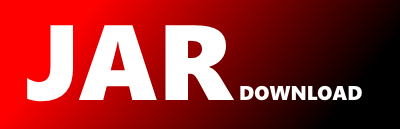
com.quorum.tessera.recovery.workflow.EncodedPayloadPublisher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tessera-recover Show documentation
Show all versions of tessera-recover Show documentation
Tessera is a stateless Java system that is used to enable the encryption, decryption, and distribution of private transactions for Quorum.
package com.quorum.tessera.recovery.workflow;
import com.quorum.tessera.enclave.EncodedPayload;
import com.quorum.tessera.recovery.resend.ResendBatchPublisher;
import java.util.ArrayList;
import java.util.List;
import java.util.Set;
import java.util.stream.Collectors;
public class EncodedPayloadPublisher implements BatchWorkflowAction {
private String targetUrl;
private final List> payloads;
private final ResendBatchPublisher resendBatchPublisher;
private long messageCounter = 0L;
public EncodedPayloadPublisher(ResendBatchPublisher resendBatchPublisher) {
this.resendBatchPublisher = resendBatchPublisher;
this.payloads = new ArrayList<>();
}
@Override
public boolean execute(BatchWorkflowContext event) {
final int batchSize = event.getBatchSize();
final long total = event.getExpectedTotal();
targetUrl = event.getRecipient().getUrl();
payloads.add(event.getPayloadsToPublish());
if (payloads.size() == batchSize || messageCounter + payloads.size() >= total) {
publish(batchSize);
}
return true;
}
public long getPublishedCount() {
return messageCounter;
}
public void checkOutstandingPayloads(BatchWorkflowContext event) {
final long total = event.getExpectedTotal();
final int noOfPayloads = payloads.size();
if (noOfPayloads > 0 && (messageCounter + noOfPayloads >= total)) {
publish(event.getBatchSize());
}
}
private void publish(final int batchSize) {
List allPayloads =
this.payloads.stream().flatMap(Set::stream).collect(Collectors.toList());
// need to split the payloads into sublists with at most batchSize,
// the publish each list individually
while (allPayloads.size() > batchSize) {
final List sublistPayloads =
new ArrayList<>(allPayloads.subList(0, batchSize));
resendBatchPublisher.publishBatch(sublistPayloads, targetUrl);
allPayloads = allPayloads.subList(batchSize, allPayloads.size());
}
// one final push for the last batch
resendBatchPublisher.publishBatch(allPayloads, targetUrl);
messageCounter += payloads.size();
payloads.clear();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy