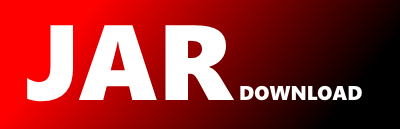
net.customware.confluence.reporting.DefaultReportContext Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2007, CustomWare Asia Pacific
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* * Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* * Neither the name of "CustomWare Asia Pacific" nor the names of its contributors
* may be used to endorse or promote products derived from this software
* without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
package net.customware.confluence.reporting;
import com.atlassian.confluence.renderer.PageContext;
import com.atlassian.renderer.WikiStyleRenderer;
import com.atlassian.renderer.v2.RenderMode;
import com.atlassian.renderer.v2.macro.Macro;
import com.atlassian.spring.container.ContainerManager;
import org.apache.commons.collections.Bag;
import org.apache.commons.collections.bag.HashBag;
import java.util.Map;
/**
* Provides utility functions for handling reports.
*/
public final class DefaultReportContext implements ReportContext {
private static class RootContext {
PageContext pageContext;
Object context;
Report, ?> report;
Map, Bag> macroContent;
RootContext( PageContext ctx ) {
this.pageContext = ctx;
this.macroContent = new java.util.HashMap, Bag>();
}
}
private final ThreadLocal CONTEXT = new ThreadLocal();
private WikiStyleRenderer wikiStyleRenderer;
public DefaultReportContext() {
}
/* (non-Javadoc)
* @see net.customware.confluence.reporting.ReportBuilder#executeRoot(com.atlassian.confluence.renderer.PageContext, net.customware.confluence.reporting.Executor)
*/
/* (non-Javadoc)
* @see net.customware.confluence.reporting.ReportContext#executeRoot(com.atlassian.confluence.renderer.PageContext, net.customware.confluence.reporting.Executor)
*/
public T executeRoot( PageContext pageContext, Executor extends T> executor ) throws ReportException {
// Get the context directly so that no exceptions are thrown.
RootContext ctx = CONTEXT.get();
boolean isRoot = false;
if ( ctx == null ) {
ctx = new RootContext( pageContext );
CONTEXT.set( ctx );
isRoot = true;
}
try {
return executor.execute();
} finally {
if ( isRoot )
CONTEXT.set( null );
}
}
/* (non-Javadoc)
* @see net.customware.confluence.reporting.ReportBuilder#isReporting()
*/
/* (non-Javadoc)
* @see net.customware.confluence.reporting.ReportContext#isReporting()
*/
public boolean isReporting() {
return CONTEXT.get() != null;
}
/**
* Returns the root context.
*
* @return
* @throws ContextException
*/
private RootContext getRootContext() throws ContextException {
RootContext ctx = CONTEXT.get();
if ( ctx == null )
throw new ContextException( "This is not currently in the context of a report." );
return ctx;
}
/* (non-Javadoc)
* @see net.customware.confluence.reporting.ReportBuilder#executeContext(java.lang.Object, net.customware.confluence.reporting.Executor)
*/
/* (non-Javadoc)
* @see net.customware.confluence.reporting.ReportContext#executeContext(java.lang.Object, net.customware.confluence.reporting.Executor)
*/
public T executeContext( Object context, Executor extends T> executor ) throws ReportException {
RootContext ctx = getRootContext();
Object prevContext = ctx.context;
ctx.context = context;
try {
return executor.execute();
} finally {
ctx.context = prevContext;
}
}
/* (non-Javadoc)
* @see net.customware.confluence.reporting.ReportBuilder#executeReport(net.customware.confluence.reporting.Report, net.customware.confluence.reporting.Executor)
*/
/* (non-Javadoc)
* @see net.customware.confluence.reporting.ReportContext#executeReport(net.customware.confluence.reporting.Report, net.customware.confluence.reporting.Executor)
*/
public T executeReport( Report, ?> report, Executor extends T> executor ) throws ReportException {
RootContext ctx = getRootContext();
Report, ?> prevReport = ctx.report;
ctx.report = report;
try {
return executor.execute();
} finally {
ctx.report = prevReport;
}
}
/* (non-Javadoc)
* @see net.customware.confluence.reporting.ReportBuilder#getCurrentReport()
*/
/* (non-Javadoc)
* @see net.customware.confluence.reporting.ReportContext#getCurrentReport()
*/
public Report, ?> getCurrentReport() throws ContextException {
return getRootContext().report;
}
/**
* Returns the current item being reported on.
*
* @return the current report item.
* @throws ReportException if not currently in the context of a report.
*/
public Object getCurrentReportItem() throws ReportException {
final Report, ?> report = getCurrentReport();
if ( report != null )
return report.getCurrentItem();
return null;
}
/* (non-Javadoc)
* @see net.customware.confluence.reporting.ReportBuilder#getCurrentContext()
*/
/* (non-Javadoc)
* @see net.customware.confluence.reporting.ReportContext#getCurrentContext()
*/
public Object getCurrentContext() throws ContextException {
return getRootContext().context;
}
/* (non-Javadoc)
* @see net.customware.confluence.reporting.ReportBuilder#getCurrentContext(java.lang.Class)
*/
/* (non-Javadoc)
* @see net.customware.confluence.reporting.ReportContext#getCurrentContext(java.lang.Class)
*/
public C getCurrentContext( Class targetClass ) throws ContextException {
Object context = getCurrentContext();
if ( targetClass.isInstance( context ) )
return ( C ) context;
return null;
}
/* (non-Javadoc)
* @see net.customware.confluence.reporting.ReportBuilder#getRootPageContext()
*/
/* (non-Javadoc)
* @see net.customware.confluence.reporting.ReportContext#getRootPageContext()
*/
public PageContext getRootPageContext() throws ContextException {
return getRootContext().pageContext;
}
/* (non-Javadoc)
* @see net.customware.confluence.reporting.ReportBuilder#getCurrentPageContext()
*/
/* (non-Javadoc)
* @see net.customware.confluence.reporting.ReportContext#getCurrentPageContext()
*/
public PageContext getCurrentPageContext() throws ReportException {
Report, ?> report = getCurrentReport();
if ( report != null )
return report.getPageContext();
else
return getRootPageContext();
}
/* (non-Javadoc)
* @see net.customware.confluence.reporting.ReportContext#renderWiki(java.lang.String, com.atlassian.renderer.v2.RenderMode, net.customware.confluence.reporting.macro.AbstractReportingMacro)
*/
public String renderWiki( String wiki, RenderMode renderMode, Macro macro )
throws ReportException {
if ( wiki != null ) {
PageContext ctx = getCurrentPageContext();
Object item = getCurrentReportItem();
if ( item == null )
item = ctx.getEntity();
// Ensure no more than 10 instances at a time to prevent infinite recursion.
if ( item != null && checkItem( macro, item, 10 ) )
throw new ReportException( "Item already rendered 10 times. Canceled to prevent infinite recursion: " + item );
String rendered;
if ( item != null )
addItem( macro, item );
try {
if ( renderMode != null )
ctx.pushRenderMode( renderMode );
try {
rendered = getWikiStyleRenderer().convertWikiToXHtml( ctx, wiki );
} finally {
if ( renderMode != null )
ctx.popRenderMode();
}
} finally {
if ( item != null )
removeItem( macro, item );
}
return rendered;
}
return null;
}
private void removeItem( Macro macro, Object item ) throws ContextException {
Bag contents = getMacroContents( macro, false );
if ( contents != null )
contents.remove( item );
}
private void addItem( Macro macro, Object item ) throws ContextException {
Bag contents = getMacroContents( macro, true );
contents.add( item );
}
private boolean checkItem( Macro macro, Object item, int maxOccurrences ) throws ContextException {
Bag contents = getMacroContents( macro, false );
if ( contents != null )
return contents.getCount( item ) >= maxOccurrences;
return false;
}
private Bag getMacroContents( Macro macro, boolean create )
throws ContextException {
RootContext root = getRootContext();
Bag contents = root.macroContent.get( macro.getClass() );
if ( contents == null && create ) {
contents = new HashBag();
root.macroContent.put( macro.getClass(), contents );
}
return contents;
}
private WikiStyleRenderer getWikiStyleRenderer() {
if ( wikiStyleRenderer == null ) {
wikiStyleRenderer = ( WikiStyleRenderer ) ContainerManager.getComponent( "wikiStyleRenderer" );
}
return wikiStyleRenderer;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy