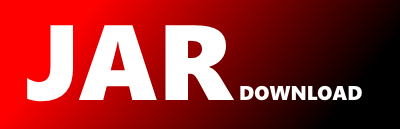
net.derquinse.common.meta.MetaClass Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of derquinse-common-base Show documentation
Show all versions of derquinse-common-base Show documentation
Module containing support classes depending on Java SE 6, Guava 11 and Joda-Time 2.0
/*
* Copyright (C) the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.derquinse.common.meta;
import com.google.common.collect.ImmutableList;
import com.google.common.reflect.TypeToken;
/**
* Meta class descriptors.
* @author Andres Rodriguez
* @param Described type.
*/
public final class MetaClass {
/** Type map. */
private static final MetaClassMap map = new MetaClassMap();
/** Base meta class. */
static final MetaClass ROOT = new MetaClass(WithMetaClass.class);
/**
* Returns the metaclass for a type, discovering it if needed.
* @param type Type.
* @return The meta class.
*/
public static MetaClass of(Class type) {
return of(TypeToken.of(type));
}
/**
* Returns the metaclass for a type.
* @param type Type.
* @return The meta class.
*/
private static MetaClass of(TypeToken type) {
MetaClass metaClass = map.get(type);
if (metaClass == null) {
metaClass = new MetaClassBuilder(type).build();
}
return metaClass;
}
/**
* Registers a metaclass for a type. If the type was already registered a warning is logged and an
* exception is thrown.
* @throws DuplicateMetaClassException if there was a previous entry.
*/
private static void register(MetaClass metaClass) {
map.put(metaClass);
}
/**
* Returns a builder for a new class.
* @throws IllegalStateException the type is already registered.
*/
public static MetaClassBuilder builder(Class type) {
return new MetaClassBuilder(TypeToken.of(type));
}
/** Described type. */
private final TypeToken type;
/** Super class. */
private final MetaClass super T> superclass;
/** Super interfaces. */
private final ImmutableList> superinterfaces;
/** Declared field map. */
private final FieldMap declaredFields;
/** All field map. */
private final FieldMap fields;
/** Root constructor. */
private MetaClass(Class rootClass) {
this.type = TypeToken.of(rootClass);
this.superclass = this;
this.superinterfaces = ImmutableList.of();
this.declaredFields = ImmutableFieldMap.of();
this.fields = ImmutableFieldMap.of();
register(this);
}
/**
* Default constructor.
*/
MetaClass(MetaClassBuilder builder) {
this.type = builder.getType();
this.superclass = builder.getSuperclass();
this.superinterfaces = builder.getSuperinterfaces();
this.declaredFields = builder.getDeclaredFields();
this.fields = builder.getFields();
register(this);
}
/** Returns whether is the root metaclass. */
public boolean isRoot() {
return this == ROOT;
}
/** Returns the enclosing type. */
public final TypeToken getType() {
return type;
}
/** Returns the supermetaclass. */
public MetaClass super T> getSuperclass() {
return superclass;
}
/** Returns the superinterfaces. */
public ImmutableList> getSuperinterfaces() {
return superinterfaces;
}
/** Returns the declared fields. */
public final FieldMap getDeclaredFields() {
return declaredFields;
}
/** Returns the all fields map. */
public FieldMap getFields() {
return fields;
}
@Override
public String toString() {
return Metas.toStringHelper(this).toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy