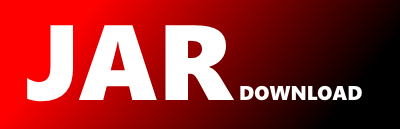
net.craftforge.essential.context.basic.BasicResponse Maven / Gradle / Ivy
The newest version!
/*
* This file is part of essential.
*
* essential is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* essential is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with essential. If not, see .
*/
package net.craftforge.essential.context.basic;
import net.craftforge.essential.context.Response;
import net.craftforge.essential.core.constants.Charsets;
import net.craftforge.essential.core.constants.HttpStatusCode;
import java.io.BufferedWriter;
import java.io.IOException;
import java.io.OutputStream;
import java.io.OutputStreamWriter;
import java.util.Map;
/**
* A basic implementation of a response.
*
* @author Christian Bick
* @since 20.06.11
*/
public class BasicResponse implements Response {
private HttpStatusCode httpStatusCode;
private Map headers;
private OutputStream outputStream;
public BasicResponse(OutputStream outputStream) {
this.outputStream = outputStream;
}
/**
* {@inheritDoc}
*/
public void sendHeaders(HttpStatusCode httpStatusCode, Map headers) {
this.httpStatusCode = httpStatusCode;
this.headers = headers;
BufferedWriter writer;
try{
writer = new BufferedWriter(new OutputStreamWriter(outputStream, Charsets.UTF8));
writer.write("HTTP/1.1 " + httpStatusCode.getCode() + " " + httpStatusCode.getName());
writer.newLine();
for (String headerName : headers.keySet()) {
for (String headerValue : headers.get(headerName)) {
writer.write(headerName + ": " + headerValue);
writer.newLine();
}
}
writer.flush();
} catch (IOException e) {
throw new RuntimeException("Unable to send response headers", e);
}
}
/**
* {@inheritDoc}
*/
public HttpStatusCode getStatusCode() {
return httpStatusCode;
}
/**
* {@inheritDoc}
*/
public Map getHeaders() {
return headers;
}
/**
* {@inheritDoc}
*/
public OutputStream getBodyOutputStream() {
return outputStream;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy