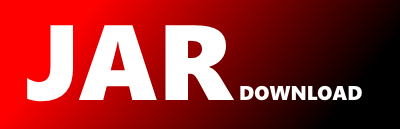
net.craftforge.essential.controller.ControllerConfiguration Maven / Gradle / Ivy
The newest version!
/*
* This file is part of essential.
*
* essential is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* essential is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with essential. If not, see .
*/
package net.craftforge.essential.controller;
import net.craftforge.commons.configuration.PropertiesHolder;
import net.craftforge.essential.controller.phases.flow.DefaultPhaseFlow;
import net.craftforge.essential.supply.suppliers.basic.BasicConsumer;
import net.craftforge.essential.supply.suppliers.basic.BasicProducer;
import net.craftforge.essential.supply.suppliers.jackson.JacksonConsumer;
import net.craftforge.essential.supply.suppliers.jackson.JacksonProducer;
import net.craftforge.essential.supply.suppliers.jaxb.JaxbConsumer;
import net.craftforge.essential.supply.suppliers.jaxb.JaxbProducer;
import javax.inject.Singleton;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
/**
* A controller configuration provides configuration
* for a controller.
*
* @author Christian Bick
* @since 03.03.2011
*/
@Singleton
public class ControllerConfiguration extends PropertiesHolder {
/**
* The default configuration file
*/
public static final String DEFAULT_CONFIG_FILE = "essential-controller.properties";
public static final String PROPERTY_DEFAULT_CONSUMER = "controller.supply.consumers";
public static final String PROPERTY_DEFAULT_PRODUCER = "controller.supply.producers";
public static final String PROPERTY_PHASE_FLOW = "controller.phases.flow";
public static final String PROPERTY_AUTHENTICATION_HANDLER = "controller.handlers.authentication";
public static final Class> DEFAULT_PHASE_FLOW = DefaultPhaseFlow.class;
public static final List> DEFAULT_CONSUMERS;
public static final List> DEFAULT_PRODUCERS;
static {
List> defaultConsumers = new ArrayList>(3);
defaultConsumers.add(BasicConsumer.class);
defaultConsumers.add(JaxbConsumer.class);
defaultConsumers.add(JacksonConsumer.class);
DEFAULT_CONSUMERS = Collections.unmodifiableList(defaultConsumers);
List> defaultProducers = new ArrayList>(3);
defaultProducers.add(BasicProducer.class);
defaultProducers.add(JaxbProducer.class);
defaultProducers.add(JacksonProducer.class);
DEFAULT_PRODUCERS = Collections.unmodifiableList(defaultProducers);
}
/**
* Initializes a configuration using the default configuration file.
*/
public ControllerConfiguration() {
this(DEFAULT_CONFIG_FILE);
}
/**
* Initializes a configuration using the given configuration file.
*
* @param configFile The configuration file
*/
public ControllerConfiguration(String configFile) {
super("/" + configFile);
}
/**
* Gets the class of the default consumer.
*
* @return The class of the default consumer
*/
public List> getDefaultConsumerClasses() {
return getClasses(PROPERTY_DEFAULT_CONSUMER, DEFAULT_CONSUMERS);
}
/**
* Gets the class of the default producer.
*
* @return The class of the default producer.
*/
public List> getDefaultProducerClasses() {
return getClasses(PROPERTY_DEFAULT_PRODUCER, DEFAULT_PRODUCERS);
}
/**
* Gets the class of the phase flow defining the controller's phase execution logic.
*
* @return The class of the phase flow
*/
public Class> getPhaseFlowClass() {
return getClass(PROPERTY_PHASE_FLOW, DEFAULT_PHASE_FLOW);
}
/**
* Gets the class of the authentication handler.
*
* @return The class of the authentication handler
*/
public Class> getAuthenticationHandler() {
return getClass(PROPERTY_AUTHENTICATION_HANDLER, null);
}
/**
* Sets the class of the authentication handler. If no authentication handler is
* specified, the authentication phase will be skipped.
*
* @param authenticationHandler The class of the authentication handler
*/
public void setAuthenticationHandler(Class> authenticationHandler) {
setProperty(PROPERTY_AUTHENTICATION_HANDLER, authenticationHandler.getName());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy