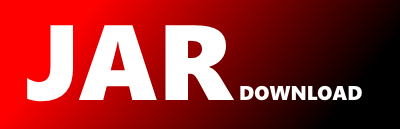
com.quorum.tessera.partyinfo.node.NodeInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tessera-partyinfo Show documentation
Show all versions of tessera-partyinfo Show documentation
Tessera is a stateless Java system that is used to enable the encryption, decryption, and distribution of private transactions for Quorum.
package com.quorum.tessera.partyinfo.node;
import com.quorum.tessera.encryption.PublicKey;
import java.util.*;
import java.util.stream.Collectors;
public interface NodeInfo {
default Map getRecipientsAsMap() {
return getRecipients().stream().collect(Collectors.toUnmodifiableMap(Recipient::getKey, Recipient::getUrl));
}
Set getRecipients();
Set supportedApiVersions();
String getUrl();
class Builder {
private String url;
private final Set recipients = new HashSet<>();
private Set supportedApiVersions = new HashSet<>();
public Builder withRecipients(Collection recipients) {
this.recipients.addAll(recipients);
return this;
}
public Builder withSupportedApiVersions(Collection supportedApiVersions) {
if (Objects.nonNull(supportedApiVersions)) {
this.supportedApiVersions = Set.copyOf(supportedApiVersions);
}
return this;
}
public NodeInfo build() {
Objects.requireNonNull(url, "URL is required");
return new NodeInfo() {
@Override
public Set getRecipients() {
return Set.copyOf(recipients);
}
@Override
public Set supportedApiVersions() {
return Set.copyOf(supportedApiVersions);
}
@Override
public String getUrl() {
return url;
}
@Override
public String toString() {
return String.format("NodeInfo[url: %s ,recipients: %s]", url, recipients);
}
};
}
public static Builder create() {
return new Builder() {};
}
private Builder() {}
public Builder withUrl(String url) {
this.url = url;
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy