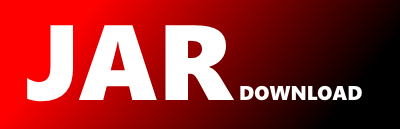
com.kscs.jaxb2.contract.test.Person Maven / Gradle / Ivy
//
// Diese Datei wurde mit der Eclipse Implementation of JAXB, v4.0.2 generiert
// Siehe https://eclipse-ee4j.github.io/jaxb-ri
// Änderungen an dieser Datei gehen bei einer Neukompilierung des Quellschemas verloren.
//
package com.kscs.jaxb2.contract.test;
import java.util.HashMap;
import java.util.Map;
import javax.xml.namespace.QName;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
import com.kscs.util.jaxb.PropertyVisitor;
import com.kscs.util.jaxb.SingleProperty;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import com.kscs.util.jaxb.SinglePropertyInfo;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSeeAlso;
import jakarta.xml.bind.annotation.XmlType;
/**
* Java-Klasse für person complex type.
*
*
Das folgende Schemafragment gibt den erwarteten Content an, der in dieser Klasse enthalten ist.
*
*
{@code
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "person", propOrder = {
"name",
"address",
"phoneNumber"
})
@XmlSeeAlso({
Worker.class
})
public abstract class Person implements Cloneable
{
@XmlElement(required = true)
protected String name;
@XmlElement(required = true)
protected Address address;
@XmlElement(name = "phone-number")
protected long phoneNumber;
protected transient Person.Modifier __cachedModifier__;
/**
* Ruft den Wert der name-Eigenschaft ab.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Legt den Wert der name-Eigenschaft fest.
*
* @param value
* allowed object is
* {@link String }
*
*/
protected void setName(String value) {
this.name = value;
}
/**
* Ruft den Wert der address-Eigenschaft ab.
*
* @return
* possible object is
* {@link Address }
*
*/
public Address getAddress() {
return address;
}
/**
* Legt den Wert der address-Eigenschaft fest.
*
* @param value
* allowed object is
* {@link Address }
*
*/
protected void setAddress(Address value) {
this.address = value;
}
/**
* Ruft den Wert der phoneNumber-Eigenschaft ab.
*
*/
public long getPhoneNumber() {
return phoneNumber;
}
/**
* Legt den Wert der phoneNumber-Eigenschaft fest.
*
*/
protected void setPhoneNumber(long value) {
this.phoneNumber = value;
}
@Override
public Person clone() {
final Person _newObject;
try {
_newObject = ((Person) super.clone());
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
_newObject.address = ((this.address == null)?null:this.address.clone());
return _newObject;
}
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
public<_B >void copyTo(final Person.Builder<_B> _other) {
_other.name = this.name;
_other.address = ((this.address == null)?null:this.address.newCopyBuilder(_other));
_other.phoneNumber = this.phoneNumber;
}
public abstract<_B >Person.Builder<_B> newCopyBuilder(final _B _parentBuilder);
public abstract Person.Builder newCopyBuilder();
/**
* Copies all state of this object to a builder. This method is used by the copyOf
* method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
public<_B >void copyTo(final Person.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final PropertyTree namePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("name"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(namePropertyTree!= null):((namePropertyTree == null)||(!namePropertyTree.isLeaf())))) {
_other.name = this.name;
}
final PropertyTree addressPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("address"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(addressPropertyTree!= null):((addressPropertyTree == null)||(!addressPropertyTree.isLeaf())))) {
_other.address = ((this.address == null)?null:this.address.newCopyBuilder(_other, addressPropertyTree, _propertyTreeUse));
}
final PropertyTree phoneNumberPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("phoneNumber"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(phoneNumberPropertyTree!= null):((phoneNumberPropertyTree == null)||(!phoneNumberPropertyTree.isLeaf())))) {
_other.phoneNumber = this.phoneNumber;
}
}
public abstract<_B >Person.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse);
public abstract Person.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse);
public Person.Modifier modifier() {
if (null == this.__cachedModifier__) {
this.__cachedModifier__ = new Person.Modifier();
}
return ((Person.Modifier) this.__cachedModifier__);
}
public Person visit(final PropertyVisitor _visitor_) {
_visitor_.visit(this);
_visitor_.visit(new SingleProperty<>(Person.PropInfo.name, this));
if (_visitor_.visit(new SingleProperty<>(Person.PropInfo.address, this))&&(this.address!= null)) {
this.address.visit(_visitor_);
}
_visitor_.visit(new SingleProperty<>(Person.PropInfo.phoneNumber, this));
return this;
}
public static class Builder<_B >implements Buildable
{
protected final _B _parentBuilder;
protected final Person _storedValue;
private String name;
private Address.Builder> address;
private long phoneNumber;
public Builder(final _B _parentBuilder, final Person _other, final boolean _copy) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
this.name = _other.name;
this.address = ((_other.address == null)?null:_other.address.newCopyBuilder(this));
this.phoneNumber = _other.phoneNumber;
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public Builder(final _B _parentBuilder, final Person _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
final PropertyTree namePropertyTree = ((_propertyTree == null)?null:_propertyTree.get("name"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(namePropertyTree!= null):((namePropertyTree == null)||(!namePropertyTree.isLeaf())))) {
this.name = _other.name;
}
final PropertyTree addressPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("address"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(addressPropertyTree!= null):((addressPropertyTree == null)||(!addressPropertyTree.isLeaf())))) {
this.address = ((_other.address == null)?null:_other.address.newCopyBuilder(this, addressPropertyTree, _propertyTreeUse));
}
final PropertyTree phoneNumberPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("phoneNumber"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(phoneNumberPropertyTree!= null):((phoneNumberPropertyTree == null)||(!phoneNumberPropertyTree.isLeaf())))) {
this.phoneNumber = _other.phoneNumber;
}
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public _B end() {
return this._parentBuilder;
}
protected<_P extends Person >_P init(final _P _product) {
_product.name = this.name;
_product.address = ((this.address == null)?null:this.address.build());
_product.phoneNumber = this.phoneNumber;
return _product;
}
/**
* Setzt den neuen Wert der Eigenschaft "name" (Vorher zugewiesener Wert wird
* ersetzt)
*
* @param name
* Neuer Wert der Eigenschaft "name".
*/
public Person.Builder<_B> withName(final String name) {
this.name = name;
return this;
}
/**
* Setzt den neuen Wert der Eigenschaft "address" (Vorher zugewiesener Wert wird
* ersetzt)
*
* @param address
* Neuer Wert der Eigenschaft "address".
*/
public Person.Builder<_B> withAddress(final Address address) {
this.address = ((address == null)?null:new Address.Builder<>(this, address, false));
return this;
}
/**
* Erzeugt den vorhandenen Builder oder einen neuen "Builder" zum Zusammenbauen des
* Wertes der Eigenschaft "address".
* Mit {@link com.kscs.jaxb2.contract.test.Address.Builder#end()} geht es zurück
* zum aktuellen Builder.
*
* @return
* Ein neuer "Builder" zum Zusammenbauen des Wertes der Eigenschaft "address".
* Mit {@link com.kscs.jaxb2.contract.test.Address.Builder#end()} geht es zurück
* zum aktuellen Builder.
*/
public Address.Builder extends Person.Builder<_B>> withAddress() {
if (this.address!= null) {
return this.address;
}
return this.address = new Address.Builder<>(this, null, false);
}
/**
* Setzt den neuen Wert der Eigenschaft "phoneNumber" (Vorher zugewiesener Wert
* wird ersetzt)
*
* @param phoneNumber
* Neuer Wert der Eigenschaft "phoneNumber".
*/
public Person.Builder<_B> withPhoneNumber(final long phoneNumber) {
this.phoneNumber = phoneNumber;
return this;
}
@Override
public Person build() {
return ((Person) _storedValue);
}
public Person.Builder<_B> copyOf(final Person _other) {
_other.copyTo(this);
return this;
}
public Person.Builder<_B> copyOf(final Person.Builder _other) {
return copyOf(_other.build());
}
}
public class Modifier {
public void setName(final String name) {
Person.this.setName(name);
}
public void setAddress(final Address address) {
Person.this.setAddress(address);
}
public void setPhoneNumber(final long phoneNumber) {
Person.this.setPhoneNumber(phoneNumber);
}
}
public static class PropInfo {
public static final transient SinglePropertyInfo name = new SinglePropertyInfo("name", Person.class, String.class, false, null, new QName("http://www.kscs.com/jaxb2/contract/test", "name"), new QName("http://www.w3.org/2001/XMLSchema", "string"), false) {
@Override
public String get(final Person _instance_) {
return ((_instance_ == null)?null:_instance_.name);
}
@Override
public void set(final Person _instance_, final String _value_) {
if (_instance_!= null) {
_instance_.name = _value_;
}
}
}
;
public static final transient SinglePropertyInfo address = new SinglePropertyInfo("address", Person.class, Address.class, false, null, new QName("http://www.kscs.com/jaxb2/contract/test", "address"), new QName("http://www.kscs.com/jaxb2/contract/test", "anononymousElementType"), false) {
@Override
public Address get(final Person _instance_) {
return ((_instance_ == null)?null:_instance_.address);
}
@Override
public void set(final Person _instance_, final Address _value_) {
if (_instance_!= null) {
_instance_.address = _value_;
}
}
}
;
public static final transient SinglePropertyInfo phoneNumber = new SinglePropertyInfo("phoneNumber", Person.class, Long.class, false, null, new QName("http://www.kscs.com/jaxb2/contract/test", "phone-number"), new QName("http://www.w3.org/2001/XMLSchema", "long"), false) {
@Override
public Long get(final Person _instance_) {
return ((_instance_ == null)?null:_instance_.phoneNumber);
}
@Override
public void set(final Person _instance_, final Long _value_) {
if (_instance_!= null) {
_instance_.phoneNumber = _value_;
}
}
}
;
}
public static class Select
extends Person.Selector
{
Select() {
super(null, null, null);
}
public static Person.Select _root() {
return new Person.Select();
}
}
public static class Selector , TParent >
extends com.kscs.util.jaxb.Selector
{
private com.kscs.util.jaxb.Selector> name = null;
private Address.Selector> address = null;
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap<>();
products.putAll(super.buildChildren());
if (this.name!= null) {
products.put("name", this.name.init());
}
if (this.address!= null) {
products.put("address", this.address.init());
}
return products;
}
public com.kscs.util.jaxb.Selector> name() {
return ((this.name == null)?this.name = new com.kscs.util.jaxb.Selector<>(this._root, this, "name"):this.name);
}
public Address.Selector> address() {
return ((this.address == null)?this.address = new Address.Selector<>(this._root, this, "address"):this.address);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy