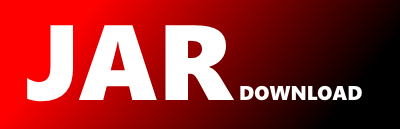
net.derquinse.common.meta.ImmutableFieldMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of derquinse-common-base Show documentation
Show all versions of derquinse-common-base Show documentation
Module containing support classes depending on Java SE 6, Guava 11 and Joda-Time 2.0
/*
* Copyright (C) the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.derquinse.common.meta;
import java.util.LinkedHashMap;
import java.util.Map;
import com.google.common.collect.ForwardingMap;
import com.google.common.collect.ImmutableBiMap;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Maps;
/**
* A metaclass field map implementation.
* @author Andres Rodriguez
* @param Enclosing type.
*/
final class ImmutableFieldMap extends ForwardingMap> implements
FieldMap {
/** Empty map. */
private static final ImmutableFieldMap> EMPTY = new ImmutableFieldMap(
ImmutableMap.> of());
/** Backing bimap. */
private final ImmutableBiMap> map;
/** Returns the empty map. */
@SuppressWarnings("unchecked")
static ImmutableFieldMap of() {
return (ImmutableFieldMap) EMPTY;
}
/** Returns a new builder. */
static Builder builder() {
return new Builder();
}
/** Constructor. */
private ImmutableFieldMap(Map> map) {
this.map = ImmutableBiMap.copyOf(map);
}
@Override
protected Map> delegate() {
return map;
}
/** Builder. */
static final class Builder implements net.derquinse.common.base.Builder> {
/** Builder map. */
private final LinkedHashMap> map = Maps.newLinkedHashMap();
Builder() {
}
Builder add(MetaField super T, ?> field) {
map.put(field.getName(), field);
return this;
}
Builder addAll(Iterable extends MetaField super T, ?>> fields) {
for (MetaField super T, ?> field : fields) {
add(field);
}
return this;
}
Builder addAll(FieldMap super T> fields) {
for (MetaField super T, ?> field : fields.values()) {
add(field);
}
return this;
}
/** Returns whether the builder is urrently empty. */
boolean isEmpty() {
return map.isEmpty();
}
@Override
public ImmutableFieldMap build() {
if (map.isEmpty()) {
return of();
}
return new ImmutableFieldMap(map);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy