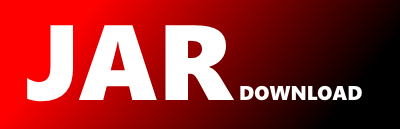
com.kscs.jaxb2.contract.test.City Maven / Gradle / Ivy
//
// Diese Datei wurde mit der Eclipse Implementation of JAXB, v4.0.2 generiert
// Siehe https://eclipse-ee4j.github.io/jaxb-ri
// Änderungen an dieser Datei gehen bei einer Neukompilierung des Quellschemas verloren.
//
package com.kscs.jaxb2.contract.test;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.xml.namespace.QName;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.CollectionProperty;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.CollectionPropertyInfo;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import com.kscs.util.jaxb.PropertyVisitor;
import com.kscs.util.jaxb.SingleProperty;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
/**
* Java-Klasse für city complex type.
*
*
Das folgende Schemafragment gibt den erwarteten Content an, der in dieser Klasse enthalten ist.
*
*
{@code
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "city", propOrder = {
"postalCode",
"town",
"inhabitants"
})
public class City implements Cloneable
{
@XmlElement(name = "postal-code", required = true)
protected String postalCode;
@XmlElement(required = true)
protected String town;
protected List inhabitants;
protected transient List inhabitants_RO = null;
protected transient City.Modifier __cachedModifier__;
/**
* Ruft den Wert der postalCode-Eigenschaft ab.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPostalCode() {
return postalCode;
}
/**
* Legt den Wert der postalCode-Eigenschaft fest.
*
* @param value
* allowed object is
* {@link String }
*
*/
protected void setPostalCode(String value) {
this.postalCode = value;
}
/**
* Ruft den Wert der town-Eigenschaft ab.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTown() {
return town;
}
/**
* Legt den Wert der town-Eigenschaft fest.
*
* @param value
* allowed object is
* {@link String }
*
*/
protected void setTown(String value) {
this.town = value;
}
@Override
public City clone() {
final City _newObject;
try {
_newObject = ((City) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
if (this.inhabitants == null) {
_newObject.inhabitants = null;
} else {
_newObject.inhabitants = new ArrayList<>();
for (Person _item: this.inhabitants) {
_newObject.inhabitants.add(((_item == null)?null:_item.clone()));
}
}
_newObject.inhabitants_RO = ((inhabitants == null)?null:Collections.unmodifiableList(_newObject.inhabitants));
return _newObject;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
public<_B >void copyTo(final City.Builder<_B> _other) {
_other.postalCode = this.postalCode;
_other.town = this.town;
if (this.inhabitants == null) {
_other.inhabitants = null;
} else {
_other.inhabitants = new ArrayList<>();
for (Person _item: this.inhabitants) {
_other.inhabitants.add(((_item == null)?null:_item.newCopyBuilder(_other)));
}
}
}
public<_B >City.Builder<_B> newCopyBuilder(final _B _parentBuilder) {
return new City.Builder<_B>(_parentBuilder, this, true);
}
public City.Builder newCopyBuilder() {
return newCopyBuilder(null);
}
public static City.Builder builder() {
return new City.Builder<>(null, null, false);
}
public static<_B >City.Builder<_B> copyOf(final City _other) {
final City.Builder<_B> _newBuilder = new City.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
public<_B >void copyTo(final City.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final PropertyTree postalCodePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("postalCode"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(postalCodePropertyTree!= null):((postalCodePropertyTree == null)||(!postalCodePropertyTree.isLeaf())))) {
_other.postalCode = this.postalCode;
}
final PropertyTree townPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("town"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(townPropertyTree!= null):((townPropertyTree == null)||(!townPropertyTree.isLeaf())))) {
_other.town = this.town;
}
final PropertyTree inhabitantsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("inhabitants"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(inhabitantsPropertyTree!= null):((inhabitantsPropertyTree == null)||(!inhabitantsPropertyTree.isLeaf())))) {
if (this.inhabitants == null) {
_other.inhabitants = null;
} else {
_other.inhabitants = new ArrayList<>();
for (Person _item: this.inhabitants) {
_other.inhabitants.add(((_item == null)?null:_item.newCopyBuilder(_other, inhabitantsPropertyTree, _propertyTreeUse)));
}
}
}
}
public<_B >City.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return new City.Builder<_B>(_parentBuilder, this, true, _propertyTree, _propertyTreeUse);
}
public City.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return newCopyBuilder(null, _propertyTree, _propertyTreeUse);
}
public static<_B >City.Builder<_B> copyOf(final City _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final City.Builder<_B> _newBuilder = new City.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
public static City.Builder copyExcept(final City _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
public static City.Builder copyOnly(final City _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
public List getInhabitants() {
if (this.inhabitants == null) {
this.inhabitants = new ArrayList<>();
}
if (this.inhabitants_RO == null) {
this.inhabitants_RO = ((inhabitants == null)?null:Collections.unmodifiableList(this.inhabitants));
}
return this.inhabitants_RO;
}
public City.Modifier modifier() {
if (null == this.__cachedModifier__) {
this.__cachedModifier__ = new City.Modifier();
}
return ((City.Modifier) this.__cachedModifier__);
}
public City visit(final PropertyVisitor _visitor_) {
_visitor_.visit(this);
_visitor_.visit(new SingleProperty<>(City.PropInfo.postalCode, this));
_visitor_.visit(new SingleProperty<>(City.PropInfo.town, this));
if (_visitor_.visit(new CollectionProperty<>(City.PropInfo.inhabitants, this))&&(this.inhabitants!= null)) {
for (Person _item_: this.inhabitants) {
if (_item_!= null) {
_item_.visit(_visitor_);
}
}
}
return this;
}
public static class Builder<_B >implements Buildable
{
protected final _B _parentBuilder;
protected final City _storedValue;
private String postalCode;
private String town;
private List>> inhabitants;
public Builder(final _B _parentBuilder, final City _other, final boolean _copy) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
this.postalCode = _other.postalCode;
this.town = _other.town;
if (_other.inhabitants == null) {
this.inhabitants = null;
} else {
this.inhabitants = new ArrayList<>();
for (Person _item: _other.inhabitants) {
this.inhabitants.add(((_item == null)?null:_item.newCopyBuilder(this)));
}
}
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public Builder(final _B _parentBuilder, final City _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
final PropertyTree postalCodePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("postalCode"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(postalCodePropertyTree!= null):((postalCodePropertyTree == null)||(!postalCodePropertyTree.isLeaf())))) {
this.postalCode = _other.postalCode;
}
final PropertyTree townPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("town"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(townPropertyTree!= null):((townPropertyTree == null)||(!townPropertyTree.isLeaf())))) {
this.town = _other.town;
}
final PropertyTree inhabitantsPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("inhabitants"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(inhabitantsPropertyTree!= null):((inhabitantsPropertyTree == null)||(!inhabitantsPropertyTree.isLeaf())))) {
if (_other.inhabitants == null) {
this.inhabitants = null;
} else {
this.inhabitants = new ArrayList<>();
for (Person _item: _other.inhabitants) {
this.inhabitants.add(((_item == null)?null:_item.newCopyBuilder(this, inhabitantsPropertyTree, _propertyTreeUse)));
}
}
}
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public _B end() {
return this._parentBuilder;
}
protected<_P extends City >_P init(final _P _product) {
_product.postalCode = this.postalCode;
_product.town = this.town;
if (this.inhabitants!= null) {
final List inhabitants = new ArrayList<>(this.inhabitants.size());
for (Person.Builder> _item: this.inhabitants) {
inhabitants.add(_item.build());
}
_product.inhabitants = inhabitants;
}
_product.inhabitants_RO = ((inhabitants == null)?null:Collections.unmodifiableList(_product.inhabitants));
return _product;
}
/**
* Setzt den neuen Wert der Eigenschaft "postalCode" (Vorher zugewiesener Wert wird
* ersetzt)
*
* @param postalCode
* Neuer Wert der Eigenschaft "postalCode".
*/
public City.Builder<_B> withPostalCode(final String postalCode) {
this.postalCode = postalCode;
return this;
}
/**
* Setzt den neuen Wert der Eigenschaft "town" (Vorher zugewiesener Wert wird
* ersetzt)
*
* @param town
* Neuer Wert der Eigenschaft "town".
*/
public City.Builder<_B> withTown(final String town) {
this.town = town;
return this;
}
/**
* Fügt Werte zur Eigenschaft "inhabitants" hinzu.
*
* @param inhabitants
* Werte, die zur Eigenschaft "inhabitants" hinzugefügt werden.
*/
public City.Builder<_B> addInhabitants(final Iterable extends Person> inhabitants) {
if (inhabitants!= null) {
if (this.inhabitants == null) {
this.inhabitants = new ArrayList<>();
}
for (Person _item: inhabitants) {
this.inhabitants.add(new Person.Builder<>(this, _item, false));
}
}
return this;
}
/**
* Setzt den neuen Wert der Eigenschaft "inhabitants" (Vorher zugewiesener Wert
* wird ersetzt)
*
* @param inhabitants
* Neuer Wert der Eigenschaft "inhabitants".
*/
public City.Builder<_B> withInhabitants(final Iterable extends Person> inhabitants) {
if (this.inhabitants!= null) {
this.inhabitants.clear();
}
return addInhabitants(inhabitants);
}
/**
* Fügt Werte zur Eigenschaft "inhabitants" hinzu.
*
* @param inhabitants
* Werte, die zur Eigenschaft "inhabitants" hinzugefügt werden.
*/
public City.Builder<_B> addInhabitants(Person... inhabitants) {
addInhabitants(Arrays.asList(inhabitants));
return this;
}
/**
* Setzt den neuen Wert der Eigenschaft "inhabitants" (Vorher zugewiesener Wert
* wird ersetzt)
*
* @param inhabitants
* Neuer Wert der Eigenschaft "inhabitants".
*/
public City.Builder<_B> withInhabitants(Person... inhabitants) {
withInhabitants(Arrays.asList(inhabitants));
return this;
}
@Override
public City build() {
if (_storedValue == null) {
return this.init(new City());
} else {
return ((City) _storedValue);
}
}
public City.Builder<_B> copyOf(final City _other) {
_other.copyTo(this);
return this;
}
public City.Builder<_B> copyOf(final City.Builder _other) {
return copyOf(_other.build());
}
}
public class Modifier {
public void setPostalCode(final String postalCode) {
City.this.setPostalCode(postalCode);
}
public void setTown(final String town) {
City.this.setTown(town);
}
public List getInhabitants() {
if (City.this.inhabitants == null) {
City.this.inhabitants = new ArrayList<>();
}
return City.this.inhabitants;
}
}
public static class PropInfo {
public static final transient SinglePropertyInfo postalCode = new SinglePropertyInfo("postalCode", City.class, String.class, false, null, new QName("http://www.kscs.com/jaxb2/contract/test", "postal-code"), new QName("http://www.w3.org/2001/XMLSchema", "string"), false) {
@Override
public String get(final City _instance_) {
return ((_instance_ == null)?null:_instance_.postalCode);
}
@Override
public void set(final City _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.postalCode = _value_;
}
}
}
;
public static final transient SinglePropertyInfo town = new SinglePropertyInfo("town", City.class, String.class, false, null, new QName("http://www.kscs.com/jaxb2/contract/test", "town"), new QName("http://www.w3.org/2001/XMLSchema", "string"), false) {
@Override
public String get(final City _instance_) {
return ((_instance_ == null)?null:_instance_.town);
}
@Override
public void set(final City _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.town = _value_;
}
}
}
;
public static final transient CollectionPropertyInfo inhabitants = new CollectionPropertyInfo("inhabitants", City.class, Person.class, true, null, new QName("http://www.kscs.com/jaxb2/contract/test", "inhabitants"), new QName("http://www.kscs.com/jaxb2/contract/test", "person"), false) {
@Override
public List get(final City _instance_) {
return ((_instance_ == null)?null:_instance_.inhabitants);
}
@Override
public void set(final City _instance_, final List _value_) {
if (_instance_!= null) {
_instance_.inhabitants = _value_;
}
}
}
;
}
public static class Select
extends City.Selector
{
Select() {
super(null, null, null);
}
public static City.Select _root() {
return new City.Select();
}
}
public static class Selector , TParent >
extends com.kscs.util.jaxb.Selector
{
private com.kscs.util.jaxb.Selector> postalCode = null;
private com.kscs.util.jaxb.Selector> town = null;
private Person.Selector> inhabitants = null;
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap<>();
products.putAll(super.buildChildren());
if (this.postalCode!= null) {
products.put("postalCode", this.postalCode.init());
}
if (this.town!= null) {
products.put("town", this.town.init());
}
if (this.inhabitants!= null) {
products.put("inhabitants", this.inhabitants.init());
}
return products;
}
public com.kscs.util.jaxb.Selector> postalCode() {
return ((this.postalCode == null)?this.postalCode = new com.kscs.util.jaxb.Selector<>(this._root, this, "postalCode"):this.postalCode);
}
public com.kscs.util.jaxb.Selector> town() {
return ((this.town == null)?this.town = new com.kscs.util.jaxb.Selector<>(this._root, this, "town"):this.town);
}
public Person.Selector> inhabitants() {
return ((this.inhabitants == null)?this.inhabitants = new Person.Selector<>(this._root, this, "inhabitants"):this.inhabitants);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy