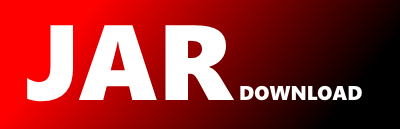
colesico.framework.dslvalidator.builder.FlowControlBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of colesico-validation Show documentation
Show all versions of colesico-validation Show documentation
Colesico framework data bean validation assistant and simple dsl validator
/*
* Copyright © 2014-2020 Vladlen V. Larionov and others as noted.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package colesico.framework.dslvalidator.builder;
import colesico.framework.dslvalidator.Command;
import colesico.framework.dslvalidator.DSLValidator;
import colesico.framework.dslvalidator.commands.*;
import colesico.framework.translation.Translatable;
import java.util.List;
import java.util.Map;
import java.util.function.Function;
abstract public class FlowControlBuilder {
/**
* Defines validation algorithm
*
* @param commands
* @return
*/
protected final DSLValidator program(final Command... commands) {
GroupSequence sequence = new GroupSequence<>();
for (Command cmd : commands) {
sequence.addCommand(cmd);
}
return new DSLValidator<>(sequence, null);
}
protected final DSLValidator program(final String subject, final Command... commands) {
ChainSequence sequence = new ChainSequence<>();
for (Command cmd : commands) {
sequence.addCommand(cmd);
}
return new DSLValidator<>(sequence, subject);
}
/**
* Executes commands within the local context.
* In case of local validation errors occur, command execution is NOT interrupted.
*
* @param commands
* @return
* @see GroupSequence
*/
protected final Command group(final Command... commands) {
GroupSequence sequence = new GroupSequence<>();
for (Command cmd : commands) {
sequence.addCommand(cmd);
}
return sequence;
}
/**
* Executes commands within the local context.
* In case of local validation errors occur, command execution is interrupted.
*
* @param commands
* @return
*/
protected final Command chain(final Command... commands) {
ChainSequence sequence = new ChainSequence<>();
for (Command cmd : commands) {
sequence.addCommand(cmd);
}
return sequence;
}
/**
* Executes commands within the new nested context with specified subject.
* In case of local validation errors occur, commands execution is interrupted.
*
* @param subject
* @param commands
* @return
* @see SubjectSequence
*/
protected final Command subject(final String subject, final Command... commands) {
SubjectSequence sequence = new SubjectSequence<>(subject);
for (Command cmd : commands) {
sequence.addCommand(cmd);
}
return sequence;
}
/**
* Creates new nested context with the subject and the value, extracted from the value of the local context.
* Execute commands within that nested context.
* In case of validation errors occur in the nested context, command execution is interrupted.
*
* @param subject
* @param extractor
* @param commands
* @return
* @see FieldSequence
*/
protected final Command field(final String subject, final Function extractor, final Command... commands) {
FieldSequence sequence = new FieldSequence<>(subject, extractor);
for (Command cmd : commands) {
sequence.addCommand(cmd);
}
return sequence;
}
/**
* In case of local validation errors occur, command execution is interrupted.
*
* @param subject
* @param index
* @param commands
* @return
*/
protected final , E> Command item(final String subject, final int index, final Command... commands) {
ListSequence sequence = new ListSequence<>(subject, index);
for (Command cmd : commands) {
sequence.addCommand(cmd);
}
return sequence;
}
protected final , K, E> Command entry(final String subject, final K key, final Command... commands) {
MapSequence sequence = new MapSequence<>(subject, key);
for (Command cmd : commands) {
sequence.addCommand(cmd);
}
return sequence;
}
/**
* Iterates the elements of value from local context.
* In case of local validation errors occur, command execution is interrupted.
*
* @param
* @return
* @see IterableSequence
*/
protected final , I> Command forEach(final Command... commands) {
IterableSequence sequence = new IterableSequence<>();
for (Command cmd : commands) {
sequence.addCommand(cmd);
}
return sequence;
}
protected final Command ifNotNull(final Command... commands) {
IfNotNullSequence sequence = new IfNotNullSequence<>();
for (Command cmd : commands) {
sequence.addCommand(cmd);
}
return sequence;
}
protected final Command hookError(String errorCode, Translatable errorMsg, final Command... commands) {
HookErrorSequence sequence = new HookErrorSequence<>(errorCode, errorMsg);
for (Command cmd : commands) {
sequence.addCommand(cmd);
}
return sequence;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy