jaxb.Selector> tools = null;
private com.kscs.util.jaxb.Selector> anvil = null;
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap<>();
products.putAll(super.buildChildren());
if (this.tools!= null) {
products.put("tools", this.tools.init());
}
if (this.anvil!= null) {
products.put("anvil", this.anvil.init());
}
return products;
}
public com.kscs.util.jaxb.Selector> tools() {
return ((this.tools == null)?this.tools = new com.kscs.util.jaxb.Selector<>(this._root, this, "tools"):this.tools);
}
public com.kscs.util.jaxb.Selector> anvil() {
return ((this.anvil == null)?this.anvil = new com.kscs.util.jaxb.Selector<>(this._root, this, "anvil"):this.anvil);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy