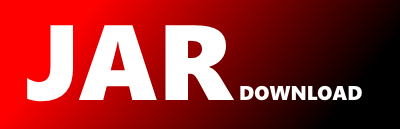
net.craftforge.essential.controller.phases.flow.DefaultPhaseFlow Maven / Gradle / Ivy
The newest version!
/*
* This file is part of essential.
*
* essential is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* essential is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with essential. If not, see .
*/
package net.craftforge.essential.controller.phases.flow;
import net.craftforge.essential.controller.ControllerConfiguration;
import net.craftforge.essential.controller.ControllerException;
import net.craftforge.essential.controller.ControllerPhase;
import net.craftforge.essential.controller.ControllerState;
import net.craftforge.essential.controller.phases.*;
import net.craftforge.essential.controller.resolvers.MethodResolver;
import net.craftforge.essential.controller.utils.ControllerReflUtils;
import net.craftforge.essential.core.constants.HttpMethods;
import javax.inject.Inject;
/**
* The standard phase flow defines a default flow for phases.
*
* @author Christian Bick
* @since 30.05.2011
*/
public class DefaultPhaseFlow implements PhaseFlow {
private MethodResolver methodResolver;
private ControllerConfiguration configuration;
private DocumentationPhase documentationPhase;
private AllocationPhase allocationPhase;
private AuthenticationPhase authenticationPhase;
private InvocationPhase invocationPhase;
private ProductionPhase productionPhase;
@Inject
public DefaultPhaseFlow(MethodResolver methodResolver, ControllerConfiguration configuration,
DocumentationPhase documentationPhase, AllocationPhase allocationPhase,
AuthenticationPhase authenticationPhase, InvocationPhase invocationPhase,
ProductionPhase productionPhase) {
this.methodResolver = methodResolver;
this.configuration = configuration;
this.documentationPhase = documentationPhase;
this.allocationPhase = allocationPhase;
this.authenticationPhase = authenticationPhase;
this.invocationPhase = invocationPhase;
this.productionPhase = productionPhase;
}
/**
* {@inheritDoc}
*/
public ControllerPhase getFirstPhase() {
if (methodResolver.getHttpMethod().equalsIgnoreCase(HttpMethods.OPTIONS)) {
return documentationPhase;
} else {
return allocationPhase;
}
}
/**
* {@inheritDoc}
*/
public ControllerPhase getPhaseAfter(ControllerPhase phase, ControllerState state) throws ControllerException {
if (phase instanceof AllocationPhase) {
return getPhaseAfterAllocation(state);
} else if (phase instanceof AuthenticationPhase) {
return getPhaseAfterAuthentication();
} else if (phase instanceof InvocationPhase) {
return getPhaseAfterInvocation();
} else if (phase instanceof DocumentationPhase) {
return getPhaseAfterDocumentation();
} else if (phase instanceof ProductionPhase) {
return getPhaseAfterProduction();
} else {
throw new ControllerException("Undefined flow for phase: " + phase.getClass());
}
}
/**
* Gets the phase following an allocation phase using the controller state
* for its decision.
*
* @param state The controller state
* @return The phase following an allocation phase
*/
protected ControllerPhase getPhaseAfterAllocation(ControllerState state) {
// The authentication phase is skipped if no authentication handler is given or the resource method is
// annotated as public
if (configuration.getAuthenticationHandler() == null
|| ControllerReflUtils.isPublic(state.getResourceMethod())) {
return getPhaseAfterAuthentication();
}
return authenticationPhase;
}
/**
* Gets the phase following an authentication phase.
*
* @return The phase following an authentication phase
*/
protected ControllerPhase getPhaseAfterAuthentication() {
return invocationPhase;
}
/**
* Gets the phase following an invocation phase.
*
* @return The phase following an invocation phase
*/
protected ControllerPhase getPhaseAfterInvocation() {
return productionPhase;
}
/**
* Gets the phase following a documentation phase.
*
* @return The phase following a documentation phase
*/
protected ControllerPhase getPhaseAfterDocumentation() {
return productionPhase;
}
/**
* Gets the phase following a production phase.
*
* @return The phase following a production phase
*/
protected ControllerPhase getPhaseAfterProduction() {
// The production phase is the last phase of the flow
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy