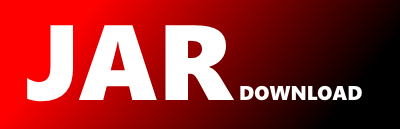
net.customware.confluence.reporting.AbstractReportSetup Maven / Gradle / Ivy
The newest version!
package net.customware.confluence.reporting;
import java.util.List;
import net.customware.confluence.reporting.query.Query;
import org.randombits.confluence.filtering.criteria.Criteria;
import org.randombits.confluence.filtering.criteria.Criterion;
import org.randombits.confluence.filtering.criteria.GroupCriteria;
import org.randombits.confluence.support.MacroInfo;
import org.randombits.storage.Storage;
/**
* A default implementation of {@link ReportSetup} with the basic requirements
* specified. Each plugin will subclass this and add any report-type-specific
* setup options for later use when rendering.
*
* @param
© 2015 - 2025 Weber Informatics LLC | Privacy Policy