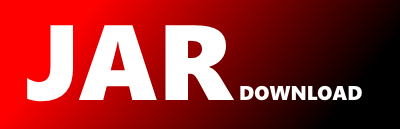
com.quorum.tessera.discovery.ActiveNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tessera-partyinfo Show documentation
Show all versions of tessera-partyinfo Show documentation
Tessera is a stateless Java system that is used to enable the encryption, decryption, and distribution of private transactions for Quorum.
package com.quorum.tessera.discovery;
import com.quorum.tessera.encryption.PublicKey;
import java.util.Collection;
import java.util.List;
import java.util.Objects;
import java.util.Set;
public class ActiveNode {
private final Set keys;
private final NodeUri uri;
private final Set supportedVersions;
public Set getKeys() {
return keys;
}
public NodeUri getUri() {
return uri;
}
public Set getSupportedVersions() {
return supportedVersions;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ActiveNode that = (ActiveNode) o;
return uri.equals(that.uri);
}
@Override
public int hashCode() {
return Objects.hash(uri);
}
@Override
public String toString() {
return "ActiveNode{" +
"keys=" + keys +
", uri=" + uri +
", supportedVersions=" + supportedVersions +
'}';
}
private ActiveNode(NodeUri uri, Set keys, Set supportedVersions) {
this.keys = keys;
this.uri = uri;
this.supportedVersions = supportedVersions;
}
public static class Builder {
private NodeUri nodeUri;
private List keys = List.of();
private List supportedVersions = List.of();
public static Builder create() {
return new Builder();
}
public static Builder from(ActiveNode activeNode) {
return create()
.withUri(activeNode.getUri())
.withSupportedVersions(activeNode.getSupportedVersions())
.withKeys(activeNode.getKeys());
}
private Builder() {
}
public Builder withUri(NodeUri nodeUri) {
this.nodeUri = nodeUri;
return this;
}
public Builder withKeys(Collection keys) {
this.keys = List.copyOf(keys);
return this;
}
public Builder withSupportedVersions(Collection supportedVersions) {
this.supportedVersions = List.copyOf(supportedVersions);
return this;
}
public ActiveNode build() {
Objects.requireNonNull(nodeUri,"Node uri is required");
Objects.requireNonNull(keys,"keys are required");
return new ActiveNode(nodeUri,Set.copyOf(keys),Set.copyOf(supportedVersions));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy