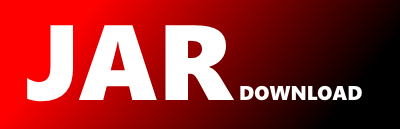
net.customware.confluence.reporting.Report Maven / Gradle / Ivy
package net.customware.confluence.reporting;
import java.util.Collection;
import java.util.List;
import org.randombits.facade.Facadable;
import net.customware.confluence.reporting.query.Query;
import com.atlassian.confluence.core.ContentEntityObject;
import com.atlassian.confluence.renderer.PageContext;
/**
* This is the basic class that defines what a report looks like.
*
* @param
* The type of value returned by the report.
* @param
* The report setup type.
* @param
* The report output type.
*/
@Facadable
public interface Report, O extends ReportOutput> {
/**
* Returns the list of outputs for this report.
*
* @return
*/
@Facadable
List getOutputs();
/**
* Returns the currently reported item.
*
* @return
*/
Object getCurrentItem();
/**
* Sets the current item to the specified new item object. If the item is a
* {@link ContentEntityObject} the item will become the current content
* object also, accessible via {@link #getContent()}.
*
* @param newItem
* the new report item.
*/
void setCurrentItem( Object newItem );
/**
* Returns the sequence number of the current item. This can be used to
* determine what 'row' the report is up to, or if it is an even or odd row,
* etc. This is incremented automatically when setting a new 'current item'.
*
* @return the current item number.
* @see #setCurrentItem(Object)
*/
long getCurrentItemNumber();
/**
* Returns the ContentEntityObject to render the current report item as.
*
* @return the current content.
* @throws ReportException
* if not currently in the context of a report.
*/
ContentEntityObject getContent() throws ReportException;
/**
* Returns the current page context. This will not necessarily be the actual
* page the report is on - it may be the context for the item being reported
* on if it is a page or news item.
*
* @return the current page context.
* @throws ReportException
* if not currently in the context of a report.
*/
PageContext getPageContext() throws ReportException;
/**
* Sets the parent report to the specified value.
*
* @param parent
* the parent report.
*/
void setParent( Report, ?> parent );
/**
* Returns the current parent report.
*
* @return the parent report.
*/
Report, ?> getParent();
/**
* Returns the {@link Query} for this report.
*
* @return the query.
*/
Query extends Object> getQuery();
/**
* Returns the setup details for this report.
*
* @return the report setup.
*/
S getSetup();
/**
* Returns the set of names of stored attributes in the current report.
*
* @return The attribute name set.
*/
public Collection getAttributeNames();
/**
* Sets the specified attribute name to the value.
*
* @param name
* The attribute name.
* @param value
* The value.
*/
public void setAttribute( String name, Object value );
/**
* Returns the value for the specified attribute name.
*
* @param name
* The attribute name.
* @return The attribute value.
*/
public Object getAttribute( String name );
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy