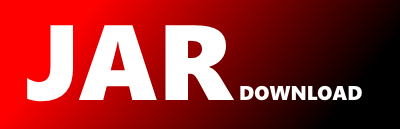
colesico.framework.weblet.internal.WebletIoclet Maven / Gradle / Ivy
// This is automatically generated file. Do not modify!
package colesico.framework.weblet.internal;
import colesico.framework.assist.LazySingleton;
import colesico.framework.assist.codegen.Genstamp;
import colesico.framework.http.HttpContext;
import colesico.framework.ioc.ioclet.AdvancedIoc;
import colesico.framework.ioc.ioclet.Catalog;
import colesico.framework.ioc.ioclet.DefaultProvider;
import colesico.framework.ioc.ioclet.Factory;
import colesico.framework.ioc.ioclet.Ioclet;
import colesico.framework.ioc.ioclet.SingletonFactory;
import colesico.framework.ioc.key.NamedKey;
import colesico.framework.ioc.key.TypeKey;
import colesico.framework.ioc.production.InstanceProducingException;
import colesico.framework.ioc.scope.ThreadScope;
import colesico.framework.router.RouterContext;
import colesico.framework.teleapi.TRWFactory;
import colesico.framework.telehttp.Origin;
import colesico.framework.telehttp.assist.CSRFProtector;
import colesico.framework.weblet.teleapi.Authenticator;
import colesico.framework.weblet.teleapi.WebletDataPort;
import colesico.framework.weblet.teleapi.WebletTeleDriver;
import colesico.framework.weblet.teleapi.origin.WebletAutoOrigin;
@Genstamp(
generator = "colesico.framework.ioc.codegen.generator.IocletGenerator",
timestamp = "2022-10-25T11:36:40.051Z",
hashId = "3d494aad-bf7c-4945-b5b2-6dcadc0742fd",
comments = "Producer: colesico.framework.weblet.internal.WebletProducer"
)
public final class WebletIoclet implements Ioclet {
private final LazySingleton producer = new LazySingleton() {
@Override
public final WebletProducer create() {
return new WebletProducer();
}
};
@Override
public final String getId() {
return "colesico.framework.weblet.internal.WebletProducer";
}
/**
* Factory to produce colesico.framework.weblet.teleapi.WebletDataPort class instance
* Scope: SINGLETON; Custom: null
*/
public Factory getWebletDataPortFactory0() {
return new SingletonFactory() {
private Factory implFac;
@Override
public final void setup(final AdvancedIoc ioc) {
// Initialize dependencies for: colesico.framework.weblet.teleapi.WebletDataPort
this.implFac = ioc.factory(new TypeKey("colesico.framework.weblet.internal.WebletDataPortImpl"));
}
@Override
public final WebletDataPort create(final Object message) {
try {
// Perform instance producing
WebletDataPort instance = producer.get().getWebletDataPort(this.implFac.get(message));
return instance;
} catch (InstanceProducingException ipe) {
throw ipe;
} catch (Throwable t) {
throw new InstanceProducingException(t, WebletDataPort.class);
}
}
};
}
/**
* Factory to produce colesico.framework.weblet.teleapi.WebletTeleDriver class instance
* Scope: SINGLETON; Custom: null
*/
public Factory getWebTeledriverFactory1() {
return new SingletonFactory() {
private Factory implFac;
@Override
public final void setup(final AdvancedIoc ioc) {
// Initialize dependencies for: colesico.framework.weblet.teleapi.WebletTeleDriver
this.implFac = ioc.factory(new TypeKey("colesico.framework.weblet.internal.WebletTeleDriverImpl"));
}
@Override
public final WebletTeleDriver create(final Object message) {
try {
// Perform instance producing
WebletTeleDriver instance = producer.get().getWebTeledriver(this.implFac.get(message));
return instance;
} catch (InstanceProducingException ipe) {
throw ipe;
} catch (Throwable t) {
throw new InstanceProducingException(t, WebletTeleDriver.class);
}
}
};
}
/**
* Factory to produce colesico.framework.weblet.teleapi.Authenticator class instance
* Scope: SINGLETON; Custom: null
*/
public Factory getAuthenticatorFactory2() {
return new SingletonFactory() {
private Factory implFac;
@Override
public final void setup(final AdvancedIoc ioc) {
// Initialize dependencies for: colesico.framework.weblet.teleapi.Authenticator
this.implFac = ioc.factory(new TypeKey("colesico.framework.weblet.internal.AuthenticatorImpl"));
}
@Override
public final Authenticator create(final Object message) {
try {
// Perform instance producing
Authenticator instance = producer.get().getAuthenticator(this.implFac.get(message));
return instance;
} catch (InstanceProducingException ipe) {
throw ipe;
} catch (Throwable t) {
throw new InstanceProducingException(t, Authenticator.class);
}
}
};
}
/**
* Factory to produce colesico.framework.telehttp.Origin class instance
* Scope: SINGLETON; Custom: null
* Named: WEBLET_AUTO
*/
public Factory getWebletAutoOriginFactory3() {
return new SingletonFactory() {
private Factory implFac;
@Override
public final void setup(final AdvancedIoc ioc) {
// Initialize dependencies for: colesico.framework.telehttp.Origin
this.implFac = ioc.factory(new TypeKey("colesico.framework.weblet.teleapi.origin.WebletAutoOrigin"));
}
@Override
public final Origin create(final Object message) {
try {
// Perform instance producing
Origin instance = producer.get().getWebletAutoOrigin(this.implFac.get(message));
return instance;
} catch (InstanceProducingException ipe) {
throw ipe;
} catch (Throwable t) {
throw new InstanceProducingException(t, Origin.class);
}
}
};
}
/**
* Factory to produce colesico.framework.weblet.internal.WebletDataPortImpl class instance
* Scope: SINGLETON; Custom: null
*/
public Factory getWebletDataPortImplFactory4() {
return new SingletonFactory() {
private Factory trwFactoryFac;
@Override
public final void setup(final AdvancedIoc ioc) {
// Initialize dependencies for: colesico.framework.weblet.internal.WebletDataPortImpl
this.trwFactoryFac = ioc.factory(new TypeKey("colesico.framework.teleapi.TRWFactory"));
}
@Override
public final WebletDataPortImpl create(final Object message) {
try {
// Perform instance producing
WebletDataPortImpl instance = new WebletDataPortImpl(this.trwFactoryFac.get(message));
// Post construct listeners invocations:
return instance;
} catch (InstanceProducingException ipe) {
throw ipe;
} catch (Throwable t) {
throw new InstanceProducingException(t, WebletDataPortImpl.class);
}
}
};
}
/**
* Factory to produce colesico.framework.weblet.internal.WebletTeleDriverImpl class instance
* Scope: SINGLETON; Custom: null
*/
public Factory getWebletTeleDriverImplFactory5() {
return new SingletonFactory() {
private Factory threadScopeFac;
private Factory dataPortFac;
private Factory authenticatorProvFac;
private Factory httpContextProvFac;
private Factory routerContextProvFac;
private Factory csrfProtectorFac;
@Override
public final void setup(final AdvancedIoc ioc) {
// Initialize dependencies for: colesico.framework.weblet.internal.WebletTeleDriverImpl
this.threadScopeFac = ioc.factory(new TypeKey("colesico.framework.ioc.scope.ThreadScope"));
this.dataPortFac = ioc.factory(new TypeKey("colesico.framework.weblet.internal.WebletDataPortImpl"));
this.authenticatorProvFac = ioc.factory(new TypeKey("colesico.framework.weblet.teleapi.Authenticator"));
this.httpContextProvFac = ioc.factory(new TypeKey("colesico.framework.http.HttpContext"));
this.routerContextProvFac = ioc.factory(new TypeKey("colesico.framework.router.RouterContext"));
this.csrfProtectorFac = ioc.factory(new TypeKey("colesico.framework.telehttp.assist.CSRFProtector"));
}
@Override
public final WebletTeleDriverImpl create(final Object message) {
try {
// Perform instance producing
WebletTeleDriverImpl instance = new WebletTeleDriverImpl(this.threadScopeFac.get(message),this.dataPortFac.get(message),new DefaultProvider(this.authenticatorProvFac,message),new DefaultProvider(this.httpContextProvFac,message),new DefaultProvider(this.routerContextProvFac,message),this.csrfProtectorFac.get(message));
// Post construct listeners invocations:
return instance;
} catch (InstanceProducingException ipe) {
throw ipe;
} catch (Throwable t) {
throw new InstanceProducingException(t, WebletTeleDriverImpl.class);
}
}
};
}
/**
* Factory to produce colesico.framework.weblet.internal.AuthenticatorImpl class instance
* Scope: UNSCOPED; Custom: null
*/
public Factory getAuthenticatorImplFactory6() {
return new Factory() {
@Override
public final void setup(final AdvancedIoc ioc) {
// Initialize dependencies for: colesico.framework.weblet.internal.AuthenticatorImpl
}
@Override
public final AuthenticatorImpl get(final Object message) {
try {
// Perform instance producing
AuthenticatorImpl instance = new AuthenticatorImpl();
// Post construct listeners invocations:
return instance;
} catch (InstanceProducingException ipe) {
throw ipe;
} catch (Throwable t) {
throw new InstanceProducingException(t, AuthenticatorImpl.class);
}
}
};
}
/**
* Factory to produce colesico.framework.weblet.teleapi.origin.WebletAutoOrigin class instance
* Scope: SINGLETON; Custom: null
*/
public Factory getWebletAutoOriginFactory7() {
return new SingletonFactory() {
private Factory httpContextProvFac;
private Factory routerContextProvFac;
@Override
public final void setup(final AdvancedIoc ioc) {
// Initialize dependencies for: colesico.framework.weblet.teleapi.origin.WebletAutoOrigin
this.httpContextProvFac = ioc.factory(new TypeKey("colesico.framework.http.HttpContext"));
this.routerContextProvFac = ioc.factory(new TypeKey("colesico.framework.router.RouterContext"));
}
@Override
public final WebletAutoOrigin create(final Object message) {
try {
// Perform instance producing
WebletAutoOrigin instance = new WebletAutoOrigin(new DefaultProvider(this.httpContextProvFac,message),new DefaultProvider(this.routerContextProvFac,message));
// Post construct listeners invocations:
return instance;
} catch (InstanceProducingException ipe) {
throw ipe;
} catch (Throwable t) {
throw new InstanceProducingException(t, WebletAutoOrigin.class);
}
}
};
}
@Override
public final void addFactories(final Catalog catalog) {
if(catalog.accept(new TypeKey("colesico.framework.weblet.teleapi.WebletDataPort"), null, null, false)){
catalog.add(getWebletDataPortFactory0());
}
if(catalog.accept(new TypeKey("colesico.framework.weblet.teleapi.WebletTeleDriver"), null, null, false)){
catalog.add(getWebTeledriverFactory1());
}
if(catalog.accept(new TypeKey("colesico.framework.weblet.teleapi.Authenticator"), null, null, false)){
catalog.add(getAuthenticatorFactory2());
}
if(catalog.accept(new NamedKey("colesico.framework.telehttp.Origin","WEBLET_AUTO"), null, null, false)){
catalog.add(getWebletAutoOriginFactory3());
}
if(catalog.accept(new TypeKey("colesico.framework.weblet.internal.WebletDataPortImpl"), null, null, false)){
catalog.add(getWebletDataPortImplFactory4());
}
if(catalog.accept(new TypeKey("colesico.framework.weblet.internal.WebletTeleDriverImpl"), null, null, false)){
catalog.add(getWebletTeleDriverImplFactory5());
}
if(catalog.accept(new TypeKey("colesico.framework.weblet.internal.AuthenticatorImpl"), null, null, false)){
catalog.add(getAuthenticatorImplFactory6());
}
if(catalog.accept(new TypeKey("colesico.framework.weblet.teleapi.origin.WebletAutoOrigin"), null, null, false)){
catalog.add(getWebletAutoOriginFactory7());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy