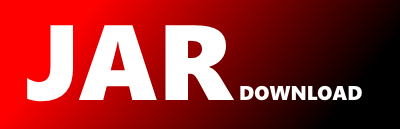
net.customware.confluence.reporting.supplier.AbstractEntitySupplier Maven / Gradle / Ivy
/*
* Copyright (c) 2007, CustomWare Asia Pacific
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* * Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* * Neither the name of "CustomWare Asia Pacific" nor the names of its contributors
* may be used to endorse or promote products derived from this software
* without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
package net.customware.confluence.reporting.supplier;
import net.customware.confluence.reporting.ReportBuilder;
import net.customware.confluence.reporting.ReportException;
import org.randombits.confluence.supplier.LinkableSupplier;
import org.randombits.confluence.supplier.SupplierException;
import org.randombits.confluence.supplier.UnsupportedContextException;
import org.randombits.confluence.support.LinkAssistant;
import org.randombits.storage.ResourceStorage;
import com.atlassian.confluence.core.ConfluenceEntityObject;
import com.atlassian.confluence.core.Versioned;
import com.atlassian.confluence.links.LinkManager;
import com.atlassian.confluence.pages.Page;
import com.atlassian.confluence.renderer.PageContext;
import com.atlassian.renderer.links.LinkResolver;
import com.atlassian.spring.container.ContainerManager;
import com.atlassian.user.User;
/**
* Supplies information about Confluence entities.
*
* @author David Peterson
*/
public abstract class AbstractEntitySupplier extends AbstractConfluenceSupplier implements LinkableSupplier {
private static final String ID_KEY = "id";
private static final String TYPE_KEY = "type";
private static final String TITLE_KEY = "title";
private static final String URL_KEY = "url";
private static final String ICON_KEY = "icon";
private static final String CREATOR_KEY = "creator";
private static final String CREATION_DATE_KEY = "creation date";
private static final String MODIFIER_KEY = "modifier";
private static final String MODIFICATION_DATE_KEY = "modification date";
private static final String VERSION_KEY = "version";
private static final String IS_LATEST_VERSION_KEY = "is latest version";
private static final String LATEST_VERSION_KEY = "latest version";
private static final String EQUALS_PREFIX = "equals ";
private static final String SELF = "@self";
private static final String PARENT = "@parent";
private ResourceStorage resources;
private LinkManager linkManager;
private LinkResolver linkResolver;
private Class type;
public AbstractEntitySupplier( Class type ) {
this.type = type;
}
protected Class getType() {
return type;
}
/**
* Finds the specified value given the context.
*
* @param context
* The context object.
* @param key
* The key of the value to find.
* @return The value, or the defaultValue.
* @throws org.randombits.confluence.supplier.UnsupportedContextException
* if the context is not supported.
*/
public Object getValue( Object context, String key ) throws UnsupportedContextException, SupplierException {
if ( type.isInstance( context ) ) {
T entity = type.cast( context );
return getValue( entity, key );
}
throw new UnsupportedContextException( context );
}
/**
* Returns any entity-type specific key values.
*
* @param entity
* The entity.
* @param key
* The key.
* @return The value, or null
.
* @throws SupplierException
*/
protected Object getValue( T entity, String key ) throws SupplierException {
if ( key == null || TITLE_KEY.equals( key ) )
return getTitle( entity );
else if ( ID_KEY.equals( key ) )
return new Long( entity.getId() );
else if ( TYPE_KEY.equals( key ) )
return getType( entity );
else if ( URL_KEY.equals( key ) )
return getUrl( entity );
else if ( key.startsWith( EQUALS_PREFIX ) )
return equalsKey( entity, key.substring( EQUALS_PREFIX.length() ) );
if ( CREATOR_KEY.equals( key ) )
return getUser( entity.getCreatorName() );
else if ( CREATION_DATE_KEY.equals( key ) )
return entity.getCreationDate();
else if ( MODIFIER_KEY.equals( key ) )
return getUser( entity.getLastModifierName() );
else if ( MODIFICATION_DATE_KEY.equals( key ) )
return entity.getLastModificationDate();
if ( ICON_KEY.equals( key ) )
return getIconURL( entity );
if ( entity instanceof Versioned ) {
Versioned versioned = ( Versioned ) entity;
if ( VERSION_KEY.equals( key ) )
return new Integer( versioned.getVersion() );
if ( IS_LATEST_VERSION_KEY.equals( key ) )
return Boolean.valueOf( versioned.isLatestVersion() );
if ( LATEST_VERSION_KEY.equals( key ) )
return versioned.getLatestVersion();
}
return null;
}
protected abstract String getIconURL( T entity );
/**
* Returns the URL to access the specified entity.
*
* @param entity
* The entity.
* @return The web-context-relative URL.
*/
protected abstract String getUrl( T entity );
/**
* Returns the human-readable title for the entity.
*
* @param entity
* The entity.
* @return The title.
*/
protected abstract String getTitle( T entity );
/**
* Returns the entity type string.
*
* @param entity
* The entity.
* @return The type string.
*/
protected abstract String getType( T entity );
public boolean supportsContext( Object context ) {
return type.isInstance( context );
}
/**
* Finds the URL which the specified value can link to for more information.
* The URL may be relative to the Confluence install, or absolute. If none
* is available, return null
.
*
* @param context
* The context object.
* @return The URL.
* @throws org.randombits.confluence.supplier.UnsupportedContextException
* if the context object is not supported.
* @throws org.randombits.confluence.supplier.SupplierException
* if there is a problem finding the value.
*/
public String getLink( Object context ) throws UnsupportedContextException, SupplierException {
if ( type.isInstance( context ) ) {
T entity = type.cast( context );
return getUrl( entity );
}
throw new UnsupportedContextException( context );
}
private static Boolean equalsKey( ConfluenceEntityObject entity, String key ) {
try {
PageContext ctx = ReportBuilder.getCurrentPageContext();
key = key.trim();
if ( ctx != null ) {
ConfluenceEntityObject target = ctx.getEntity();
if ( SELF.equals( key ) )
return new Boolean( entity.equals( target ) );
else if ( PARENT.equals( key ) && target instanceof Page )
return new Boolean( entity.equals( ( ( Page ) target ).getParent() ) );
target = LinkAssistant.getInstance().getLinkedEntity( ctx, key );
return new Boolean( entity.equals( target ) );
}
return Boolean.FALSE;
} catch ( ReportException e ) {
return Boolean.FALSE;
}
}
private Object getUser( String username ) {
if ( username != null ) {
User user = getUserAccessor().getUser( username );
if ( user != null )
return user;
}
return username;
}
/**
* Returns I18N text for the specified key.
*
* @param key
* @return
*/
protected String getText( String key, String def ) {
if ( resources == null ) {
resources = new ResourceStorage( getClass() );
}
return resources.getString( key, def );
}
protected LinkManager getLinkManager() {
if ( linkManager == null )
linkManager = ( LinkManager ) ContainerManager.getComponent( "linkManager" );
return linkManager;
}
protected LinkResolver getLinkResolver() {
if ( linkResolver == null )
linkResolver = ( LinkResolver ) ContainerManager.getComponent( "linkResolver" );
return linkResolver;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy