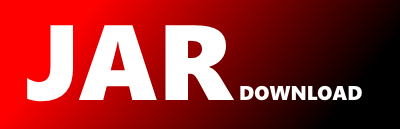
net.customware.confluence.reporting.ReportSetup Maven / Gradle / Ivy
The newest version!
package net.customware.confluence.reporting;
import java.util.List;
import org.randombits.facade.Facadable;
import net.customware.confluence.reporting.query.Query;
import net.customware.confluence.reporting.query.Queryable;
@Facadable
public interface ReportSetup extends Queryable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy