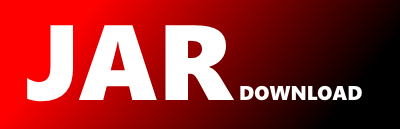
net.dankito.utils.javafx.ui.extensions.TableViewExtensions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-fx-utils Show documentation
Show all versions of java-fx-utils Show documentation
Some basic JavaFX utils needed in many projects
package net.dankito.utils.javafx.ui.extensions
import javafx.scene.control.TableColumn
import javafx.scene.control.TableView
import tornadofx.cellFormat
import tornadofx.column
import java.text.DateFormat
import java.text.NumberFormat
import kotlin.reflect.KMutableProperty1
class TableViewExtensions {
companion object {
const val DateColumnPrefWidth = 80.0
val DateTimeFormat = DateFormat.getDateInstance(DateFormat.SHORT)
const val CurrencyColumnPrefWidth = 110.0
val CurrencyNumberFormat = NumberFormat.getCurrencyInstance()
}
}
/**
* Create a column with default values set suitable for columns displaying a date:
* Cell alignment gets set to center and cell text gets formatted with DateFormat.getDateInstance(DateFormat.SHORT).
*/
inline fun TableView.dateColumn(title: String,
prop: KMutableProperty1,
dateFormat: DateFormat = TableViewExtensions.DateTimeFormat,
noinline op: TableColumn.() -> Unit = {}): TableColumn {
return this.column(title, prop, op).apply {
prefWidth = TableViewExtensions.DateColumnPrefWidth
addStyleToCurrentStyle("-fx-alignment: CENTER;")
cellFormat { text = dateFormat.format(it) }
}
}
/**
* Create a column with default values set suitable for columns displaying a concurrency:
* Cell alignment gets set to center right and cell text gets formatted with NumberFormat.getCurrencyInstance().
*/
inline fun TableView.currencyColumn(title: String,
prop: KMutableProperty1,
concurrencyNumberFormat: NumberFormat = TableViewExtensions.CurrencyNumberFormat,
noinline op: TableColumn.() -> Unit = {}): TableColumn {
return this.column(title, prop, op).apply {
prefWidth = TableViewExtensions.CurrencyColumnPrefWidth
addStyleToCurrentStyle("-fx-alignment: CENTER-RIGHT;")
cellFormat { text = concurrencyNumberFormat.format(it) }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy