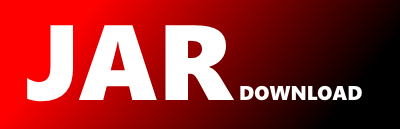
com.kscs.jaxb2.contract.test.Car Maven / Gradle / Ivy
//
// Diese Datei wurde mit der Eclipse Implementation of JAXB, v4.0.2 generiert
// Siehe https://eclipse-ee4j.github.io/jaxb-ri
// Änderungen an dieser Datei gehen bei einer Neukompilierung des Quellschemas verloren.
//
package com.kscs.jaxb2.contract.test;
import java.util.HashMap;
import java.util.Map;
import javax.xml.namespace.QName;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import com.kscs.util.jaxb.PropertyVisitor;
import com.kscs.util.jaxb.SingleProperty;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
/**
* car complex type. And this is some extra text to test that line breaking is working correctly.
*
* Java-Klasse für car complex type.
*
*
Das folgende Schemafragment gibt den erwarteten Content an, der in dieser Klasse enthalten ist.
*
*
{@code
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "car", propOrder = {
"engineSize"
})
public class Car
extends Transport
implements Cloneable
{
@XmlElement(name = "engine-size")
protected int engineSize;
/**
* Ruft den Wert der engineSize-Eigenschaft ab.
*
*/
public int getEngineSize() {
return engineSize;
}
/**
* Legt den Wert der engineSize-Eigenschaft fest.
*
*/
protected void setEngineSize(int value) {
this.engineSize = value;
}
@Override
public Car clone() {
final Car _newObject;
_newObject = ((Car) super.clone());
return _newObject;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
public<_B >void copyTo(final Car.Builder<_B> _other) {
super.copyTo(_other);
_other.engineSize = this.engineSize;
}
@Override
public<_B >Car.Builder<_B> newCopyBuilder(final _B _parentBuilder) {
return new Car.Builder<_B>(_parentBuilder, this, true);
}
@Override
public Car.Builder newCopyBuilder() {
return newCopyBuilder(null);
}
public static Car.Builder builder() {
return new Car.Builder<>(null, null, false);
}
public static<_B >Car.Builder<_B> copyOf(final Transport _other) {
final Car.Builder<_B> _newBuilder = new Car.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
public static<_B >Car.Builder<_B> copyOf(final Car _other) {
final Car.Builder<_B> _newBuilder = new Car.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
public<_B >void copyTo(final Car.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
super.copyTo(_other, _propertyTree, _propertyTreeUse);
final PropertyTree engineSizePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("engineSize"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(engineSizePropertyTree!= null):((engineSizePropertyTree == null)||(!engineSizePropertyTree.isLeaf())))) {
_other.engineSize = this.engineSize;
}
}
@Override
public<_B >Car.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return new Car.Builder<_B>(_parentBuilder, this, true, _propertyTree, _propertyTreeUse);
}
@Override
public Car.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return newCopyBuilder(null, _propertyTree, _propertyTreeUse);
}
public static<_B >Car.Builder<_B> copyOf(final Transport _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Car.Builder<_B> _newBuilder = new Car.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
public static<_B >Car.Builder<_B> copyOf(final Car _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final Car.Builder<_B> _newBuilder = new Car.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
public static Car.Builder copyExcept(final Transport _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
public static Car.Builder copyExcept(final Car _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
public static Car.Builder copyOnly(final Transport _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
public static Car.Builder copyOnly(final Car _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
public Car.Modifier modifier() {
if (null == this.__cachedModifier__) {
this.__cachedModifier__ = new Car.Modifier();
}
return ((Car.Modifier) this.__cachedModifier__);
}
public Car visit(final PropertyVisitor _visitor_) {
super.visit(_visitor_);
_visitor_.visit(new SingleProperty<>(Car.PropInfo.engineSize, this));
return this;
}
public static class Builder<_B >
extends Transport.Builder<_B>
implements Buildable
{
private int engineSize;
public Builder(final _B _parentBuilder, final Car _other, final boolean _copy) {
super(_parentBuilder, _other, _copy);
if (_other!= null) {
this.engineSize = _other.engineSize;
}
}
public Builder(final _B _parentBuilder, final Car _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
super(_parentBuilder, _other, _copy, _propertyTree, _propertyTreeUse);
if (_other!= null) {
final PropertyTree engineSizePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("engineSize"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(engineSizePropertyTree!= null):((engineSizePropertyTree == null)||(!engineSizePropertyTree.isLeaf())))) {
this.engineSize = _other.engineSize;
}
}
}
protected<_P extends Car >_P init(final _P _product) {
_product.engineSize = this.engineSize;
return super.init(_product);
}
/**
* Setzt den neuen Wert der Eigenschaft "engineSize" (Vorher zugewiesener Wert wird
* ersetzt)
*
* @param engineSize
* Neuer Wert der Eigenschaft "engineSize".
*/
public Car.Builder<_B> withEngineSize(final int engineSize) {
this.engineSize = engineSize;
return this;
}
/**
* Setzt den neuen Wert der Eigenschaft "name" (Vorher zugewiesener Wert wird
* ersetzt)
*
* @param name
* Neuer Wert der Eigenschaft "name".
*/
@Override
public Car.Builder<_B> withName(final String name) {
super.withName(name);
return this;
}
@Override
public Car build() {
if (_storedValue == null) {
return this.init(new Car());
} else {
return ((Car) _storedValue);
}
}
public Car.Builder<_B> copyOf(final Car _other) {
_other.copyTo(this);
return this;
}
public Car.Builder<_B> copyOf(final Car.Builder _other) {
return copyOf(_other.build());
}
}
public class Modifier
extends Transport.Modifier
{
public void setEngineSize(final int engineSize) {
Car.this.setEngineSize(engineSize);
}
}
public static class PropInfo {
public static final transient SinglePropertyInfo engineSize = new SinglePropertyInfo("engineSize", Car.class, Integer.class, false, null, new QName("http://www.kscs.com/jaxb2/contract/test", "engine-size"), new QName("http://www.w3.org/2001/XMLSchema", "int"), false) {
@Override
public Integer get(final Car _instance_) {
return ((_instance_ == null)?null:_instance_.engineSize);
}
@Override
public void set(final Car _instance_, final Integer _value_) {
if (_instance_!= null) {
_instance_.engineSize = _value_;
}
}
}
;
}
public static class Select
extends Car.Selector
{
Select() {
super(null, null, null);
}
public static Car.Select _root() {
return new Car.Select();
}
}
public static class Selector , TParent >
extends Transport.Selector
{
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap<>();
products.putAll(super.buildChildren());
return products;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy