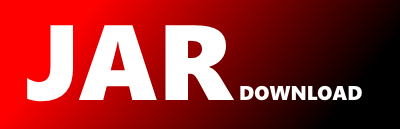
com.kscs.jaxb2.contract.test.CompanyMember Maven / Gradle / Ivy
//
// Diese Datei wurde mit der Eclipse Implementation of JAXB, v4.0.2 generiert
// Siehe https://eclipse-ee4j.github.io/jaxb-ri
// Änderungen an dieser Datei gehen bei einer Neukompilierung des Quellschemas verloren.
//
package com.kscs.jaxb2.contract.test;
import java.util.HashMap;
import java.util.Map;
import javax.xml.namespace.QName;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import com.kscs.util.jaxb.PropertyVisitor;
import com.kscs.util.jaxb.SingleProperty;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlAttribute;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlID;
import jakarta.xml.bind.annotation.XmlRootElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.CollapsedStringAdapter;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
/**
* Java-Klasse für anonymous complex type.
*
*
Das folgende Schemafragment gibt den erwarteten Content an, der in dieser Klasse enthalten ist.
*
*
{@code
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"phone",
"firstName",
"lastName",
"age",
"type"
})
@XmlRootElement(name = "company-member")
public class CompanyMember implements Cloneable, IdentifyingPropertiesLifecycle, UserPropertiesLifecycle
{
@XmlElement(required = true)
protected String phone;
@XmlElement(name = "first-name", required = true)
protected String firstName;
@XmlElement(name = "last-name", required = true)
protected String lastName;
protected int age;
@XmlElement(required = true)
protected String type;
@XmlAttribute(name = "id")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlID
@XmlSchemaType(name = "ID")
protected String id;
@XmlAttribute(name = "unique-name")
protected String uniqueName;
@XmlAttribute(name = "display-name")
protected String displayName;
@XmlAttribute(name = "version")
protected String version;
protected transient CompanyMember.Modifier __cachedModifier__;
/**
* Ruft den Wert der phone-Eigenschaft ab.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPhone() {
return phone;
}
/**
* Legt den Wert der phone-Eigenschaft fest.
*
* @param value
* allowed object is
* {@link String }
*
*/
protected void setPhone(String value) {
this.phone = value;
}
/**
* Ruft den Wert der firstName-Eigenschaft ab.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFirstName() {
return firstName;
}
/**
* Legt den Wert der firstName-Eigenschaft fest.
*
* @param value
* allowed object is
* {@link String }
*
*/
protected void setFirstName(String value) {
this.firstName = value;
}
/**
* Ruft den Wert der lastName-Eigenschaft ab.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLastName() {
return lastName;
}
/**
* Legt den Wert der lastName-Eigenschaft fest.
*
* @param value
* allowed object is
* {@link String }
*
*/
protected void setLastName(String value) {
this.lastName = value;
}
/**
* Ruft den Wert der age-Eigenschaft ab.
*
*/
public int getAge() {
return age;
}
/**
* Legt den Wert der age-Eigenschaft fest.
*
*/
protected void setAge(int value) {
this.age = value;
}
/**
* Ruft den Wert der type-Eigenschaft ab.
*
* @return
* possible object is
* {@link String }
*
*/
public String getType() {
return type;
}
/**
* Legt den Wert der type-Eigenschaft fest.
*
* @param value
* allowed object is
* {@link String }
*
*/
protected void setType(String value) {
this.type = value;
}
/**
* Ruft den Wert der id-Eigenschaft ab.
*
* @return
* possible object is
* {@link String }
*
*/
public String getId() {
return id;
}
/**
* Legt den Wert der id-Eigenschaft fest.
*
* @param value
* allowed object is
* {@link String }
*
*/
protected void setId(String value) {
this.id = value;
}
/**
* Ruft den Wert der uniqueName-Eigenschaft ab.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUniqueName() {
return uniqueName;
}
/**
* Legt den Wert der uniqueName-Eigenschaft fest.
*
* @param value
* allowed object is
* {@link String }
*
*/
protected void setUniqueName(String value) {
this.uniqueName = value;
}
/**
* Ruft den Wert der displayName-Eigenschaft ab.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDisplayName() {
return displayName;
}
/**
* Legt den Wert der displayName-Eigenschaft fest.
*
* @param value
* allowed object is
* {@link String }
*
*/
protected void setDisplayName(String value) {
this.displayName = value;
}
/**
* Ruft den Wert der version-Eigenschaft ab.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVersion() {
if (version == null) {
return "1.1.2";
} else {
return version;
}
}
/**
* Legt den Wert der version-Eigenschaft fest.
*
* @param value
* allowed object is
* {@link String }
*
*/
protected void setVersion(String value) {
this.version = value;
}
@Override
public CompanyMember clone() {
final CompanyMember _newObject;
try {
_newObject = ((CompanyMember) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
return _newObject;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
public<_B >void copyTo(final CompanyMember.Builder<_B> _other) {
_other.phone = this.phone;
_other.firstName = this.firstName;
_other.lastName = this.lastName;
_other.age = this.age;
_other.type = this.type;
_other.id = this.id;
_other.uniqueName = this.uniqueName;
_other.displayName = this.displayName;
_other.version = this.version;
}
public<_B >CompanyMember.Builder<_B> newCopyBuilder(final _B _parentBuilder) {
return new CompanyMember.Builder<_B>(_parentBuilder, this, true);
}
public CompanyMember.Builder newCopyBuilder() {
return newCopyBuilder(null);
}
public static CompanyMember.Builder builder() {
return new CompanyMember.Builder<>(null, null, false);
}
public static<_B >CompanyMember.Builder<_B> copyOf(final CompanyMember _other) {
final CompanyMember.Builder<_B> _newBuilder = new CompanyMember.Builder<>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
public<_B >void copyTo(final CompanyMember.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final PropertyTree phonePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("phone"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(phonePropertyTree!= null):((phonePropertyTree == null)||(!phonePropertyTree.isLeaf())))) {
_other.phone = this.phone;
}
final PropertyTree firstNamePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("firstName"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(firstNamePropertyTree!= null):((firstNamePropertyTree == null)||(!firstNamePropertyTree.isLeaf())))) {
_other.firstName = this.firstName;
}
final PropertyTree lastNamePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("lastName"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(lastNamePropertyTree!= null):((lastNamePropertyTree == null)||(!lastNamePropertyTree.isLeaf())))) {
_other.lastName = this.lastName;
}
final PropertyTree agePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("age"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(agePropertyTree!= null):((agePropertyTree == null)||(!agePropertyTree.isLeaf())))) {
_other.age = this.age;
}
final PropertyTree typePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("type"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(typePropertyTree!= null):((typePropertyTree == null)||(!typePropertyTree.isLeaf())))) {
_other.type = this.type;
}
final PropertyTree idPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("id"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(idPropertyTree!= null):((idPropertyTree == null)||(!idPropertyTree.isLeaf())))) {
_other.id = this.id;
}
final PropertyTree uniqueNamePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("uniqueName"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(uniqueNamePropertyTree!= null):((uniqueNamePropertyTree == null)||(!uniqueNamePropertyTree.isLeaf())))) {
_other.uniqueName = this.uniqueName;
}
final PropertyTree displayNamePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("displayName"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(displayNamePropertyTree!= null):((displayNamePropertyTree == null)||(!displayNamePropertyTree.isLeaf())))) {
_other.displayName = this.displayName;
}
final PropertyTree versionPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("version"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(versionPropertyTree!= null):((versionPropertyTree == null)||(!versionPropertyTree.isLeaf())))) {
_other.version = this.version;
}
}
public<_B >CompanyMember.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return new CompanyMember.Builder<_B>(_parentBuilder, this, true, _propertyTree, _propertyTreeUse);
}
public CompanyMember.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return newCopyBuilder(null, _propertyTree, _propertyTreeUse);
}
public static<_B >CompanyMember.Builder<_B> copyOf(final CompanyMember _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final CompanyMember.Builder<_B> _newBuilder = new CompanyMember.Builder<>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
public static CompanyMember.Builder copyExcept(final CompanyMember _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
public static CompanyMember.Builder copyOnly(final CompanyMember _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
public CompanyMember.Modifier modifier() {
if (null == this.__cachedModifier__) {
this.__cachedModifier__ = new CompanyMember.Modifier();
}
return ((CompanyMember.Modifier) this.__cachedModifier__);
}
public CompanyMember visit(final PropertyVisitor _visitor_) {
_visitor_.visit(this);
_visitor_.visit(new SingleProperty<>(CompanyMember.PropInfo.phone, this));
_visitor_.visit(new SingleProperty<>(CompanyMember.PropInfo.firstName, this));
_visitor_.visit(new SingleProperty<>(CompanyMember.PropInfo.lastName, this));
_visitor_.visit(new SingleProperty<>(CompanyMember.PropInfo.age, this));
_visitor_.visit(new SingleProperty<>(CompanyMember.PropInfo.type, this));
_visitor_.visit(new SingleProperty<>(CompanyMember.PropInfo.id, this));
_visitor_.visit(new SingleProperty<>(CompanyMember.PropInfo.uniqueName, this));
_visitor_.visit(new SingleProperty<>(CompanyMember.PropInfo.displayName, this));
_visitor_.visit(new SingleProperty<>(CompanyMember.PropInfo.version, this));
return this;
}
public static class Builder<_B >implements com.kscs.jaxb2.contract.test.IdentifyingProperties.BuildSupport<_B> , com.kscs.jaxb2.contract.test.UserProperties.BuildSupport<_B> , Buildable
{
protected final _B _parentBuilder;
protected final CompanyMember _storedValue;
private String phone;
private String firstName;
private String lastName;
private int age;
private String type;
private String id;
private String uniqueName;
private String displayName;
private String version = "1.1.2";
public Builder(final _B _parentBuilder, final CompanyMember _other, final boolean _copy) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
this.phone = _other.phone;
this.firstName = _other.firstName;
this.lastName = _other.lastName;
this.age = _other.age;
this.type = _other.type;
this.id = _other.id;
this.uniqueName = _other.uniqueName;
this.displayName = _other.displayName;
this.version = _other.version;
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public Builder(final _B _parentBuilder, final CompanyMember _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
final PropertyTree phonePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("phone"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(phonePropertyTree!= null):((phonePropertyTree == null)||(!phonePropertyTree.isLeaf())))) {
this.phone = _other.phone;
}
final PropertyTree firstNamePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("firstName"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(firstNamePropertyTree!= null):((firstNamePropertyTree == null)||(!firstNamePropertyTree.isLeaf())))) {
this.firstName = _other.firstName;
}
final PropertyTree lastNamePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("lastName"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(lastNamePropertyTree!= null):((lastNamePropertyTree == null)||(!lastNamePropertyTree.isLeaf())))) {
this.lastName = _other.lastName;
}
final PropertyTree agePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("age"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(agePropertyTree!= null):((agePropertyTree == null)||(!agePropertyTree.isLeaf())))) {
this.age = _other.age;
}
final PropertyTree typePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("type"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(typePropertyTree!= null):((typePropertyTree == null)||(!typePropertyTree.isLeaf())))) {
this.type = _other.type;
}
final PropertyTree idPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("id"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(idPropertyTree!= null):((idPropertyTree == null)||(!idPropertyTree.isLeaf())))) {
this.id = _other.id;
}
final PropertyTree uniqueNamePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("uniqueName"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(uniqueNamePropertyTree!= null):((uniqueNamePropertyTree == null)||(!uniqueNamePropertyTree.isLeaf())))) {
this.uniqueName = _other.uniqueName;
}
final PropertyTree displayNamePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("displayName"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(displayNamePropertyTree!= null):((displayNamePropertyTree == null)||(!displayNamePropertyTree.isLeaf())))) {
this.displayName = _other.displayName;
}
final PropertyTree versionPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("version"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(versionPropertyTree!= null):((versionPropertyTree == null)||(!versionPropertyTree.isLeaf())))) {
this.version = _other.version;
}
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public _B end() {
return this._parentBuilder;
}
protected<_P extends CompanyMember >_P init(final _P _product) {
_product.phone = this.phone;
_product.firstName = this.firstName;
_product.lastName = this.lastName;
_product.age = this.age;
_product.type = this.type;
_product.id = this.id;
_product.uniqueName = this.uniqueName;
_product.displayName = this.displayName;
_product.version = this.version;
return _product;
}
/**
* Setzt den neuen Wert der Eigenschaft "phone" (Vorher zugewiesener Wert wird
* ersetzt)
*
* @param phone
* Neuer Wert der Eigenschaft "phone".
*/
public CompanyMember.Builder<_B> withPhone(final String phone) {
this.phone = phone;
return this;
}
/**
* Setzt den neuen Wert der Eigenschaft "firstName" (Vorher zugewiesener Wert wird
* ersetzt)
*
* @param firstName
* Neuer Wert der Eigenschaft "firstName".
*/
public CompanyMember.Builder<_B> withFirstName(final String firstName) {
this.firstName = firstName;
return this;
}
/**
* Setzt den neuen Wert der Eigenschaft "lastName" (Vorher zugewiesener Wert wird
* ersetzt)
*
* @param lastName
* Neuer Wert der Eigenschaft "lastName".
*/
public CompanyMember.Builder<_B> withLastName(final String lastName) {
this.lastName = lastName;
return this;
}
/**
* Setzt den neuen Wert der Eigenschaft "age" (Vorher zugewiesener Wert wird
* ersetzt)
*
* @param age
* Neuer Wert der Eigenschaft "age".
*/
public CompanyMember.Builder<_B> withAge(final int age) {
this.age = age;
return this;
}
/**
* Setzt den neuen Wert der Eigenschaft "type" (Vorher zugewiesener Wert wird
* ersetzt)
*
* @param type
* Neuer Wert der Eigenschaft "type".
*/
public CompanyMember.Builder<_B> withType(final String type) {
this.type = type;
return this;
}
/**
* Setzt den neuen Wert der Eigenschaft "id" (Vorher zugewiesener Wert wird
* ersetzt)
*
* @param id
* Neuer Wert der Eigenschaft "id".
*/
public CompanyMember.Builder<_B> withId(final String id) {
this.id = id;
return this;
}
/**
* Setzt den neuen Wert der Eigenschaft "uniqueName" (Vorher zugewiesener Wert wird
* ersetzt)
*
* @param uniqueName
* Neuer Wert der Eigenschaft "uniqueName".
*/
public CompanyMember.Builder<_B> withUniqueName(final String uniqueName) {
this.uniqueName = uniqueName;
return this;
}
/**
* Setzt den neuen Wert der Eigenschaft "displayName" (Vorher zugewiesener Wert
* wird ersetzt)
*
* @param displayName
* Neuer Wert der Eigenschaft "displayName".
*/
public CompanyMember.Builder<_B> withDisplayName(final String displayName) {
this.displayName = displayName;
return this;
}
/**
* Setzt den neuen Wert der Eigenschaft "version" (Vorher zugewiesener Wert wird
* ersetzt)
*
* @param version
* Neuer Wert der Eigenschaft "version".
*/
public CompanyMember.Builder<_B> withVersion(final String version) {
this.version = version;
return this;
}
@Override
public CompanyMember build() {
if (_storedValue == null) {
return this.init(new CompanyMember());
} else {
return ((CompanyMember) _storedValue);
}
}
public CompanyMember.Builder<_B> copyOf(final CompanyMember _other) {
_other.copyTo(this);
return this;
}
public CompanyMember.Builder<_B> copyOf(final CompanyMember.Builder _other) {
return copyOf(_other.build());
}
}
public class Modifier
implements com.kscs.jaxb2.contract.test.IdentifyingProperties.Modifier, com.kscs.jaxb2.contract.test.UserProperties.Modifier
{
public void setPhone(final String phone) {
CompanyMember.this.setPhone(phone);
}
public void setFirstName(final String firstName) {
CompanyMember.this.setFirstName(firstName);
}
public void setLastName(final String lastName) {
CompanyMember.this.setLastName(lastName);
}
public void setAge(final int age) {
CompanyMember.this.setAge(age);
}
public void setType(final String type) {
CompanyMember.this.setType(type);
}
public void setId(final String id) {
CompanyMember.this.setId(id);
}
public void setUniqueName(final String uniqueName) {
CompanyMember.this.setUniqueName(uniqueName);
}
public void setDisplayName(final String displayName) {
CompanyMember.this.setDisplayName(displayName);
}
public void setVersion(final String version) {
CompanyMember.this.setVersion(version);
}
}
public static class PropInfo {
public static final transient SinglePropertyInfo phone = new SinglePropertyInfo("phone", CompanyMember.class, String.class, false, null, new QName("http://www.kscs.com/jaxb2/contract/test", "phone"), new QName("http://www.w3.org/2001/XMLSchema", "string"), false) {
@Override
public String get(final CompanyMember _instance_) {
return ((_instance_ == null)?null:_instance_.phone);
}
@Override
public void set(final CompanyMember _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.phone = _value_;
}
}
}
;
public static final transient SinglePropertyInfo firstName = new SinglePropertyInfo("firstName", CompanyMember.class, String.class, false, null, new QName("http://www.kscs.com/jaxb2/contract/test", "first-name"), new QName("http://www.w3.org/2001/XMLSchema", "string"), false) {
@Override
public String get(final CompanyMember _instance_) {
return ((_instance_ == null)?null:_instance_.firstName);
}
@Override
public void set(final CompanyMember _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.firstName = _value_;
}
}
}
;
public static final transient SinglePropertyInfo lastName = new SinglePropertyInfo("lastName", CompanyMember.class, String.class, false, null, new QName("http://www.kscs.com/jaxb2/contract/test", "last-name"), new QName("http://www.w3.org/2001/XMLSchema", "string"), false) {
@Override
public String get(final CompanyMember _instance_) {
return ((_instance_ == null)?null:_instance_.lastName);
}
@Override
public void set(final CompanyMember _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.lastName = _value_;
}
}
}
;
public static final transient SinglePropertyInfo age = new SinglePropertyInfo("age", CompanyMember.class, Integer.class, false, null, new QName("http://www.kscs.com/jaxb2/contract/test", "age"), new QName("http://www.w3.org/2001/XMLSchema", "int"), false) {
@Override
public Integer get(final CompanyMember _instance_) {
return ((_instance_ == null)?null:_instance_.age);
}
@Override
public void set(final CompanyMember _instance_, final Integer _value_) {
if (_instance_!= null) {
_instance_.age = _value_;
}
}
}
;
public static final transient SinglePropertyInfo type = new SinglePropertyInfo("type", CompanyMember.class, String.class, false, null, new QName("http://www.kscs.com/jaxb2/contract/test", "type"), new QName("http://www.w3.org/2001/XMLSchema", "string"), false) {
@Override
public String get(final CompanyMember _instance_) {
return ((_instance_ == null)?null:_instance_.type);
}
@Override
public void set(final CompanyMember _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.type = _value_;
}
}
}
;
public static final transient SinglePropertyInfo id = new SinglePropertyInfo("id", CompanyMember.class, String.class, false, null, new QName("", "id"), new QName("http://www.w3.org/2001/XMLSchema", "ID"), true) {
@Override
public String get(final CompanyMember _instance_) {
return ((_instance_ == null)?null:_instance_.id);
}
@Override
public void set(final CompanyMember _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.id = _value_;
}
}
}
;
public static final transient SinglePropertyInfo uniqueName = new SinglePropertyInfo("uniqueName", CompanyMember.class, String.class, false, null, new QName("", "unique-name"), new QName("http://www.w3.org/2001/XMLSchema", "string"), true) {
@Override
public String get(final CompanyMember _instance_) {
return ((_instance_ == null)?null:_instance_.uniqueName);
}
@Override
public void set(final CompanyMember _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.uniqueName = _value_;
}
}
}
;
public static final transient SinglePropertyInfo displayName = new SinglePropertyInfo("displayName", CompanyMember.class, String.class, false, null, new QName("", "display-name"), new QName("http://www.w3.org/2001/XMLSchema", "string"), true) {
@Override
public String get(final CompanyMember _instance_) {
return ((_instance_ == null)?null:_instance_.displayName);
}
@Override
public void set(final CompanyMember _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.displayName = _value_;
}
}
}
;
public static final transient SinglePropertyInfo version = new SinglePropertyInfo("version", CompanyMember.class, String.class, false, "1.1.2", new QName("", "version"), new QName("http://www.w3.org/2001/XMLSchema", "string"), true) {
@Override
public String get(final CompanyMember _instance_) {
return ((_instance_ == null)?null:_instance_.version);
}
@Override
public void set(final CompanyMember _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.version = _value_;
}
}
}
;
}
public static class Select
extends CompanyMember.Selector
{
Select() {
super(null, null, null);
}
public static CompanyMember.Select _root() {
return new CompanyMember.Select();
}
}
public static class Selector , TParent >
extends com.kscs.util.jaxb.Selector
{
private com.kscs.util.jaxb.Selector> phone = null;
private com.kscs.util.jaxb.Selector> firstName = null;
private com.kscs.util.jaxb.Selector> lastName = null;
private com.kscs.util.jaxb.Selector> type = null;
private com.kscs.util.jaxb.Selector> id = null;
private com.kscs.util.jaxb.Selector> uniqueName = null;
private com.kscs.util.jaxb.Selector> displayName = null;
private com.kscs.util.jaxb.Selector> version = null;
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap<>();
products.putAll(super.buildChildren());
if (this.phone!= null) {
products.put("phone", this.phone.init());
}
if (this.firstName!= null) {
products.put("firstName", this.firstName.init());
}
if (this.lastName!= null) {
products.put("lastName", this.lastName.init());
}
if (this.type!= null) {
products.put("type", this.type.init());
}
if (this.id!= null) {
products.put("id", this.id.init());
}
if (this.uniqueName!= null) {
products.put("uniqueName", this.uniqueName.init());
}
if (this.displayName!= null) {
products.put("displayName", this.displayName.init());
}
if (this.version!= null) {
products.put("version", this.version.init());
}
return products;
}
public com.kscs.util.jaxb.Selector> phone() {
return ((this.phone == null)?this.phone = new com.kscs.util.jaxb.Selector<>(this._root, this, "phone"):this.phone);
}
public com.kscs.util.jaxb.Selector> firstName() {
return ((this.firstName == null)?this.firstName = new com.kscs.util.jaxb.Selector<>(this._root, this, "firstName"):this.firstName);
}
public com.kscs.util.jaxb.Selector> lastName() {
return ((this.lastName == null)?this.lastName = new com.kscs.util.jaxb.Selector<>(this._root, this, "lastName"):this.lastName);
}
public com.kscs.util.jaxb.Selector> type() {
return ((this.type == null)?this.type = new com.kscs.util.jaxb.Selector<>(this._root, this, "type"):this.type);
}
public com.kscs.util.jaxb.Selector> id() {
return ((this.id == null)?this.id = new com.kscs.util.jaxb.Selector<>(this._root, this, "id"):this.id);
}
public com.kscs.util.jaxb.Selector> uniqueName() {
return ((this.uniqueName == null)?this.uniqueName = new com.kscs.util.jaxb.Selector<>(this._root, this, "uniqueName"):this.uniqueName);
}
public com.kscs.util.jaxb.Selector> displayName() {
return ((this.displayName == null)?this.displayName = new com.kscs.util.jaxb.Selector<>(this._root, this, "displayName"):this.displayName);
}
public com.kscs.util.jaxb.Selector> version() {
return ((this.version == null)?this.version = new com.kscs.util.jaxb.Selector<>(this._root, this, "version"):this.version);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy