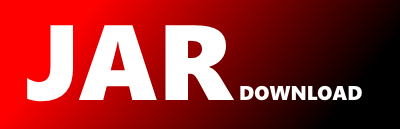
net.customware.confluence.reporting.ReportUtils Maven / Gradle / Ivy
package net.customware.confluence.reporting;
import net.customware.confluence.reporting.query.Query;
import net.customware.confluence.reporting.query.QueryException;
import org.apache.commons.collections.IteratorUtils;
import org.randombits.confluence.support.ServletAssistant;
import javax.servlet.http.HttpServletRequest;
import java.util.*;
import static com.atlassian.confluence.util.GeneralUtil.escapeXml;
/**
* Provides utility methods for working with collection, iterators, etc.
*
* @author David Peterson
*/
public final class ReportUtils {
private static final String ATTRIBUTES_NAME = "net.customware.confluence.reporting:attributes";
private ReportUtils() {
}
/**
* Returns true
if the value is either an {@link Iterator},
* {@link Iterable}, {@link Enumeration} or an array.
*
* @param value The value to check.
* @return true
if the value is iterable.
*/
public static boolean isIterable( Object value ) {
return value instanceof Iterator || value instanceof Iterable || value instanceof Enumeration
|| value instanceof Query || value != null && value.getClass().isArray();
}
/**
* Returns the value as an {@link Iterator}. If the value is itself an
* iterator, an instance of {@link Iterable}, or is an array, the items in
* the value will be returned as the list. Otherwise, if the value is not
* null
, it will be returned as the only item in the
* iterator. If the value is null
, an empty iterator is
* returned.
*
* @param value
* The value to convert.
* @return The iterator.
*/
public static Iterator> asIterator( Object value ) {
if ( value instanceof Iterator ) {
return ( Iterator> ) value;
} else if ( value instanceof Iterable> ) {
return ( ( Iterable> ) value ).iterator();
} else if ( value instanceof Enumeration ) {
return IteratorUtils.asIterator( ( Enumeration> ) value );
} else if ( value instanceof Query> ) {
try {
return ( ( Query> ) value ).execute();
} catch ( QueryException e ) {
throw new RuntimeException( e.getMessage(), e );
}
} else if ( value != null ) {
if ( value.getClass().isArray() ) {
return IteratorUtils.arrayIterator( ( Object[] ) value );
} else {
return Arrays.asList( value ).iterator();
}
} else {
return IteratorUtils.emptyIterator();
}
}
/**
* Returns the value as a {@link Collection}, which may or may not be
* mutable. It's safest to assume you will not be able to modify the
* results. The result will never be null
.
*
* @param value
* The value to convert to a collection.
* @return The collection.
*/
public static Collection> asCollection( Object value ) {
if ( value instanceof Collection ) {
return ( Collection> ) value;
} else if ( value instanceof Iterable ) {
return IteratorUtils.toList( ( ( Iterable> ) value ).iterator() );
} else if ( value instanceof Iterator ) {
return IteratorUtils.toList( ( Iterator> ) value );
} else if ( value instanceof Enumeration ) {
return Collections.list( ( Enumeration> ) value );
} else if ( value instanceof Query ) {
try {
return IteratorUtils.toList( (( Query> ) value ).execute() );
} catch (QueryException e) {
return Collections.EMPTY_LIST;
}
} else if ( value != null ) {
if ( value.getClass().isArray() )
return Arrays.asList( ( Object[] ) value );
else
return Arrays.asList( value );
} else {
return Collections.EMPTY_LIST;
}
}
public static void appendAttribute( StringBuffer out, String name, Object value ) {
if ( value != null )
out.append( " " ).append( escapeXml( name ) ).append( "='" ).append( escapeXml( value.toString() ) )
.append( "'" );
}
public static void appendStyleParam( StringBuffer out, String name, String value ) {
if ( value != null )
out.append( escapeXml( name ) ).append( ": " ).append( escapeXml( value ) ).append( "; " );
}
public static void appendAttribute( StringBuilder out, String name, Object value ) {
if ( value != null )
out.append( " " ).append( escapeXml( name ) ).append( "='" ).append( escapeXml( value.toString() ) )
.append( "'" );
}
public static void appendStyleParam( StringBuilder out, String name, String value ) {
if ( value != null )
out.append( escapeXml( name ) ).append( ": " ).append( escapeXml( value ) ).append( "; " );
}
public static void setAttribute( Object context, String name, Object value ) throws ReportException {
Report, ?> report = getReport( context );
if ( report != null ) {
report.setAttribute( name, value );
} else {
Map attributes = getRequestAttributes( true );
if ( attributes != null )
attributes.put( name, value );
}
}
@SuppressWarnings({"unchecked"})
private static Map getRequestAttributes( boolean create ) {
Map attributes = null;
HttpServletRequest req = ServletAssistant.getInstance().getRequest();
if ( req != null ) {
Object value = req.getAttribute( ATTRIBUTES_NAME );
if ( value instanceof Map )
attributes = ( Map ) value;
if ( attributes == null && create ) {
attributes = new java.util.HashMap();
req.setAttribute( ATTRIBUTES_NAME, attributes );
}
}
return attributes;
}
private static Report, ?> getReport( Object context ) throws ReportException {
Report, ?> report = null;
if ( context instanceof Report ) {
report = ( Report, ?> ) context;
} else if ( ReportBuilder.isReporting() && ReportBuilder.getRootContent() != context ) {
// Only get the 'current report' if the context is not the root
// content
report = ReportBuilder.getCurrentReport();
}
return report;
}
public static Object getAttribute( Object context, String name ) throws ReportException {
Report, ?> report = getReport( context );
if ( report != null ) {
return report.getAttribute( name );
} else {
Map attributes = getRequestAttributes( false );
if ( attributes != null ) {
return attributes.get( name );
} else {
return null;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy