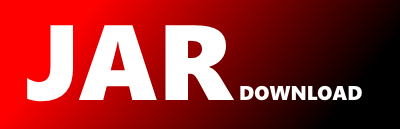
net.craftforge.essential.controller.utils.ControllerRegExUtils Maven / Gradle / Ivy
/*
* This file is part of essential.
*
* essential is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* essential is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with essential. If not, see .
*/
package net.craftforge.essential.controller.utils;
import java.util.regex.Pattern;
/**
* Utilities for regular expression actions.
*
* @author Christian Bick
* @since 17.02.2011
*/
public class ControllerRegExUtils {
/**
* The regular expression used for default resource path variables
*/
public static final String DEFAULT_REG_EX = "[^/]+?";
/**
* Finds regular expression characters
*/
public static final Pattern FIND_REG_EX_OPERATORS = Pattern.compile("([\\\\*+\\[\\]()\\$.?\\^|])");
/**
* Finds semi colons
*/
public static final Pattern FIND_SEMI_COLON = Pattern.compile(";");
/**
* Finds colons
*/
public static final Pattern FIND_COLON = Pattern.compile(":");
/**
* Finds all literals and numbers
*/
public static final Pattern FIND_ALL_LITERALS = Pattern.compile("[a-zA-Z0-9]");
/**
* Finds all none literals and numbers
*/
public static final Pattern FIND_ALL_NONE_LITERALS = Pattern.compile("[^a-zA-Z0-9]");
/**
* Finds all opening and closing curly brackets
*/
public static final Pattern FIND_CURLY_BRACKETS = Pattern.compile("[\\{\\}]");
/**
* Finds all characters enclosed by curly brackets including the brackets
*/
public static final Pattern FIND_ENCLOSED_BY_CURLY_BRACKETS = Pattern.compile("\\{.*\\}");
/**
* Gets the regular expression encoded in a (virtual) path part.
* The following cases are handled by this method:
*
* - If the path part is static (e.g. 'wine') then this method will
* only escape regular expression characters.
* - In case the part is not static (e.g. '{wineId}') then a
* default regular expression matching all characters is returned.
* - For none-static parts containing an own regular expression
* (e.g. '[wineId:([0-9])+'), the regular expression is being extracted.
*
*
* @param pathPart The path part
* @return The regular expression
*/
public static String getRegExFromPathPart(String pathPart) {
String regEx;
if (FIND_ENCLOSED_BY_CURLY_BRACKETS.matcher(pathPart).matches()) {
if (FIND_COLON.matcher(pathPart).find()) {
String[] paramParts = pathPart.split(":");
regEx = FIND_CURLY_BRACKETS.matcher(paramParts[1]).replaceAll("");
} else {
regEx = DEFAULT_REG_EX;
}
} else {
regEx = FIND_REG_EX_OPERATORS.matcher(pathPart).replaceAll("\\\\$");
}
return regEx;
}
/**
* Gets the values from a (real) path part assuming the part to be variable.
* Multiple values are expected to be semi-colon separated.
*
Examples:
*
* - single value: '123' -> { '123' }
* - multi value: '123;456;789', -> { '123', '456', '789' }
*
*
* @param pathPart The path part
* @return The values from the part
*/
public static String[] getValuesFromPathPart(String pathPart) {
return FIND_SEMI_COLON.split(pathPart);
}
/**
* Gets the param name from a (virtual) path part assuming it to not be static.
* Depending on the flag, the parameter name is returned in curly brackets or without.
*
*
* @param pathPart The path part
* @param withCurlyBrackets Whether the result has curly brackets or not
* @return The param name
*/
public static String getParamNameFromPathPart(String pathPart, boolean withCurlyBrackets) {
String paramNamePart;
if (pathPart.contains(":")) {
paramNamePart = pathPart.split(":")[0];
} else {
paramNamePart = pathPart;
}
paramNamePart = FIND_CURLY_BRACKETS.matcher(paramNamePart).replaceAll("");
return withCurlyBrackets ? "{" + paramNamePart + "}" : paramNamePart;
}
/**
* Gets the param name from a (virtual) path part assuming it to not be static.
*
* @param pathPart The path part
* @return The param name (with curly brackets)
*/
public static String getParamNameFromPathPart(String pathPart) {
return getParamNameFromPathPart(pathPart, true);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy