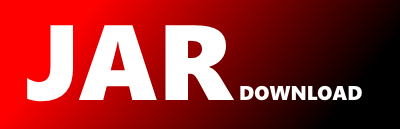
net.customware.confluence.reporting.DefaultReport Maven / Gradle / Ivy
package net.customware.confluence.reporting;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import net.customware.confluence.reporting.query.Query;
import org.randombits.confluence.filtering.criteria.Criteria;
import org.randombits.confluence.support.MacroInfo;
import com.atlassian.confluence.core.ContentEntityObject;
import com.atlassian.confluence.pages.Attachment;
import com.atlassian.confluence.renderer.PageContext;
import com.atlassian.confluence.spaces.Space;
/**
* The default implementation for a report during its execution. Most reports
* will be able to use this class without modification, but those wishing to
* enhance or replace it in a report can do so easily by overriding the
* {@link AbstractReportMacro#createReport( MacroInfo )} method in their report
* type.
*/
public class DefaultReport, O extends ReportOutput> implements Report {
private Report, ?> parent;
private Object currentItem;
private ContentEntityObject content;
private PageContext pageContext;
private Exception queryException;
private long currentItemNumber;
private S setup;
private Map attributes;
/**
* Constructs a new report based on the provided {@link ReportSetup}
*
* @param setup
* the report setup.
*/
public DefaultReport( S setup ) {
this.setup = setup;
}
/**
* @see Report#setParent(Report)
*/
public void setParent( Report, ?> parent ) {
this.parent = parent;
}
/**
* @see Report#getSetup()
*/
public S getSetup() {
return setup;
}
/**
* Returns the criteria for this report. This is useful when sorting
* children of reports if the depth is increased.
*/
public Criteria getCriteria() {
return setup.getCriteria();
}
/**
* Returns the list of outputs added to this report.
*/
public List getOutputs() {
return setup.getOutputs();
}
/**
* Returns the current item being reported on.
*/
public Object getCurrentItem() {
return currentItem;
}
/**
* Sets the current item to a new value, incrementing the current item
* number. It will also check if the item is a {@link ContentEntityObject},
* and if so will make it the current content value.
*
* @param newItem
* the new item.
* @see #getContent()
*/
public void setCurrentItem( Object newItem ) {
this.currentItem = newItem;
currentItemNumber++;
if ( currentItem == null )
content = null;
else if ( currentItem instanceof ContentEntityObject )
content = ( ContentEntityObject ) currentItem;
else if ( currentItem instanceof Space )
content = ( ( Space ) currentItem ).getDescription();
else if ( currentItem instanceof Attachment )
content = ( ( Attachment ) currentItem ).getContent();
// Reset the page context regardless.
pageContext = null;
}
/**
* @see net.customware.confluence.plugin.reporting.Report#getCurrentItemNumber()
*/
public long getCurrentItemNumber() {
return currentItemNumber;
}
/**
* @see net.customware.confluence.plugin.reporting.Report#getReporterException()
*/
public Exception getQueryException() {
return queryException;
}
/**
* @see net.customware.confluence.plugin.reporting.Report#setReporterException(java.lang.Exception)
*/
public void setQueryException( Exception reporterException ) {
this.queryException = reporterException;
}
/**
* @throws ReportException
* @see net.customware.confluence.plugin.reporting.Report#getContent()
*/
public ContentEntityObject getContent() throws ReportException {
if ( content != null )
return content;
if ( parent != null )
return parent.getContent();
return ReportBuilder.getRootContent();
}
/**
* @throws ReportException
* @see net.customware.confluence.plugin.reporting.Report#getPageContext()
*/
public PageContext getPageContext() throws ReportException {
if ( pageContext == null ) {
pageContext = parent != null ? parent.getPageContext() : null;
if ( pageContext == null )
pageContext = ReportBuilder.getRootPageContext();
ContentEntityObject contextEntity = pageContext == null ? null : pageContext.getEntity();
if ( pageContext == null || ( content != null && !content.equals( contextEntity ) ) ) {
pageContext = new PageContext( content, pageContext );
}
}
return pageContext;
}
/**
* @see net.customware.confluence.plugin.reporting.Report#getParent()
*/
public Report, ?> getParent() {
return parent;
}
/**
* @see Report#getQuery()
*/
public Query extends Object> getQuery() {
return setup.getQuery();
}
public Object getAttribute( String name ) {
if ( attributes != null ) {
return attributes.get( name );
}
return null;
}
public Collection getAttributeNames() {
if ( attributes != null )
return attributes.keySet();
return Collections.EMPTY_SET;
}
public void setAttribute( String name, Object value ) {
if ( attributes == null )
attributes = new java.util.HashMap();
attributes.put( name, value );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy