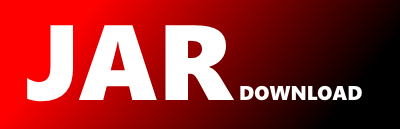
net.craftforge.essential.controller.managers.ResourceManager Maven / Gradle / Ivy
The newest version!
/*
* This file is part of essential.
*
* essential is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* essential is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with essential. If not, see .
*/
package net.craftforge.essential.controller.managers;
import net.craftforge.essential.controller.allocation.ResourceNode;
import net.craftforge.essential.controller.allocation.ResourceTree;
import net.craftforge.essential.controller.utils.ControllerReflUtils;
import net.craftforge.essential.controller.utils.ControllerRegExUtils;
import net.craftforge.essential.core.utils.UriUtils;
import net.craftforge.reflection.utils.ClassUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.inject.Inject;
import java.lang.reflect.Method;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* A resource manager takes care of matching resource URLs against resource classes and methods.
*
* @author Christian Bick
* @since 02.02.2011
*/
public class ResourceManager {
private static final Logger LOGGER = LoggerFactory.getLogger(ResourceManager.class);
private ResourceTree resourceTree;
@Inject
public ResourceManager(ResourceTree resourceTree) {
this.resourceTree = resourceTree;
}
/**
* Gets the request path parameter map corresponding to the request path.
*
* @param requestPath The request requestPath
* @return The request requestPath parameter map
*/
public Map getPathParameters(String requestPath) {
String[] requestPathParts = UriUtils.splitPath(requestPath);
Map pathParameterMap = new HashMap(requestPathParts.length);
List resourceNodes = resourceTree.findResourceNodesAlongPath(requestPath);
for (ResourceNode resourceNode : resourceNodes) {
String pathPart = resourceNode.getPathPart();
if (ControllerRegExUtils.FIND_ENCLOSED_BY_CURLY_BRACKETS.matcher(pathPart).matches()) {
String requestPathPart = requestPathParts[resourceNode.getLevel()];
String key = ControllerRegExUtils.getParamNameFromPathPart(resourceNode.getPathPart());
String[] values = ControllerRegExUtils.getValuesFromPathPart(requestPathPart);
pathParameterMap.put(key, values );
}
}
return pathParameterMap;
}
/**
* Gets the resource class corresponding to the request path.
*
* @param path The request path
* @return The resource class
*/
public Class> getResourceClass(String path) {
ResourceNode resourceNode = resourceTree.findResourceNode(path);
return resourceNode == null ? null : resourceNode.getResourceClass();
}
/**
* Gets the resource method corresponding to the HTTP method
* and request path.
*
* @param httpMethod The HTTP method
* @param path The request path
* @param resourceClass The resource class for this method
* @return The resource method
*/
public Method getResourceMethod(String httpMethod, String path, Class> resourceClass) {
ResourceNode resourceNode = resourceTree.findResourceNode(path);
if (resourceNode == null) {
return null;
}
for (Method resourceMethod : resourceNode.getResourceMethods()) {
if (httpMethod.equalsIgnoreCase(ControllerReflUtils.getHttpMethod(resourceMethod))) {
return ClassUtils.getFirstImplementation(resourceClass, resourceMethod);
}
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy