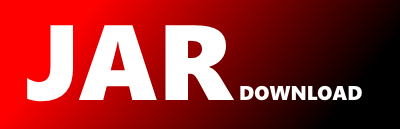
com.mojang.brigadier.builder.ArgumentBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of khl Show documentation
Show all versions of khl Show documentation
Java sdk for Kaiheila bot development
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT license.
package com.mojang.brigadier.builder;
import com.mojang.brigadier.Command;
import com.mojang.brigadier.RedirectModifier;
import com.mojang.brigadier.SingleRedirectModifier;
import com.mojang.brigadier.tree.CommandNode;
import com.mojang.brigadier.tree.RootCommandNode;
import java.util.Collection;
import java.util.Collections;
import java.util.function.Predicate;
public abstract class ArgumentBuilder> {
private final RootCommandNode arguments = new RootCommandNode<>();
private Command command;
private Predicate requirement = s -> true;
private CommandNode target;
private RedirectModifier modifier = null;
private boolean forks;
protected abstract T getThis();
public T then(final ArgumentBuilder argument) {
if (target != null) {
throw new IllegalStateException("Cannot add children to a redirected node");
}
arguments.addChild(argument.build());
return getThis();
}
public T then(final CommandNode argument) {
if (target != null) {
throw new IllegalStateException("Cannot add children to a redirected node");
}
arguments.addChild(argument);
return getThis();
}
public Collection> getArguments() {
return arguments.getChildren();
}
public T executes(final Command command) {
this.command = command;
return getThis();
}
public Command getCommand() {
return command;
}
public T requires(final Predicate requirement) {
this.requirement = requirement;
return getThis();
}
public Predicate getRequirement() {
return requirement;
}
public T redirect(final CommandNode target) {
return forward(target, null, false);
}
public T redirect(final CommandNode target, final SingleRedirectModifier modifier) {
return forward(target, modifier == null ? null : o -> Collections.singleton(modifier.apply(o)), false);
}
public T fork(final CommandNode target, final RedirectModifier modifier) {
return forward(target, modifier, true);
}
public T forward(final CommandNode target, final RedirectModifier modifier, final boolean fork) {
if (!arguments.getChildren().isEmpty()) {
throw new IllegalStateException("Cannot forward a node with children");
}
this.target = target;
this.modifier = modifier;
this.forks = fork;
return getThis();
}
public CommandNode getRedirect() {
return target;
}
public RedirectModifier getRedirectModifier() {
return modifier;
}
public boolean isFork() {
return forks;
}
public abstract CommandNode build();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy